QByteArray¶
The
QByteArray
class provides an array of bytes. More…
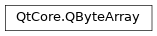
Synopsis¶
Functions¶
def
__add__
(, a2)def
__add__
(, a2)def
__add__
(arg__1)def
__add__
(arg__1)def
__add__
(arg__1)def
__eq__
(, a2)def
__eq__
(, rhs)def
__eq__
(arg__1)def
__eq__
(lhs)def
__ge__
(, a2)def
__ge__
(, rhs)def
__ge__
(arg__1)def
__ge__
(lhs)def
__getitem__
()def
__gt__
(, a2)def
__gt__
(, rhs)def
__gt__
(arg__1)def
__gt__
(lhs)def
__iadd__
(a)def
__iadd__
(arg__1)def
__iadd__
(c)def
__le__
(, a2)def
__le__
(, rhs)def
__le__
(arg__1)def
__le__
(lhs)def
__len__
()def
__lt__
(, a2)def
__lt__
(, a2)def
__lt__
(, rhs)def
__lt__
(arg__1)def
__lt__
(lhs)def
__mgetitem__
()def
__msetitem__
()def
__ne__
(, a2)def
__ne__
(, rhs)def
__ne__
(arg__1)def
__ne__
(lhs)def
__reduce__
()def
__repr__
()def
__setitem__
()def
__str__
()def
append
(a)def
append
(c)def
append
(count, c)def
at
(i)def
back
()def
capacity
()def
cbegin
()def
cend
()def
chop
(n)def
chopped
(len)def
clear
()def
compare
(a[, cs=Qt.CaseSensitive])def
compare
(c[, cs=Qt.CaseSensitive])def
contains
(a)def
contains
(c)def
count
()def
count
(a)def
count
(c)def
data
()def
endsWith
(a)def
endsWith
(c)def
fill
(c[, size=-1])def
front
()def
indexOf
(a[, from=0])def
insert
(i, a)def
insert
(i, count, c)def
isEmpty
()def
isLower
()def
isNull
()def
isSharedWith
(other)def
isUpper
()def
lastIndexOf
(a[, from=-1])def
left
(len)def
leftJustified
(width[, fill=’ ‘[, truncate=false]])def
length
()def
mid
(index[, len=-1])def
operator=
(str)def
prepend
(a)def
prepend
(c)def
prepend
(count, c)def
remove
(index, len)def
repeated
(times)def
replace
(before, after)def
replace
(before, after)def
replace
(before, after)def
replace
(before, after)def
replace
(index, len, s)def
reserve
(size)def
resize
(size)def
right
(len)def
rightJustified
(width[, fill=’ ‘[, truncate=false]])def
setNum
(arg__1[, base=10])def
setNum
(arg__1[, base=10])def
setNum
(arg__1[, f=’g’[, prec=6]])def
setRawData
(a, n)def
shrink_to_fit
()def
simplified
()def
size
()def
split
(sep)def
squeeze
()def
startsWith
(a)def
startsWith
(c)def
swap
(other)def
toBase64
()def
toBase64
(options)def
toDouble
()def
toFloat
()def
toHex
()def
toHex
(separator)def
toInt
([, base=10])def
toLong
([, base=10])def
toLongLong
([, base=10])def
toLower
()def
toPercentEncoding
([exclude=QByteArray()[, include=QByteArray()[, percent=’%’]]])def
toShort
([, base=10])def
toUInt
([, base=10])def
toULong
([, base=10])def
toULongLong
([, base=10])def
toUShort
([, base=10])def
toUpper
()def
trimmed
()def
truncate
(pos)
Static functions¶
def
fromBase64
(base64)def
fromBase64
(base64, options)def
fromBase64Encoding
(base64[, options=QByteArray.Base64Encoding])def
fromBase64Encoding
(base64[, options=QByteArray.Base64Encoding])def
fromHex
(hexEncoded)def
fromPercentEncoding
(pctEncoded[, percent=’%’])def
fromRawData
(arg__1)def
number
(arg__1[, base=10])def
number
(arg__1[, base=10])def
number
(arg__1[, f=’g’[, prec=6]])
Detailed Description¶
QByteArray
can be used to store both raw bytes (including ‘\0’s) and traditional 8-bit ‘\0’-terminated strings. UsingQByteArray
is much more convenient than usingconst char *
. Behind the scenes, it always ensures that the data is followed by a ‘\0’ terminator, and uses implicit sharing (copy-on-write) to reduce memory usage and avoid needless copying of data.In addition to
QByteArray
, Qt also provides theQString
class to store string data. For most purposes,QString
is the class you want to use. It stores 16-bit Unicode characters, making it easy to store non-ASCII/non-Latin-1 characters in your application. Furthermore,QString
is used throughout in the Qt API. The two main cases whereQByteArray
is appropriate are when you need to store raw binary data, and when memory conservation is critical (e.g., with Qt for Embedded Linux).One way to initialize a
QByteArray
is simply to pass aconst char *
to its constructor. For example, the following code creates a byte array of size 5 containing the data “Hello”:ba = QByteArray("Hello")Although the
size()
is 5, the byte array also maintains an extra ‘\0’ character at the end so that if a function is used that asks for a pointer to the underlying data (e.g. a call todata()
), the data pointed to is guaranteed to be ‘\0’-terminated.
