QDirIterator¶
The
QDirIterator
class provides an iterator for directory entrylists. More…
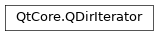
Synopsis¶
Functions¶
Detailed Description¶
You can use
QDirIterator
to navigate entries of a directory one at a time. It is similar toentryList()
andentryInfoList()
, but because it lists entries one at a time instead of all at once, it scales better and is more suitable for large directories. It also supports listing directory contents recursively, and following symbolic links. UnlikeentryList()
,QDirIterator
does not support sorting.The
QDirIterator
constructor takes aQDir
or a directory as argument. After construction, the iterator is located before the first directory entry. Here’s how to iterate over all the entries sequentially:it = QDirIterator("/etc", QDirIterator.Subdirectories) while it.hasNext(): print it.next() # /etc/. # /etc/.. # /etc/X11 # /etc/X11/fs # ...Here’s how to find and read all files filtered by name, recursively:
QDirIterator it("/sys", QStringList() << "scaling_cur_freq", QDir::NoFilter, QDirIterator::Subdirectories); while (it.hasNext()) { QFile f(it.next()); f.open(QIODevice::ReadOnly); qDebug() << f.fileName() << f.readAll().trimmed().toDouble() / 1000 << "MHz"; }The
next()
function returns the path to the next directory entry and advances the iterator. You can also callfilePath()
to get the current file path without advancing the iterator. ThefileName()
function returns only the name of the file, similar to howentryList()
works. You can also callfileInfo()
to get aQFileInfo
for the current entry.Unlike Qt’s container iterators,
QDirIterator
is uni-directional (i.e., you cannot iterate directories in reverse order) and does not allow random access.See also
- class PySide2.QtCore.QDirIterator(dir[, flags=QDirIterator.NoIteratorFlags])¶
PySide2.QtCore.QDirIterator(path, filter[, flags=QDirIterator.NoIteratorFlags])
PySide2.QtCore.QDirIterator(path[, flags=QDirIterator.NoIteratorFlags])
PySide2.QtCore.QDirIterator(path, nameFilters[, filters=QDir.NoFilter[, flags=QDirIterator.NoIteratorFlags]])
- param filter:
Filters
- param dir:
- param path:
str
- param nameFilters:
list of strings
- param filters:
Filters
- param flags:
IteratorFlags
Constructs a
QDirIterator
that can iterate overpath
, with no name filtering andfilters
for entry filtering. You can pass options viaflags
to decide how the directory should be iterated.By default,
filters
isNoFilter
, andflags
isNoIteratorFlags
, which provides the same behavior as inentryList()
.Note
To list symlinks that point to non existing files,
System
must be passed to the flags.Constructs a
QDirIterator
that can iterate overpath
, usingnameFilters
andfilters
. You can pass options viaflags
to decide how the directory should be iterated.By default,
flags
isNoIteratorFlags
, which provides the same behavior asentryList()
.For example, the following iterator could be used to iterate over audio files:
QDirIterator audioFileIt(audioPath, {"*.mp3", "*.wav"}, QDir::Files);
Note
To list symlinks that point to non existing files,
System
must be passed to the flags.See also
hasNext()
next()
IteratorFlags
setNameFilters()
- PySide2.QtCore.QDirIterator.IteratorFlag¶
This enum describes flags that you can combine to configure the behavior of
QDirIterator
.Constant
Description
QDirIterator.NoIteratorFlags
The default value, representing no flags. The iterator will return entries for the assigned path.
QDirIterator.Subdirectories
List entries inside all subdirectories as well.
QDirIterator.FollowSymlinks
When combined with Subdirectories, this flag enables iterating through all subdirectories of the assigned path, following all symbolic links. Symbolic link loops (e.g., “link” => “.” or “link” => “..”) are automatically detected and ignored.
- PySide2.QtCore.QDirIterator.fileInfo()¶
- Return type:
Returns a
QFileInfo
for the current directory entry.See also
- PySide2.QtCore.QDirIterator.fileName()¶
- Return type:
str
Returns the file name for the current directory entry, without the path prepended.
This function is convenient when iterating a single directory. When using the
Subdirectories
flag, you can usefilePath()
to get the full path.See also
- PySide2.QtCore.QDirIterator.filePath()¶
- Return type:
str
Returns the full file path for the current directory entry.
See also
- PySide2.QtCore.QDirIterator.hasNext()¶
- Return type:
bool
Returns
true
if there is at least one more entry in the directory; otherwise, false is returned.See also
- PySide2.QtCore.QDirIterator.next()¶
- Return type:
str
Advances the iterator to the next entry, and returns the file path of this new entry. If
hasNext()
returnsfalse
, this function does nothing, and returns an emptyQString
.You can call
fileName()
orfilePath()
to get the current entry file name or path, orfileInfo()
to get aQFileInfo
for the current entry.See also
- PySide2.QtCore.QDirIterator.path()¶
- Return type:
str
Returns the base directory of the iterator.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.