QLayout¶
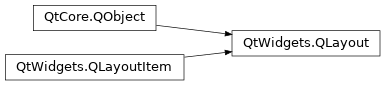
Inherited by: QBoxLayout, QFormLayout, QGridLayout, QHBoxLayout, QStackedLayout, QVBoxLayout
Synopsis¶
Functions¶
def
activate
()def
addChildLayout
(l)def
addChildWidget
(w)def
addWidget
(w)def
adoptLayout
(layout)def
alignmentRect
(arg__1)def
contentsMargins
()def
contentsRect
()def
getContentsMargins
()def
indexOf
(arg__1)def
isEnabled
()def
margin
()def
menuBar
()def
parentWidget
()def
removeItem
(arg__1)def
removeWidget
(w)def
replaceWidget
(from, to[, options=Qt.FindChildrenRecursively])def
setAlignment
(l, alignment)def
setAlignment
(w, alignment)def
setContentsMargins
(left, top, right, bottom)def
setContentsMargins
(margins)def
setEnabled
(arg__1)def
setMargin
(arg__1)def
setMenuBar
(w)def
setSizeConstraint
(arg__1)def
setSpacing
(arg__1)def
sizeConstraint
()def
spacing
()def
totalHeightForWidth
(w)def
totalMaximumSize
()def
totalMinimumSize
()def
totalSizeHint
()def
update
()def
widgetEvent
(arg__1)
Virtual functions¶
Static functions¶
def
closestAcceptableSize
(w, s)
Detailed Description¶
This is an abstract base class inherited by the concrete classes
QBoxLayout
,QGridLayout
,QFormLayout
, andQStackedLayout
.For users of
QLayout
subclasses or ofQMainWindow
there is seldom any need to use the basic functions provided byQLayout
, such assetSizeConstraint()
orsetMenuBar()
. See Layout Management for more information.To make your own layout manager, implement the functions
addItem()
,sizeHint()
,setGeometry()
,itemAt()
andtakeAt()
. You should also implementminimumSize()
to ensure your layout isn’t resized to zero size if there is too little space. To support children whose heights depend on their widths, implementhasHeightForWidth()
andheightForWidth()
. See the Border Layout and Flow Layout examples for more information about implementing custom layout managers.Geometry management stops when the layout manager is deleted.
- class PySide2.QtWidgets.QLayout¶
PySide2.QtWidgets.QLayout(parent)
- param parent:
Constructs a new child
QLayout
.This layout has to be inserted into another layout before geometry management will work.
Constructs a new top-level
QLayout
, with parentparent
.parent
may not beNone
.The layout is set directly as the top-level layout for
parent
. There can be only one top-level layout for a widget. It is returned bylayout()
.
- PySide2.QtWidgets.QLayout.SizeConstraint¶
The possible values are:
Constant
Description
QLayout.SetDefaultConstraint
The main widget’s minimum size is set to
minimumSize()
, unless the widget already has a minimum size.QLayout.SetFixedSize
The main widget’s size is set to
sizeHint()
; it cannot be resized at all.QLayout.SetMinimumSize
The main widget’s minimum size is set to
minimumSize()
; it cannot be smaller.QLayout.SetMaximumSize
The main widget’s maximum size is set to
maximumSize()
; it cannot be larger.QLayout.SetMinAndMaxSize
The main widget’s minimum size is set to
minimumSize()
and its maximum size is set tomaximumSize()
.QLayout.SetNoConstraint
The widget is not constrained.
See also
- PySide2.QtWidgets.QLayout.activate()¶
- Return type:
bool
Redoes the layout for
parentWidget()
if necessary.You should generally not need to call this because it is automatically called at the most appropriate times. It returns true if the layout was redone.
See also
- PySide2.QtWidgets.QLayout.addChildLayout(l)¶
- Parameters:
This function is called from
addLayout()
orinsertLayout()
functions in subclasses to add layoutl
as a sub-layout.The only scenario in which you need to call it directly is if you implement a custom layout that supports nested layouts.
See also
- PySide2.QtWidgets.QLayout.addChildWidget(w)¶
- Parameters:
This function is called from
addWidget()
functions in subclasses to addw
as a managed widget of a layout.If
w
is already managed by a layout, this function will give a warning and removew
from that layout. This function must therefore be called before addingw
to the layout’s data structure.
- PySide2.QtWidgets.QLayout.addItem(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtWidgets.QLayoutItem
Implemented in subclasses to add an
item
. How it is added is specific to each subclass.This function is not usually called in application code. To add a widget to a layout, use the
addWidget()
function; to add a child layout, use the addLayout() function provided by the relevantQLayout
subclass.Note
The ownership of
item
is transferred to the layout, and it’s the layout’s responsibility to delete it.See also
- PySide2.QtWidgets.QLayout.addWidget(w)¶
- Parameters:
Adds widget
w
to this layout in a manner specific to the layout. This function usesaddItem()
.