QByteArray
makes a deep copy of theconst char *
data, so you can modify it later without experiencing side effects. (If for performance reasons you don’t want to take a deep copy of the character data, usefromRawData()
instead.)Another approach is to set the size of the array using
resize()
and to initialize the data byte per byte.QByteArray
uses 0-based indexes, just like C++ arrays. To access the byte at a particular index position, you can use operator[](). On non-const byte arrays, operator[]() returns a reference to a byte that can be used on the left side of an assignment. For example:ba = QByteArray() ba.resize(5) ba[0] = 'H' ba[1] = 'e' ba[2] = 'l' ba[3] = 'l' ba[4] = 'o'For read-only access, an alternative syntax is to use
at()
:for i in range(0, ba.size()): if ba.at(i) >= 'a' and ba.at(i) <= 'f': print "Found character in range [a-f]"
at()
can be faster than operator[](), because it never causes a deep copy to occur.To extract many bytes at a time, use
left()
,right()
, ormid()
.A
QByteArray
can embed ‘\0’ bytes. Thesize()
function always returns the size of the whole array, including embedded ‘\0’ bytes, but excluding the terminating ‘\0’ added byQByteArray
. For example:QByteArray ba1("ca\0r\0t"); ba1.size(); // Returns 2. ba1.constData(); // Returns "ca" with terminating \0. QByteArray ba2("ca\0r\0t", 3); ba2.size(); // Returns 3. ba2.constData(); // Returns "ca\0" with terminating \0. QByteArray ba3("ca\0r\0t", 4); ba3.size(); // Returns 4. ba3.constData(); // Returns "ca\0r" with terminating \0. const char cart[] = {'c', 'a', '\0', 'r', '\0', 't'}; QByteArray ba4(QByteArray::fromRawData(cart, 6)); ba4.size(); // Returns 6. ba4.constData(); // Returns "ca\0r\0t" without terminating \0.If you want to obtain the length of the data up to and excluding the first ‘\0’ character, call
qstrlen()
on the byte array.After a call to
resize()
, newly allocated bytes have undefined values. To set all the bytes to a particular value, callfill()
.To obtain a pointer to the actual character data, call
data()
orconstData()
. These functions return a pointer to the beginning of the data. The pointer is guaranteed to remain valid until a non-const function is called on theQByteArray
. It is also guaranteed that the data ends with a ‘\0’ byte unless theQByteArray
was created from araw data
. This ‘\0’ byte is automatically provided byQByteArray
and is not counted insize()
.
QByteArray
provides the following basic functions for modifying the byte data:append()
,prepend()
,insert()
,replace()
, andremove()
. For example:x = QByteArray("and") x.prepend("rock ") # x == "rock and" x.append(" roll") # x == "rock and roll" x.replace(5, 3, "&") # x == "rock & roll"The
replace()
andremove()
functions’ first two arguments are the position from which to start erasing and the number of bytes that should be erased.When you
append()
data to a non-empty array, the array will be reallocated and the new data copied to it. You can avoid this behavior by callingreserve()
, which preallocates a certain amount of memory. You can also callcapacity()
to find out how much memoryQByteArray
actually allocated. Data appended to an empty array is not copied.A frequent requirement is to remove whitespace characters from a byte array (’\n’, ‘\t’, ‘ ‘, etc.). If you want to remove whitespace from both ends of a
QByteArray
, usetrimmed()
. If you want to remove whitespace from both ends and replace multiple consecutive whitespaces with a single space character within the byte array, usesimplified()
.If you want to find all occurrences of a particular character or substring in a
QByteArray
, useindexOf()
orlastIndexOf()
. The former searches forward starting from a given index position, the latter searches backward. Both return the index position of the character or substring if they find it; otherwise, they return -1. For example, here’s a typical loop that finds all occurrences of a particular substring:ba = QByteArray("We must be <b>bold</b>, very <b>bold</b>") j = 0 while (j = ba.indexOf("<b>", j)) != -1: print "Found <b> tag at index position %d" % j ++jIf you simply want to check whether a
QByteArray
contains a particular character or substring, usecontains()
. If you want to find out how many times a particular character or substring occurs in the byte array, usecount()
. If you want to replace all occurrences of a particular value with another, use one of the two-parameterreplace()
overloads.
QByteArray
s can be compared using overloaded operators such as operator<(), operator<=(), operator==(), operator>=(), and so on. The comparison is based exclusively on the numeric values of the characters and is very fast, but is not what a human would expect.localeAwareCompare()
is a better choice for sorting user-interface strings.For historical reasons,
QByteArray
distinguishes between a null byte array and an empty byte array. A null byte array is a byte array that is initialized usingQByteArray
‘s default constructor or by passing (const char *)0 to the constructor. An empty byte array is any byte array with size 0. A null byte array is always empty, but an empty byte array isn’t necessarily null:QByteArray().isNull() # returns true QByteArray().isEmpty() # returns true QByteArray("").isNull() # returns false QByteArray("").isEmpty() # returns true QByteArray("abc").isNull() # returns false QByteArray("abc").isEmpty() # returns falseAll functions except
isNull()
treat null byte arrays the same as empty byte arrays. For example,data()
returns a valid pointer (not nullptr) to a ‘\0’ character for a byte array andQByteArray()
compares equal toQByteArray
(“”). We recommend that you always useisEmpty()
and avoidisNull()
.
Maximum size and out-of-memory conditions¶
The current version of
QByteArray
is limited to just under 2 GB (2^31 bytes) in size. The exact value is architecture-dependent, since it depends on the overhead required for managing the data block, but is no more than 32 bytes. Raw data blocks are also limited by the use ofint
type in the current version to 2 GB minus 1 byte.In case memory allocation fails,
QByteArray
will throw astd::bad_alloc
exception. Out of memory conditions in the Qt containers are the only case where Qt will throw exceptions.Note that the operating system may impose further limits on applications holding a lot of allocated memory, especially large, contiguous blocks. Such considerations, the configuration of such behavior or any mitigation are outside the scope of the
QByteArray
API.
Notes on Locale¶
Number-String Conversions¶
Functions that perform conversions between numeric data types and strings are performed in the C locale, irrespective of the user’s locale settings. Use
QString
to perform locale-aware conversions between numbers and strings.
