QPageLayout¶
Describes the size, orientation and margins of a page. More…
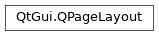
Synopsis¶
Functions¶
def
__eq__
(, rhs)def
__ne__
(, rhs)def
fullRect
()def
fullRect
(units)def
fullRectPixels
(resolution)def
fullRectPoints
()def
isEquivalentTo
(other)def
isValid
()def
margins
()def
margins
(units)def
marginsPixels
(resolution)def
marginsPoints
()def
maximumMargins
()def
minimumMargins
()def
mode
()def
orientation
()def
pageSize
()def
paintRect
()def
paintRect
(units)def
paintRectPixels
(resolution)def
paintRectPoints
()def
setBottomMargin
(bottomMargin)def
setLeftMargin
(leftMargin)def
setMargins
(margins)def
setMinimumMargins
(minMargins)def
setMode
(mode)def
setOrientation
(orientation)def
setPageSize
(pageSize[, minMargins=QMarginsF(0, 0, 0, 0)])def
setRightMargin
(rightMargin)def
setTopMargin
(topMargin)def
setUnits
(units)def
swap
(other)def
units
()
Detailed Description¶
The
QPageLayout
class defines the layout of a page in a paged document, with the page size, orientation and margins able to be set and the full page and paintable page rectangles defined by those attributes able to be queried in a variety of units.The page size is defined by the
QPageSize
class which can be queried for page size attributes. Note that theQPageSize
itself is always defined in a Portrait orientation.The minimum margins can be defined for the layout but normally default to 0. When used in conjunction with Qt’s printing support the minimum margins will reflect the minimum printable area defined by the printer.
In the default
StandardMode
the current margins and minimum margins are always taken into account. The paintable rectangle is the full page rectangle less the current margins, and the current margins can only be set to values between the minimum margins and the maximum margins allowed by the full page size.In
FullPageMode
the current margins and minimum margins are not taken into account. The paintable rectangle is the full page rectangle, and the current margins can be set to any values regardless of the minimum margins and page size.See also
- class PySide2.QtGui.QPageLayout¶
PySide2.QtGui.QPageLayout(other)
PySide2.QtGui.QPageLayout(pageSize, orientation, margins[, units=Point[, minMargins=QMarginsF(0, 0, 0, 0)]])
- param minMargins:
- param other:
- param orientation:
- param units:
- param pageSize:
- param margins:
Creates an invalid
QPageLayout
.Copy constructor, copies
other
to this.Creates a
QPageLayout
with the givenpageSize
,orientation
andmargins
in the givenunits
.Optionally define the minimum allowed margins
minMargins
, e.g. the minimum margins able to be printed by a physical print device.The constructed
QPageLayout
will be inStandardMode
.The
margins
given will be clamped to the minimum margins and the maximum margins allowed by the page size.
- PySide2.QtGui.QPageLayout.Unit¶
This enum type is used to specify the measurement unit for page layout and margins.
Constant
Description
QPageLayout.Millimeter
QPageLayout.Point
1/72th of an inch
QPageLayout.Inch
QPageLayout.Pica
1/72th of a foot, 1/6th of an inch, 12 Points
QPageLayout.Didot
1/72th of a French inch, 0.375 mm
QPageLayout.Cicero
1/6th of a French inch, 12 Didot, 4.5mm
- PySide2.QtGui.QPageLayout.Orientation¶
This enum type defines the page orientation
Constant
Description
QPageLayout.Portrait
The page size is used in its default orientation
QPageLayout.Landscape
The page size is rotated through 90 degrees
Note that some standard page sizes are defined with a width larger than their height, hence the orientation is defined relative to the standard page size and not using the relative page dimensions.
- PySide2.QtGui.QPageLayout.Mode¶
Defines the page layout mode
Constant
Description
QPageLayout.StandardMode
Paint Rect includes margins, margins must fall between the minimum and maximum.
QPageLayout.FullPageMode
Paint Rect excludes margins, margins can be any value and must be managed manually.
- PySide2.QtGui.QPageLayout.fullRect()¶
- Return type:
Returns the full page rectangle in the current layout units.
The page rectangle takes into account the page size and page orientation, but not the page margins.