- PySide2.QtWidgets.QLayout.adoptLayout(layout)¶
- Parameters:
layout –
PySide2.QtWidgets.QLayout
- Return type:
bool
- PySide2.QtWidgets.QLayout.alignmentRect(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QRect
- Return type:
Returns the rectangle that should be covered when the geometry of this layout is set to
r
, provided that this layout supportssetAlignment()
.The result is derived from
sizeHint()
and expanding(). It is never larger thanr
.
- static PySide2.QtWidgets.QLayout.closestAcceptableSize(w, s)¶
- Parameters:
- Return type:
Returns a size that satisfies all size constraints on
widget
, includingheightForWidth()
and that is as close as possible tosize
.
- PySide2.QtWidgets.QLayout.contentsMargins()¶
- Return type:
- PySide2.QtWidgets.QLayout.contentsRect()¶
- Return type:
Returns the layout’s
geometry()
rectangle, but taking into account the contents margins.See also
- PySide2.QtWidgets.QLayout.count()¶
- Return type:
int
Must be implemented in subclasses to return the number of items in the layout.
See also
- PySide2.QtWidgets.QLayout.getContentsMargins()¶
For each of
left
,top
,right
andbottom
that is notNone
, stores the size of the margin named in the location the pointer refers to.By default,
QLayout
uses the values provided by the style. On most platforms, the margin is 11 pixels in all directions.See also
setContentsMargins()
pixelMetric()
PM_LayoutLeftMargin
PM_LayoutTopMargin
PM_LayoutRightMargin
PM_LayoutBottomMargin
- PySide2.QtWidgets.QLayout.indexOf(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtWidgets.QLayoutItem
- Return type:
int
Searches for layout item
layoutItem
in this layout (not including child layouts).Returns the index of
layoutItem
, or -1 iflayoutItem
is not found.
- PySide2.QtWidgets.QLayout.indexOf(arg__1)
- Parameters:
arg__1 –
PySide2.QtWidgets.QWidget
- Return type:
int
Searches for widget
widget
in this layout (not including child layouts).Returns the index of
widget
, or -1 ifwidget
is not found.The default implementation iterates over all items using
itemAt()
- PySide2.QtWidgets.QLayout.isEnabled()¶
- Return type:
bool
Returns
true
if the layout is enabled; otherwise returnsfalse
.See also
- PySide2.QtWidgets.QLayout.itemAt(index)¶
- Parameters:
index – int
- Return type:
Must be implemented in subclasses to return the layout item at
index
. If there is no such item, the function must return 0. Items are numbered consecutively from 0. If an item is deleted, other items will be renumbered.This function can be used to iterate over a layout. The following code will draw a rectangle for each layout item in the layout structure of the widget.
def paintLayout(self, painter, item): layout = item.layout() if layout: for layout_item in layout: self.paintLayout(painter, layout_item) painter.drawRect(item.geometry()) def paintEvent(self, event): painter = QPainter(self) if self.layout(): self.paintLayout(painter, self.layout())
- PySide2.QtWidgets.QLayout.margin()¶
- Return type:
int
This property holds the width of the outside border of the layout.
Use
setContentsMargins()
andgetContentsMargins()
instead.See also
- Return type:
Returns the menu bar set for this layout, or
None
if no menu bar is set.See also
- PySide2.QtWidgets.QLayout.parentWidget()¶
- Return type:
Returns the parent widget of this layout, or
None
if this layout is not installed on any widget.If the layout is a sub-layout, this function returns the parent widget of the parent layout.
See also
parent()
- PySide2.QtWidgets.QLayout.removeItem(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtWidgets.QLayoutItem
Removes the layout item
item
from the layout. It is the caller’s responsibility to delete the item.Notice that
item
can be a layout (sinceQLayout
inheritsQLayoutItem
).See also
- PySide2.QtWidgets.QLayout.removeWidget(w)¶
- Parameters:
Removes the widget
widget
from the layout. After this call, it is the caller’s responsibility to give the widget a reasonable geometry or to put the widget back into a layout or to explicitly hide it if necessary.Note
The ownership of
widget
remains the same as when it was added.See also
- PySide2.QtWidgets.QLayout.replaceWidget(from, to[, options=Qt.FindChildrenRecursively])¶
- Parameters:
from –
PySide2.QtWidgets.QWidget
options –
FindChildOptions
- Return type:
Searches for widget
from
and replaces it with widgetto
if found. Returns the layout item that contains the widgetfrom
on success. OtherwiseNone
is returned. Ifoptions
containsQt::FindChildrenRecursively
(the default), sub-layouts are searched for doing the replacement. Any other flag inoptions
is ignored.Notice that the returned item therefore might not belong to this layout, but to a sub-layout.
The returned layout item is no longer owned by the layout and should be either deleted or inserted to another layout. The widget
from
is no longer managed by the layout and may need to be deleted or hidden. The parent of widgetfrom
is left unchanged.This function works for the built-in Qt layouts, but might not work for custom layouts.