8-bit Character Comparisons¶
In
QByteArray
, the notion of uppercase and lowercase and of which character is greater than or less than another character is done in the Latin-1 locale. This affects functions that support a case insensitive option or that compare or lowercase or uppercase their arguments. Case insensitive operations and comparisons will be accurate if both strings contain only Latin-1 characters. Functions that this affects includecontains()
,indexOf()
,lastIndexOf()
, operator<(), operator<=(), operator>(), operator>=(),isLower()
,isUpper()
,toLower()
andtoUpper()
.This issue does not apply to
QString
s since they represent characters using Unicode.See also
QString
QBitArray
- class PySide2.QtCore.QByteArray¶
PySide2.QtCore.QByteArray(arg__1)
PySide2.QtCore.QByteArray(arg__1)
PySide2.QtCore.QByteArray(arg__1)
PySide2.QtCore.QByteArray(size, c)
- param size:
int
- param arg__1:
PyByteArray
- param c:
char
Constructs an empty byte array.
See also
Constructs a byte array of size
size
with every byte set to characterch
.See also
- PySide2.QtCore.QByteArray.Base64Option¶
This enum contains the options available for encoding and decoding Base64. Base64 is defined by RFC 4648 , with the following options:
Constant
Description
QByteArray.Base64Encoding
(default) The regular Base64 alphabet, called simply “base64”
QByteArray.Base64UrlEncoding
An alternate alphabet, called “base64url”, which replaces two characters in the alphabet to be more friendly to URLs.
QByteArray.KeepTrailingEquals
(default) Keeps the trailing padding equal signs at the end of the encoded data, so the data is always a size multiple of four.
QByteArray.OmitTrailingEquals
Omits adding the padding equal signs at the end of the encoded data.
QByteArray.IgnoreBase64DecodingErrors
When decoding Base64-encoded data, ignores errors in the input; invalid characters are simply skipped. This enum value has been added in Qt 5.15.
QByteArray.AbortOnBase64DecodingErrors
When decoding Base64-encoded data, stops at the first decoding error. This enum value has been added in Qt 5.15.
fromBase64Encoding()
andfromBase64()
ignore the and options. If the option is specified, they will not flag errors in case trailing equal signs are missing or if there are too many of them. If instead the is specified, then the input must either have no padding or have the correct amount of equal signs.
New in version 5.2.
- PySide2.QtCore.QByteArray.Base64DecodingStatus¶
New in version 5.15.
- PySide2.QtCore.QByteArray.__getitem__()¶
- PySide2.QtCore.QByteArray.__len__()¶
- PySide2.QtCore.QByteArray.__mgetitem__()¶
- PySide2.QtCore.QByteArray.__msetitem__()¶
- PySide2.QtCore.QByteArray.__reduce__()¶
- Return type:
object
- PySide2.QtCore.QByteArray.__repr__()¶
- Return type:
object
- PySide2.QtCore.QByteArray.__setitem__()¶
- PySide2.QtCore.QByteArray.__str__()¶
- Return type:
object
- PySide2.QtCore.QByteArray.append(count, c)¶
- Parameters:
count – int
c –
char
- Return type:
This is an overloaded function.
Appends
count
copies of characterch
to this byte array and returns a reference to this byte array.If
count
is negative or zero nothing is appended to the byte array.
- PySide2.QtCore.QByteArray.append(a)
- Parameters:
- Return type:
- PySide2.QtCore.QByteArray.append(c)
- Parameters:
c –
char
- Return type:
This is an overloaded function.
Appends the character
ch
to this byte array.
- PySide2.QtCore.QByteArray.at(i)¶
- Parameters:
i – int
- Return type:
char
Returns the character at index position
i
in the byte array.i
must be a valid index position in the byte array (i.e., 0 <=i
<size()
).See also
operator[]()
- PySide2.QtCore.QByteArray.back()¶
- Return type:
char
Returns the last character in the byte array. Same as
at(size() - 1)
.This function is provided for STL compatibility.
Warning
Calling this function on an empty byte array constitutes undefined behavior.
- PySide2.QtCore.QByteArray.capacity()¶
- Return type:
int
Returns the maximum number of bytes that can be stored in the byte array without forcing a reallocation.
The sole purpose of this function is to provide a means of fine tuning
QByteArray
‘s memory usage. In general, you will rarely ever need to call this function. If you want to know how many bytes are in the byte array, callsize()
.
- PySide2.QtCore.QByteArray.cbegin()¶
- Return type:
str
Returns a const STL-style iterator pointing to the first character in the byte-array.
See also
begin()
cend()
- PySide2.QtCore.QByteArray.cend()¶
- Return type:
str
Returns a const STL-style iterator pointing to the imaginary character after the last character in the list.
See also
cbegin()
end()
- PySide2.QtCore.QByteArray.chop(n)¶
- Parameters:
n – int
Removes
n
bytes from the end of the byte array.If
n
is greater thansize()
, the result is an empty byte array.Example:
ba = QByteArray("STARTTLS\r\n") ba.chop(2) # ba == "STARTTLS"
See also
- PySide2.QtCore.QByteArray.chopped(len)¶
- Parameters:
len – int
- Return type:
Returns a byte array that contains the leftmost
size()
-len
bytes of this byte array.Note
The behavior is undefined if
len
is negative or greater thansize()
.See also
- PySide2.QtCore.QByteArray.clear()¶
Clears the contents of the byte array and makes it null.
- PySide2.QtCore.QByteArray.compare(a[, cs=Qt.CaseSensitive])¶
- Parameters:
cs –
CaseSensitivity
- Return type:
int
- PySide2.QtCore.QByteArray.compare(c[, cs=Qt.CaseSensitive])
- Parameters:
c – str
cs –
CaseSensitivity
- Return type:
int
Returns an integer less than, equal to, or greater than zero depending on whether this
QByteArray
sorts before, at the same position, or after the string pointed to byc
. The comparison is performed according to case sensitivitycs
.See also
operator==
- PySide2.QtCore.QByteArray.contains(c)¶
- Parameters:
c –
char
- Return type:
bool
This is an overloaded function.
Returns
true
if the byte array contains the characterch
; otherwise returnsfalse
.