See also
- PySide2.QtGui.QPageLayout.fullRect(units)
- Parameters:
units –
Unit
- Return type:
Returns the full page rectangle in the required
units
.The page rectangle takes into account the page size and page orientation, but not the page margins.
See also
- PySide2.QtGui.QPageLayout.fullRectPixels(resolution)¶
- Parameters:
resolution – int
- Return type:
Returns the full page rectangle in device pixels for the given
resolution
.The page rectangle takes into account the page size and page orientation, but not the page margins.
See also
- PySide2.QtGui.QPageLayout.fullRectPoints()¶
- Return type:
Returns the full page rectangle in Postscript Points (1/72 of an inch).
The page rectangle takes into account the page size and page orientation, but not the page margins.
See also
- PySide2.QtGui.QPageLayout.isEquivalentTo(other)¶
- Parameters:
other –
PySide2.QtGui.QPageLayout
- Return type:
bool
Returns
true
if this page layout is equivalent to theother
page layout, i.e. if the page has the same size, margins and orientation.
- PySide2.QtGui.QPageLayout.isValid()¶
- Return type:
bool
Returns
true
if this page layout is valid.
- PySide2.QtGui.QPageLayout.margins()¶
- Return type:
Returns the margins of the page layout using the currently set units.
See also
- PySide2.QtGui.QPageLayout.margins(units)
- Parameters:
units –
Unit
- Return type:
Returns the margins of the page layout using the requested
units
.See also
- PySide2.QtGui.QPageLayout.marginsPixels(resolution)¶
- Parameters:
resolution – int
- Return type:
Returns the margins of the page layout in device pixels for the given
resolution
.See also
- PySide2.QtGui.QPageLayout.marginsPoints()¶
- Return type:
Returns the margins of the page layout in Postscript Points (1/72 of an inch).
See also
- PySide2.QtGui.QPageLayout.maximumMargins()¶
- Return type:
Returns the maximum margins that would be applied if the page layout was in
StandardMode
.The maximum margins allowed are calculated as the full size of the page minus the minimum margins set. For example, if the page width is 100 points and the minimum right margin is 10 points, then the maximum left margin will be 90 points.
See also
- PySide2.QtGui.QPageLayout.minimumMargins()¶
- Return type:
Returns the minimum margins of the page layout.
See also
- PySide2.QtGui.QPageLayout.mode()¶
- Return type:
Returns the page layout mode.
See also
- PySide2.QtGui.QPageLayout.__ne__(rhs)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPageLayout.__eq__(rhs)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPageLayout.orientation()¶
- Return type:
Returns the page orientation of the page layout.
See also
- PySide2.QtGui.QPageLayout.pageSize()¶
- Return type:
Returns the page size of the page layout.
Note that the
QPageSize
is always defined in a Portrait orientation. To obtain a size that takes the set orientation into account you must usefullRect()
.See also
- PySide2.QtGui.QPageLayout.paintRect()¶
- Return type:
Returns the page rectangle in the current layout units.
The paintable rectangle takes into account the page size, orientation and margins.
If the
FullPageMode
mode is set then thefullRect()
is returned and the margins must be manually managed.
- PySide2.QtGui.QPageLayout.paintRect(units)
- Parameters:
units –
Unit
- Return type:
Returns the page rectangle in the required
units
.The paintable rectangle takes into account the page size, orientation and margins.
If the
FullPageMode
mode is set then thefullRect()
is returned and the margins must be manually managed.
- PySide2.QtGui.QPageLayout.paintRectPixels(resolution)¶
- Parameters:
resolution – int
- Return type:
Returns the paintable rectangle in rounded device pixels for the given
resolution
.The paintable rectangle takes into account the page size, orientation and margins.
If the
FullPageMode
mode is set then thefullRect()
is returned and the margins must be manually managed.
- PySide2.QtGui.QPageLayout.paintRectPoints()¶
- Return type:
Returns the paintable rectangle in rounded Postscript Points (1/72 of an inch).
The paintable rectangle takes into account the page size, orientation and margins.
If the
FullPageMode
mode is set then thefullRect()
is returned and the margins must be manually managed.