See also
- PySide2.QtWidgets.QLayout.setAlignment(l, alignment)¶
- Parameters:
alignment –
Alignment
- Return type:
bool
This is an overloaded function.
Sets the alignment for the layout
l
toalignment
and returnstrue
ifl
is found in this layout (not including child layouts); otherwise returnsfalse
.
- PySide2.QtWidgets.QLayout.setAlignment(w, alignment)
- Parameters:
alignment –
Alignment
- Return type:
bool
Sets the alignment for widget
w
toalignment
and returns true ifw
is found in this layout (not including child layouts); otherwise returnsfalse
.
- PySide2.QtWidgets.QLayout.setContentsMargins(margins)¶
- Parameters:
margins –
PySide2.QtCore.QMargins
- PySide2.QtWidgets.QLayout.setContentsMargins(left, top, right, bottom)
- Parameters:
left – int
top – int
right – int
bottom – int
Sets the
left
,top
,right
, andbottom
margins to use around the layout.By default,
QLayout
uses the values provided by the style. On most platforms, the margin is 11 pixels in all directions.See also
contentsMargins()
getContentsMargins()
pixelMetric()
PM_LayoutLeftMargin
PM_LayoutTopMargin
PM_LayoutRightMargin
PM_LayoutBottomMargin
- PySide2.QtWidgets.QLayout.setEnabled(arg__1)¶
- Parameters:
arg__1 – bool
Enables this layout if
enable
is true, otherwise disables it.An enabled layout adjusts dynamically to changes; a disabled layout acts as if it did not exist.
By default all layouts are enabled.
See also
- PySide2.QtWidgets.QLayout.setMargin(arg__1)¶
- Parameters:
arg__1 – int
This property holds the width of the outside border of the layout.
Use
setContentsMargins()
andgetContentsMargins()
instead.See also
- PySide2.QtWidgets.QLayout.setMenuBar(w)¶
- Parameters:
Tells the geometry manager to place the menu bar
widget
at the top ofparentWidget()
, outsidecontentsMargins()
. All child widgets are placed below the bottom edge of the menu bar.See also
- PySide2.QtWidgets.QLayout.setSizeConstraint(arg__1)¶
- Parameters:
arg__1 –
SizeConstraint
This property holds the resize mode of the layout.
The default mode is
SetDefaultConstraint
.
- PySide2.QtWidgets.QLayout.setSpacing(arg__1)¶
- Parameters:
arg__1 – int
This property holds the spacing between widgets inside the layout.
If no value is explicitly set, the layout’s spacing is inherited from the parent layout, or from the style settings for the parent widget.
For
QGridLayout
andQFormLayout
, it is possible to set different horizontal and vertical spacings usingsetHorizontalSpacing()
andsetVerticalSpacing()
. In that case, returns -1.
- PySide2.QtWidgets.QLayout.sizeConstraint()¶
- Return type:
This property holds the resize mode of the layout.
The default mode is
SetDefaultConstraint
.
- PySide2.QtWidgets.QLayout.spacing()¶
- Return type:
int
This property holds the spacing between widgets inside the layout.
If no value is explicitly set, the layout’s spacing is inherited from the parent layout, or from the style settings for the parent widget.
For
QGridLayout
andQFormLayout
, it is possible to set different horizontal and vertical spacings usingsetHorizontalSpacing()
andsetVerticalSpacing()
. In that case, returns -1.
- PySide2.QtWidgets.QLayout.takeAt(index)¶
- Parameters:
index – int
- Return type:
Must be implemented in subclasses to remove the layout item at
index
from the layout, and return the item. If there is no such item, the function must do nothing and return 0. Items are numbered consecutively from 0. If an item is removed, other items will be renumbered.The following code fragment shows a safe way to remove all items from a layout:
child = layout.takeAt(0) while child: ... del child
- PySide2.QtWidgets.QLayout.totalHeightForWidth(w)¶
- Parameters:
w – int
- Return type:
int
Also takes
contentsMargins
and menu bar into account.
- PySide2.QtWidgets.QLayout.totalMaximumSize()¶
- Return type:
Also takes
contentsMargins
and menu bar into account.
- PySide2.QtWidgets.QLayout.totalMinimumSize()¶
- Return type:
Also takes
contentsMargins
and menu bar into account.
- PySide2.QtWidgets.QLayout.totalSizeHint()¶
- Return type:
Also takes
contentsMargins
and menu bar into account.
- PySide2.QtWidgets.QLayout.update()¶
Updates the layout for
parentWidget()
.You should generally not need to call this because it is automatically called at the most appropriate times.
See also
activate()
invalidate()
- PySide2.QtWidgets.QLayout.widgetEvent(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QEvent
Performs child widget layout when the parent widget is resized. Also handles removal of widgets.
e
is the event
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.