- PySide2.QtCore.QByteArray.contains(a)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.count()¶
- Return type:
int
This is an overloaded function.
Same as
size()
.
- PySide2.QtCore.QByteArray.count(c)
- Parameters:
c –
char
- Return type:
int
This is an overloaded function.
Returns the number of occurrences of character
ch
in the byte array.See also
- PySide2.QtCore.QByteArray.count(a)
- Parameters:
- Return type:
int
- PySide2.QtCore.QByteArray.data()¶
- Return type:
str
This is an overloaded function.
- PySide2.QtCore.QByteArray.endsWith(a)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.endsWith(c)
- Parameters:
c –
char
- Return type:
bool
This is an overloaded function.
Returns
true
if this byte array ends with characterch
; otherwise returnsfalse
.
- PySide2.QtCore.QByteArray.fill(c[, size=-1])¶
- Parameters:
c –
char
size – int
- Return type:
Sets every byte in the byte array to character
ch
. Ifsize
is different from -1 (the default), the byte array is resized to sizesize
beforehand.Example:
ba = QByteArray("Istambul") ba.fill('o') # ba == "oooooooo" ba.fill('X', 2) # ba == "XX"
See also
- static PySide2.QtCore.QByteArray.fromBase64(base64)¶
- Parameters:
base64 –
PySide2.QtCore.QByteArray
- Return type:
This is an overloaded function.
Returns a decoded copy of the Base64 array
base64
. Input is not checked for validity; invalid characters in the input are skipped, enabling the decoding process to continue with subsequent characters.For example:
text = QByteArray.fromBase64("UXQgaXMgZ3JlYXQh") text.data() # returns "Qt is great!"
The algorithm used to decode Base64-encoded data is defined in RFC 4648 .
Note
The
fromBase64Encoding()
function is recommended in new code.See also
- static PySide2.QtCore.QByteArray.fromBase64(base64, options)
- Parameters:
base64 –
PySide2.QtCore.QByteArray
options –
Base64Options
- Return type:
This is an overloaded function.
Returns a decoded copy of the Base64 array
base64
, using the options defined byoptions
. Ifoptions
containsIgnoreBase64DecodingErrors
(the default), the input is not checked for validity; invalid characters in the input are skipped, enabling the decoding process to continue with subsequent characters. Ifoptions
containsAbortOnBase64DecodingErrors
, then decoding will stop at the first invalid character.For example:
QByteArray::fromBase64("PHA+SGVsbG8/PC9wPg==", QByteArray::Base64Encoding); // returns "<p>Hello?</p>" QByteArray::fromBase64("PHA-SGVsbG8_PC9wPg==", QByteArray::Base64UrlEncoding); // returns "<p>Hello?</p>"
The algorithm used to decode Base64-encoded data is defined in RFC 4648 .
Returns the decoded data, or, if the
AbortOnBase64DecodingErrors
option was passed and the input data was invalid, an empty byte array.Note
The
fromBase64Encoding()
function is recommended in new code.See also
- static PySide2.QtCore.QByteArray.fromBase64Encoding(base64[, options=QByteArray.Base64Encoding])¶
- Parameters:
base64 –
PySide2.QtCore.QByteArray
options –
Base64Options
- Return type:
- static PySide2.QtCore.QByteArray.fromBase64Encoding(base64[, options=QByteArray.Base64Encoding])
- Parameters:
base64 –
PySide2.QtCore.QByteArray
options –
Base64Options
- Return type:
- static PySide2.QtCore.QByteArray.fromHex(hexEncoded)¶
- Parameters:
hexEncoded –
PySide2.QtCore.QByteArray
- Return type:
Returns a decoded copy of the hex encoded array
hexEncoded
. Input is not checked for validity; invalid characters in the input are skipped, enabling the decoding process to continue with subsequent characters.For example:
text = QByteArray.fromHex("517420697320677265617421") text.data() # returns "Qt is great!"
See also
- static PySide2.QtCore.QByteArray.fromPercentEncoding(pctEncoded[, percent='%'])¶
- Parameters:
pctEncoded –
PySide2.QtCore.QByteArray
percent –
char
- Return type:
Returns a decoded copy of the URI/URL-style percent-encoded
input
. Thepercent
parameter allows you to replace the ‘%’ character for another (for instance, ‘_’ or ‘=’).For example:
QByteArray text = QByteArray::fromPercentEncoding("Qt%20is%20great%33"); text.data(); // returns "Qt is great!"
Note
Given invalid input (such as a string containing the sequence “%G5”, which is not a valid hexadecimal number) the output will be invalid as well. As an example: the sequence “%G5” could be decoded to ‘W’.
See also
- static PySide2.QtCore.QByteArray.fromRawData(arg__1)¶
- Parameters:
arg__1 – str
- Return type:
Constructs a
QByteArray
that uses the firstsize
bytes of thedata
array. The bytes are not copied. TheQByteArray
will contain thedata
pointer. The caller guarantees thatdata
will not be deleted or modified as long as thisQByteArray
and any copies of it exist that have not been modified. In other words, becauseQByteArray
is an implicitly shared class and the instance returned by this function contains thedata
pointer, the caller must not deletedata
or modify it directly as long as the returnedQByteArray
and any copies exist. However,QByteArray
does not take ownership ofdata
, so theQByteArray
destructor will never delete the rawdata
, even when the lastQByteArray
referring todata
is destroyed.A subsequent attempt to modify the contents of the returned
QByteArray
or any copy made from it will cause it to create a deep copy of thedata
array before doing the modification. This ensures that the rawdata
array itself will never be modified byQByteArray
.Here is an example of how to read data using a
QDataStream
on raw data in memory without copying the raw data into aQByteArray
:mydata = '\x00\x00\x03\x84\x78\x9c\x3b\x76'\ '\xec\x18\xc3\x31\x0a\xf1\xcc\x99'\ ... '\x6d\x5b' data = QByteArray.fromRawData(mydata) in_ = QDataStream(data, QIODevice.ReadOnly) ...