- PySide2.QtGui.QPageLayout.setBottomMargin(bottomMargin)¶
- Parameters:
bottomMargin – float
- Return type:
bool
Sets the bottom page margin of the page layout to
bottomMargin
. Returns true if the margin was successfully set.The units used are those currently defined for the layout. To use different units call
setUnits()
first.If in the default
StandardMode
then the new margin must fall between the minimum margin set and the maximum margin allowed by the page size, otherwise the margin will not be set.If in
FullPageMode
then any margin values will be accepted.See also
- PySide2.QtGui.QPageLayout.setLeftMargin(leftMargin)¶
- Parameters:
leftMargin – float
- Return type:
bool
Sets the left page margin of the page layout to
leftMargin
. Returns true if the margin was successfully set.The units used are those currently defined for the layout. To use different units call
setUnits()
first.If in the default
StandardMode
then the new margin must fall between the minimum margin set and the maximum margin allowed by the page size, otherwise the margin will not be set.If in
FullPageMode
then any margin values will be accepted.See also
- PySide2.QtGui.QPageLayout.setMargins(margins)¶
- Parameters:
margins –
PySide2.QtCore.QMarginsF
- Return type:
bool
Sets the page margins of the page layout to
margins
Returns true if the margins were successfully set.The units used are those currently defined for the layout. To use different units then call
setUnits()
first.If in the default
StandardMode
then all the new margins must fall between the minimum margins set and the maximum margins allowed by the page size, otherwise the margins will not be set.If in
FullPageMode
then any margin values will be accepted.
- PySide2.QtGui.QPageLayout.setMinimumMargins(minMargins)¶
- Parameters:
minMargins –
PySide2.QtCore.QMarginsF
Sets the minimum page margins of the page layout to
minMargins
.It is not recommended to override the default values set for a page size as this may be the minimum printable area for a physical print device.
If the
StandardMode
mode is set then the existing margins will be clamped to the newminMargins
and the maximum allowed by the page size. If theFullPageMode
is set then the existing margins will be unchanged.See also
- PySide2.QtGui.QPageLayout.setMode(mode)¶
- Parameters:
mode –
Mode
Sets a page layout mode to
mode
.See also
- PySide2.QtGui.QPageLayout.setOrientation(orientation)¶
- Parameters:
orientation –
Orientation
Sets the page orientation of the page layout to
orientation
.Changing the orientation does not affect the current margins or the minimum margins.
See also
- PySide2.QtGui.QPageLayout.setPageSize(pageSize[, minMargins=QMarginsF(0, 0, 0, 0)])¶
- Parameters:
pageSize –
PySide2.QtGui.QPageSize
minMargins –
PySide2.QtCore.QMarginsF
Sets the page size of the page layout to
pageSize
.Optionally define the minimum allowed margins
minMargins
, e.g. the minimum margins able to be printed by a physical print device, otherwise the minimum margins will default to 0.If
StandardMode
is set then the existing margins will be clamped to the new minimum margins and the maximum margins allowed by the page size. IfFullPageMode
is set then the existing margins will be unchanged.See also
- PySide2.QtGui.QPageLayout.setRightMargin(rightMargin)¶
- Parameters:
rightMargin – float
- Return type:
bool
Sets the right page margin of the page layout to
rightMargin
. Returns true if the margin was successfully set.The units used are those currently defined for the layout. To use different units call
setUnits()
first.If in the default
StandardMode
then the new margin must fall between the minimum margin set and the maximum margin allowed by the page size, otherwise the margin will not be set.If in
FullPageMode
then any margin values will be accepted.See also
- PySide2.QtGui.QPageLayout.setTopMargin(topMargin)¶
- Parameters:
topMargin – float
- Return type:
bool
Sets the top page margin of the page layout to
topMargin
. Returns true if the margin was successfully set.The units used are those currently defined for the layout. To use different units call
setUnits()
first.If in the default
StandardMode
then the new margin must fall between the minimum margin set and the maximum margin allowed by the page size, otherwise the margin will not be set.If in
FullPageMode
then any margin values will be accepted.See also
- PySide2.QtGui.QPageLayout.setUnits(units)¶
- Parameters:
units –
Unit
Sets the
units
used to define the page layout.See also
- PySide2.QtGui.QPageLayout.swap(other)¶
- Parameters:
other –
PySide2.QtGui.QPageLayout
Swaps this page layout with
other
. This function is very fast and never fails.
- PySide2.QtGui.QPageLayout.units()¶
- Return type:
Returns the units the page layout is currently defined in.
See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.