QCollator¶
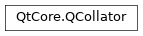
New in version 5.2.
Synopsis¶
Functions¶
def
caseSensitivity
()def
compare
(s1, len1, s2, len2)def
compare
(s1, s2)def
compare
(s1, s2)def
ignorePunctuation
()def
locale
()def
numericMode
()def
operator()
(s1, s2)def
setCaseSensitivity
(cs)def
setIgnorePunctuation
(on)def
setLocale
(locale)def
setNumericMode
(on)def
sortKey
(string)def
swap
(other)
Detailed Description¶
QCollator
is initialized with aQLocale
and an optional collation strategy. It tries to initialize the collator with the specified values. The collator can then be used to compare and sort strings in a locale dependent fashion.A
QCollator
object can be used together with template based sorting algorithms such as std::sort to sort a list of QStrings.In addition to the locale and collation strategy, several optional flags can be set that influence the result of the collation.
- class PySide2.QtCore.QCollator¶
PySide2.QtCore.QCollator(arg__1)
PySide2.QtCore.QCollator(locale)
- param locale:
- param arg__1:
Constructs a
QCollator
using the default locale’s collation locale.The system locale, when used as default locale, may have a collation locale other than itself (e.g. on Unix, if LC_COLLATE is set differently to LANG in the environment). All other locales are their own collation locales.
See also
- PySide2.QtCore.QCollator.caseSensitivity()¶
- Return type:
Returns case sensitivity of the collator.
See also
- PySide2.QtCore.QCollator.compare(s1, len1, s2, len2)¶
- Parameters:
s1 –
QChar
len1 – int
s2 –
QChar
len2 – int
- Return type:
int
This is an overloaded function.
Compares
s1
withs2
.len1
andlen2
specify the lengths of theQChar
arrays pointed to bys1
ands2
.Returns an integer less than, equal to, or greater than zero depending on whether
s1
sorts before, with or afters2
.
- PySide2.QtCore.QCollator.compare(s1, s2)
- Parameters:
s1 – str
s2 – str
- Return type:
int
- PySide2.QtCore.QCollator.compare(s1, s2)
- Parameters:
s1 –
QStringRef
s2 –
QStringRef
- Return type:
int
- PySide2.QtCore.QCollator.ignorePunctuation()¶
- Return type:
bool
Returns
true
if punctuation characters and symbols are ignored when determining sort order.See also
- PySide2.QtCore.QCollator.locale()¶
- Return type:
Returns the locale of the collator.
See also
- PySide2.QtCore.QCollator.numericMode()¶
- Return type:
bool
Returns
true
if numeric sorting is enabled, false otherwise.See also
- PySide2.QtCore.QCollator.operator()(s1, s2)¶
- Parameters:
s1 – str
s2 – str
- Return type:
bool
- PySide2.QtCore.QCollator.setCaseSensitivity(cs)¶
- Parameters:
cs –
CaseSensitivity
Sets the case
sensitivity
of the collator.See also
- PySide2.QtCore.QCollator.setIgnorePunctuation(on)¶
- Parameters:
on – bool
If
on
is set to true, punctuation characters and symbols are ignored when determining sort order.The default is locale dependent.
Note
This method is not currently supported if Qt is configured to not use ICU on Linux.
See also
- PySide2.QtCore.QCollator.setLocale(locale)¶
- Parameters:
locale –
PySide2.QtCore.QLocale
Sets the locale of the collator to
locale
.See also
- PySide2.QtCore.QCollator.setNumericMode(on)¶
- Parameters:
on – bool
Enables numeric sorting mode when
on
is set to true.This will enable proper sorting of numeric digits, so that e.g. 100 sorts after 99.
By default this mode is off.
See also
- PySide2.QtCore.QCollator.sortKey(string)¶
- Parameters:
string – str
- Return type:
Returns a for
string
.Creating the sort key is usually somewhat slower, than using the
compare()
methods directly. But if the string is compared repeatedly (e.g. when sorting a whole list of strings), it’s usually faster to create the sort keys for each string and then sort using the keys.Note
Not supported with the C (a.k.a. POSIX) locale on Darwin.
- PySide2.QtCore.QCollator.swap(other)¶
- Parameters:
other –
PySide2.QtCore.QCollator
Swaps this collator with
other
. This function is very fast and never fails.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.