Warning
A byte array created with is not ‘\0’-terminated, unless the raw data contains a 0 character at position
size
. While that does not matter forQDataStream
or functions likeindexOf()
, passing the byte array to a function accepting aconst char *
expected to be ‘\0’-terminated will fail.See also
setRawData()
data()
constData()
- PySide2.QtCore.QByteArray.front()¶
- Return type:
char
Returns the first character in the byte array. Same as
at(0)
.This function is provided for STL compatibility.
Warning
Calling this function on an empty byte array constitutes undefined behavior.
- PySide2.QtCore.QByteArray.indexOf(a[, from=0])¶
- Parameters:
from – int
- Return type:
int
- PySide2.QtCore.QByteArray.insert(i, a)¶
- Parameters:
i – int
- Return type:
- PySide2.QtCore.QByteArray.insert(i, count, c)
- Parameters:
i – int
count – int
c –
char
- Return type:
This is an overloaded function.
Inserts
count
copies of characterch
at index positioni
in the byte array.If
i
is greater thansize()
, the array is first extended usingresize()
.
- PySide2.QtCore.QByteArray.isEmpty()¶
- Return type:
bool
Returns
true
if the byte array has size 0; otherwise returnsfalse
.Example:
QByteArray().isEmpty() # returns true QByteArray("").isEmpty() # returns true QByteArray("abc").isEmpty() # returns false
See also
- PySide2.QtCore.QByteArray.isLower()¶
- Return type:
bool
Returns
true
if this byte array contains only lowercase letters, otherwise returnsfalse
. The byte array is interpreted as a Latin-1 encoded string.
- PySide2.QtCore.QByteArray.isNull()¶
- Return type:
bool
Returns
true
if this byte array is null; otherwise returnsfalse
.Example:
QByteArray().isNull() # returns true QByteArray("").isNull() # returns false QByteArray("abc").isNull() # returns false
Qt makes a distinction between null byte arrays and empty byte arrays for historical reasons. For most applications, what matters is whether or not a byte array contains any data, and this can be determined using
isEmpty()
.See also
- Parameters:
other –
PySide2.QtCore.QByteArray
- Return type:
bool
- PySide2.QtCore.QByteArray.isUpper()¶
- Return type:
bool
Returns
true
if this byte array contains only uppercase letters, otherwise returnsfalse
. The byte array is interpreted as a Latin-1 encoded string.
- PySide2.QtCore.QByteArray.lastIndexOf(a[, from=-1])¶
- Parameters:
from – int
- Return type:
int
- PySide2.QtCore.QByteArray.left(len)¶
- Parameters:
len – int
- Return type:
Returns a byte array that contains the leftmost
len
bytes of this byte array.The entire byte array is returned if
len
is greater thansize()
.Example:
x = QByteArray("Pineapple") y = x.left(4) # y == "Pine"
See also
- PySide2.QtCore.QByteArray.leftJustified(width[, fill=' '[, truncate=false]])¶
- Parameters:
width – int
fill –
char
truncate – bool
- Return type:
Returns a byte array of size
width
that contains this byte array padded by thefill
character.If
truncate
is false and thesize()
of the byte array is more thanwidth
, then the returned byte array is a copy of this byte array.If
truncate
is true and thesize()
of the byte array is more thanwidth
, then any bytes in a copy of the byte array after positionwidth
are removed, and the copy is returned.Example:
x = QByteArray("apple") y = x.leftJustified(8, '.') # y == "apple..."
See also
- PySide2.QtCore.QByteArray.mid(index[, len=-1])¶
- Parameters:
index – int
len – int
- Return type:
Returns a byte array containing
len
bytes from this byte array, starting at positionpos
.If
len
is -1 (the default), orpos
+len
>=size()
, returns a byte array containing all bytes starting at positionpos
until the end of the byte array.Example:
x = QByteArray("Five pineapples") y = x.mid(5, 4) # y == "pine" z = x.mid(5) # z == "pineapples"
See also
- static PySide2.QtCore.QByteArray.number(arg__1[, f='g'[, prec=6]])¶
- Parameters:
arg__1 –
double
f –
char
prec – int
- Return type:
This is an overloaded function.
Returns a byte array that contains the printed value of
n
, formatted in formatf
with precisionprec
.Argument
n
is formatted according to thef
format specified, which isg
by default, and can be any of the following:Format
Meaning
e
format as [-]9.9e[+|-]999
E
format as [-]9.9E[+|-]999
f
format as [-]9.9
g
use
e
orf
format, whichever is the most conciseG
use
E
orf
format, whichever is the most conciseWith ‘e’, ‘E’, and ‘f’,
prec
is the number of digits after the decimal point. With ‘g’ and ‘G’,prec
is the maximum number of significant digits (trailing zeroes are omitted).ba = QByteArray.number(12.3456, 'E', 3) # ba == 1.235E+01
Note
The format of the number is not localized; the default C locale is used irrespective of the user’s locale.
See also
- static PySide2.QtCore.QByteArray.number(arg__1[, base=10])
- Parameters:
arg__1 – int
base – int
- Return type:
Returns a byte array containing the string equivalent of the number
n
to basebase
(10 by default). Thebase
can be any value between 2 and 36.Example:
n = 63; QByteArray.number(n) # returns "63" QByteArray.number(n, 16) # returns "3f" QByteArray.number(n, 16).toUpper() # returns "3F"
Note
The format of the number is not localized; the default C locale is used irrespective of the user’s locale.
- static PySide2.QtCore.QByteArray.number(arg__1[, base=10])
- Parameters:
arg__1 –
qlonglong
base – int
- Return type:
This is an overloaded function.
See also
- PySide2.QtCore.QByteArray.__ne__(arg__1)¶
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.__ne__(a2)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__ne__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__ne__(rhs)
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__add__(a2)¶
- Parameters:
- Return type:
- PySide2.QtCore.QByteArray.__add__(a2)
- Parameters:
a2 –
char
- Return type:
- PySide2.QtCore.QByteArray.__add__(arg__1)
- Parameters:
arg__1 –
PyBytes
- PySide2.QtCore.QByteArray.__add__(arg__1)
- Parameters:
arg__1 –
PyByteArray
- Return type:
- PySide2.QtCore.QByteArray.__add__(arg__1)
- Parameters:
arg__1 –
PyByteArray
- Return type:
- PySide2.QtCore.QByteArray.__iadd__(arg__1)¶
- Parameters:
arg__1 –
PyByteArray
- Return type:
- PySide2.QtCore.QByteArray.__iadd__(c)
- Parameters:
c –
char
- Return type:
This is an overloaded function.
Appends the character
ch
onto the end of this byte array and returns a reference to this byte array.
- PySide2.QtCore.QByteArray.__iadd__(a)
- Parameters:
- Return type:
- PySide2.QtCore.QByteArray.__lt__(a2)¶
- Parameters:
a2 – str
- Return type:
bool
- PySide2.QtCore.QByteArray.__lt__(rhs)
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__lt__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__lt__(a2)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__lt__(arg__1)
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.__le__(rhs)¶
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__le__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__le__(a2)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__le__(arg__1)
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.operator=(str)
- Parameters:
str – str
- Return type:
This is an overloaded function.
Assigns
str
to this byte array.
- PySide2.QtCore.QByteArray.__eq__(rhs)¶
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__eq__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__eq__(a2)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__eq__(arg__1)
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.__gt__(arg__1)¶
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.__gt__(a2)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__gt__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__gt__(rhs)
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__ge__(a2)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.__ge__(rhs)
- Parameters:
rhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.__ge__(arg__1)
- Parameters:
arg__1 –
PyUnicode
- PySide2.QtCore.QByteArray.__ge__(lhs)
- Parameters:
lhs –
QStringRef
- Return type:
bool
- PySide2.QtCore.QByteArray.prepend(c)¶
- Parameters:
c –
char
- Return type:
This is an overloaded function.
Prepends the character
ch
to this byte array.
- PySide2.QtCore.QByteArray.prepend(a)
- Parameters:
- Return type:
- PySide2.QtCore.QByteArray.prepend(count, c)
- Parameters:
count – int
c –
char
- Return type:
This is an overloaded function.
Prepends
count
copies of characterch
to this byte array.
- PySide2.QtCore.QByteArray.remove(index, len)¶
- Parameters:
index – int
len – int
- Return type:
Removes
len
bytes from the array, starting at index positionpos
, and returns a reference to the array.If
pos
is out of range, nothing happens. Ifpos
is valid, butpos
+len
is larger than the size of the array, the array is truncated at positionpos
.Example:
ba = QByteArray("Montreal") ba.remove(1, 4) # ba == "Meal"
- PySide2.QtCore.QByteArray.repeated(times)¶
- Parameters:
times – int
- Return type:
Returns a copy of this byte array repeated the specified number of
times
.If
times
is less than 1, an empty byte array is returned.Example:
QByteArray ba("ab"); ba.repeated(4); // returns "abababab"
- PySide2.QtCore.QByteArray.replace(index, len, s)¶
- Parameters:
index – int
len – int
- Return type:
- PySide2.QtCore.QByteArray.replace(before, after)
- Parameters:
before – str
after –
PySide2.QtCore.QByteArray
- Return type:
Note
This function is deprecated.
- PySide2.QtCore.QByteArray.replace(before, after)
- Parameters:
before –
char
after –
char
- Return type:
This is an overloaded function.
Replaces every occurrence of the character
before
with the characterafter
.
- PySide2.QtCore.QByteArray.replace(before, after)
- Parameters:
before –
char
after –
PySide2.QtCore.QByteArray
- Return type:
- PySide2.QtCore.QByteArray.replace(before, after)
- Parameters:
before –
PySide2.QtCore.QByteArray
after –
PySide2.QtCore.QByteArray
- Return type:
- PySide2.QtCore.QByteArray.reserve(size)¶
- Parameters:
size – int
Attempts to allocate memory for at least
size
bytes. If you know in advance how large the byte array will be, you can call this function, and if you callresize()
often you are likely to get better performance. Ifsize
is an underestimate, the worst that will happen is that theQByteArray
will be a bit slower.The sole purpose of this function is to provide a means of fine tuning
QByteArray
‘s memory usage. In general, you will rarely ever need to call this function. If you want to change the size of the byte array, callresize()
.See also
- PySide2.QtCore.QByteArray.resize(size)¶
- Parameters:
size – int
Sets the size of the byte array to
size
bytes.If
size
is greater than the current size, the byte array is extended to make itsize
bytes with the extra bytes added to the end. The new bytes are uninitialized.If
size
is less than the current size, bytes are removed from the end.See also
- PySide2.QtCore.QByteArray.right(len)¶
- Parameters:
len – int
- Return type:
Returns a byte array that contains the rightmost
len
bytes of this byte array.The entire byte array is returned if
len
is greater thansize()
.Example:
x = QByteArray("Pineapple") y = x.right(5) # y == "apple"
See also
- PySide2.QtCore.QByteArray.rightJustified(width[, fill=' '[, truncate=false]])¶
- Parameters:
width – int
fill –
char
truncate – bool
- Return type:
Returns a byte array of size
width
that contains thefill
character followed by this byte array.If
truncate
is false and the size of the byte array is more thanwidth
, then the returned byte array is a copy of this byte array.If
truncate
is true and the size of the byte array is more thanwidth
, then the resulting byte array is truncated at positionwidth
.Example:
x = QByteArray("apple") y = x.rightJustified(8, '.') # y == "...apple"
See also
- PySide2.QtCore.QByteArray.setNum(arg__1[, base=10])¶
- Parameters:
arg__1 –
qlonglong
base – int
- Return type:
This is an overloaded function.
See also
- PySide2.QtCore.QByteArray.setNum(arg__1[, base=10])
- Parameters:
arg__1 – int
base – int
- Return type:
Sets the byte array to the printed value of
n
in basebase
(10 by default) and returns a reference to the byte array. Thebase
can be any value between 2 and 36. For bases other than 10, n is treated as an unsigned integer.Example:
ba = QByteArray() n = 63 ba.setNum(n) # ba == "63" ba.setNum(n, 16) # ba == "3f"
Note
The format of the number is not localized; the default C locale is used irrespective of the user’s locale.
- PySide2.QtCore.QByteArray.setNum(arg__1[, f='g'[, prec=6]])
- Parameters:
arg__1 –
double
f –
char
prec – int
- Return type:
This is an overloaded function.
Sets the byte array to the printed value of
n
, formatted in formatf
with precisionprec
, and returns a reference to the byte array.The format
f
can be any of the following:Format
Meaning
e
format as [-]9.9e[+|-]999
E
format as [-]9.9E[+|-]999
f
format as [-]9.9
g
use
e
orf
format, whichever is the most conciseG
use
E
orf
format, whichever is the most conciseWith ‘e’, ‘E’, and ‘f’,
prec
is the number of digits after the decimal point. With ‘g’ and ‘G’,prec
is the maximum number of significant digits (trailing zeroes are omitted).Note
The format of the number is not localized; the default C locale is used irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.setRawData(a, n)¶
- Parameters:
a – str
n –
uint
- Return type:
Resets the
QByteArray
to use the firstsize
bytes of thedata
array. The bytes are not copied. TheQByteArray
will contain thedata
pointer. The caller guarantees thatdata
will not be deleted or modified as long as thisQByteArray
and any copies of it exist that have not been modified.This function can be used instead of
fromRawData()
to re-use existingQByteArray
objects to save memory re-allocations.See also
fromRawData()
data()
constData()
- PySide2.QtCore.QByteArray.shrink_to_fit()¶
This function is provided for STL compatibility. It is equivalent to
squeeze()
.
- PySide2.QtCore.QByteArray.simplified()¶
- Return type:
Returns a byte array that has whitespace removed from the start and the end, and which has each sequence of internal whitespace replaced with a single space.
Whitespace means any character for which the standard C++
isspace()
function returnstrue
in the C locale. This includes the ASCII isspace() function returnstrue
in the C locale. This includes the ASCII characters ‘\t’, ‘\n’, ‘\v’, ‘\f’, ‘\r’, and ‘ ‘.Example:
ba = QByteArray(" lots\t of\nwhitespace\r\n ") ba = ba.simplified() # ba == "lots of whitespace";
See also
- PySide2.QtCore.QByteArray.size()¶
- Return type:
int
Returns the number of bytes in this byte array.
The last byte in the byte array is at position - 1. In addition,
QByteArray
ensures that the byte at position is always ‘\0’, so that you can use the return value ofdata()
andconstData()
as arguments to functions that expect ‘\0’-terminated strings. If theQByteArray
object was created from araw data
that didn’t include the trailing null-termination character thenQByteArray
doesn’t add it automatically unless the deep copy is created.Example:
ba = QByteArray("Hello") n = ba.size() # n == 5 ba.data()[0] # returns 'H' ba.data()[4] # returns 'o'
- PySide2.QtCore.QByteArray.split(sep)¶
- Parameters:
sep –
char
- Return type:
Splits the byte array into subarrays wherever
sep
occurs, and returns the list of those arrays. Ifsep
does not match anywhere in the byte array, returns a single-element list containing this byte array.
- PySide2.QtCore.QByteArray.squeeze()¶
Releases any memory not required to store the array’s data.
The sole purpose of this function is to provide a means of fine tuning
QByteArray
‘s memory usage. In general, you will rarely ever need to call this function.See also
- PySide2.QtCore.QByteArray.startsWith(c)¶
- Parameters:
c –
char
- Return type:
bool
This is an overloaded function.
Returns
true
if this byte array starts with characterch
; otherwise returnsfalse
.
- PySide2.QtCore.QByteArray.startsWith(a)
- Parameters:
- Return type:
bool
- PySide2.QtCore.QByteArray.swap(other)¶
- Parameters:
other –
PySide2.QtCore.QByteArray
Swaps byte array
other
with this byte array. This operation is very fast and never fails.
- PySide2.QtCore.QByteArray.toBase64()¶
- Return type:
Returns a copy of the byte array, encoded as Base64.
text = QByteArray("Qt is great!") text.toBase64() # returns "UXQgaXMgZ3JlYXQh"
The algorithm used to encode Base64-encoded data is defined in RFC 4648 .
See also
- PySide2.QtCore.QByteArray.toBase64(options)
- Parameters:
options –
Base64Options
- Return type:
This is an overloaded function.
Returns a copy of the byte array, encoded using the options
options
.QByteArray text("<p>Hello?</p>"); text.toBase64(QByteArray::Base64Encoding | QByteArray::OmitTrailingEquals); // returns "PHA+SGVsbG8/PC9wPg" text.toBase64(QByteArray::Base64Encoding); // returns "PHA+SGVsbG8/PC9wPg==" text.toBase64(QByteArray::Base64UrlEncoding); // returns "PHA-SGVsbG8_PC9wPg==" text.toBase64(QByteArray::Base64UrlEncoding | QByteArray::OmitTrailingEquals); // returns "PHA-SGVsbG8_PC9wPg"
The algorithm used to encode Base64-encoded data is defined in RFC 4648 .
See also
- PySide2.QtCore.QByteArray.toDouble()¶
- Return type:
double
Returns the byte array converted to a
double
value.Returns an infinity if the conversion overflows or 0.0 if the conversion fails for other reasons (e.g. underflow).
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.string = QByteArray("1234.56") (a, ok) = string.toDouble() # a == 1234.56, ok == true
Warning
The
QByteArray
content may only contain valid numerical characters which includes the plus/minus sign, the character e used in scientific notation, and the decimal point. Including the unit or additional characters leads to a conversion error.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
This function ignores leading and trailing whitespace.
See also
- PySide2.QtCore.QByteArray.toFloat()¶
- Return type:
float
Returns the byte array converted to a
float
value.Returns an infinity if the conversion overflows or 0.0 if the conversion fails for other reasons (e.g. underflow).
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.QByteArray string("1234.56"); bool ok; float a = string.toFloat(&ok); // a == 1234.56, ok == true string = "1234.56 Volt"; a = str.toFloat(&ok); // a == 0, ok == false
Warning
The
QByteArray
content may only contain valid numerical characters which includes the plus/minus sign, the character e used in scientific notation, and the decimal point. Including the unit or additional characters leads to a conversion error.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
This function ignores leading and trailing whitespace.
See also
- PySide2.QtCore.QByteArray.toHex()¶
- Return type:
Returns a hex encoded copy of the byte array. The hex encoding uses the numbers 0-9 and the letters a-f.
See also
- PySide2.QtCore.QByteArray.toHex(separator)
- Parameters:
separator –
char
- Return type:
This is an overloaded function.
Returns a hex encoded copy of the byte array. The hex encoding uses the numbers 0-9 and the letters a-f.
If
separator
is not ‘\0’, the separator character is inserted between the hex bytes.Example:
QByteArray macAddress = QByteArray::fromHex("123456abcdef"); macAddress.toHex(':'); // returns "12:34:56:ab:cd:ef" macAddress.toHex(0); // returns "123456abcdef"
See also
- PySide2.QtCore.QByteArray.toInt([base=10])¶
- Parameters:
base – int
- Return type:
int
Returns the byte array converted to an
int
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.string = QByteArray("FF") (hex, ok) = string.toInt(16) # hex == 255, ok == true (dec, ok) = string.toInt(10) # dec == 0, ok == false
Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toLong([base=10])¶
- Parameters:
base – int
- Return type:
long
Returns the byte array converted to a
long
int using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.string = QByteArray("FF") (hex, ok) = str.toLong(16); # hex == 255, ok == true (dec, ok) = str.toLong(10); # dec == 0, ok == false
Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toLongLong([base=10])¶
- Parameters:
base – int
- Return type:
qlonglong
Returns the byte array converted to a
long long
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toLower()¶
- Return type:
Returns a lowercase copy of the byte array. The bytearray is interpreted as a Latin-1 encoded string.
Example:
x = QByteArray("Qt by THE QT COMPANY") y = x.toLower() # y == "qt by the qt company"
- PySide2.QtCore.QByteArray.toPercentEncoding([exclude=QByteArray()[, include=QByteArray()[, percent='%']]])¶
- Parameters:
exclude –
PySide2.QtCore.QByteArray
include –
PySide2.QtCore.QByteArray
percent –
char
- Return type:
Returns a URI/URL-style percent-encoded copy of this byte array. The
percent
parameter allows you to override the default ‘%’ character for another.By default, this function will encode all characters that are not one of the following:
ALPHA (“a” to “z” and “A” to “Z”) / DIGIT (0 to 9) / “-” / “.” / “_” / “~”
To prevent characters from being encoded pass them to
exclude
. To force characters to be encoded pass them toinclude
. Thepercent
character is always encoded.Example:
QByteArray text = "{a fishy string?}"; QByteArray ba = text.toPercentEncoding("{}", "s"); qDebug(ba.constData()); // prints "{a fi%73hy %73tring%3F}"
The hex encoding uses the numbers 0-9 and the uppercase letters A-F.
See also
- PySide2.QtCore.QByteArray.toShort([base=10])¶
- Parameters:
base – int
- Return type:
short
Returns the byte array converted to a
short
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toUInt([base=10])¶
- Parameters:
base – int
- Return type:
uint
Returns the byte array converted to an
unsigned int
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toULong([base=10])¶
- Parameters:
base – int
- Return type:
ulong
Returns the byte array converted to an
unsigned long int
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toULongLong([base=10])¶
- Parameters:
base – int
- Return type:
qulonglong
Returns the byte array converted to an
unsigned long long
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toUShort([base=10])¶
- Parameters:
base – int
- Return type:
ushort
Returns the byte array converted to an
unsigned short
using basebase
, which is 10 by default and must be between 2 and 36, or 0.If
base
is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal; if it begins with “0”, it is assumed to be octal; otherwise it is assumed to be decimal.Returns 0 if the conversion fails.
If
ok
is notNone
, failure is reported by setting *``ok`` tofalse
, and success by setting *``ok`` totrue
.Note
The conversion of the number is performed in the default C locale, irrespective of the user’s locale.
See also
- PySide2.QtCore.QByteArray.toUpper()¶
- Return type:
Returns an uppercase copy of the byte array. The bytearray is interpreted as a Latin-1 encoded string.
Example:
x = QByteArray("Qt by THE QT COMPANY") y = x.toUpper() # y == "QT BY THE QT COMPANY"
- PySide2.QtCore.QByteArray.trimmed()¶
- Return type:
Returns a byte array that has whitespace removed from the start and the end.
Whitespace means any character for which the standard C++
isspace()
function returnstrue
in the C locale. This includes the ASCII characters ‘\t’, ‘\n’, ‘\v’, ‘\f’, ‘\r’, and ‘ ‘.Example:
ba = QByteArray(" lots\t of\nwhitespace\r\n "); ba = ba.trimmed(); # ba == "lots\t of\nwhitespace";
Unlike
simplified()
, leaves internal whitespace alone.See also
- PySide2.QtCore.QByteArray.truncate(pos)¶
- Parameters:
pos – int
Truncates the byte array at index position
pos
.If
pos
is beyond the end of the array, nothing happens.Example:
ba = QByteArray("Stockholm") ba.truncate(5) # ba == "Stock"
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.