QPoint¶
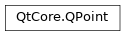
Synopsis¶
Functions¶
def
__add__
()def
__add__
(, p2)def
__div__
(, c)def
__eq__
(, p2)def
__iadd__
(p)def
__idiv__
(divisor)def
__imul__
(factor)def
__imul__
(factor)def
__imul__
(factor)def
__isub__
(p)def
__mul__
(, factor)def
__mul__
(, factor)def
__mul__
(, factor)def
__mul__
(, m)def
__mul__
(, m)def
__mul__
(, matrix)def
__mul__
(factor)def
__mul__
(factor)def
__mul__
(factor)def
__mul__
(matrix)def
__ne__
(, p2)def
__reduce__
()def
__repr__
()def
__sub__
()def
__sub__
(, p2)def
isNull
()def
manhattanLength
()def
setX
(x)def
setY
(y)def
toTuple
()def
transposed
()def
x
()def
y
()
Static functions¶
def
dotProduct
(p1, p2)
Detailed Description¶
A point is specified by a x coordinate and an y coordinate which can be accessed using the
x()
andy()
functions. TheisNull()
function returnstrue
if both x and y are set to 0. The coordinates can be set (or altered) using thesetX()
andsetY()
functions, or alternatively therx()
andry()
functions which return references to the coordinates (allowing direct manipulation).Given a point p , the following statements are all equivalent:
p = QPoint() p.setX(p.x() + 1) p += QPoint(1, 0)A
QPoint
object can also be used as a vector: Addition and subtraction are defined as for vectors (each component is added separately). AQPoint
object can also be divided or multiplied by anint
or aqreal
.In addition, the
QPoint
class provides themanhattanLength()
function which gives an inexpensive approximation of the length of theQPoint
object interpreted as a vector. Finally,QPoint
objects can be streamed as well as compared.See also
QPointF
QPolygon
- class PySide2.QtCore.QPoint¶
PySide2.QtCore.QPoint(QPoint)
PySide2.QtCore.QPoint(xpos, ypos)
- param QPoint:
- param ypos:
int
- param xpos:
int
Constructs a null point, i.e. with coordinates (0, 0)
See also
Constructs a point with the given coordinates (
xpos
,ypos
).
- PySide2.QtCore.QPoint.__reduce__()¶
- Return type:
object
- PySide2.QtCore.QPoint.__repr__()¶
- Return type:
object
- static PySide2.QtCore.QPoint.dotProduct(p1, p2)¶
- Parameters:
- Return type:
int
QPoint p( 3, 7); QPoint q(-1, 4); int lengthSquared = QPoint::dotProduct(p, q); // lengthSquared becomes 25
Returns the dot product of
p1
andp2
.
- PySide2.QtCore.QPoint.isNull()¶
- Return type:
bool
Returns
true
if both the x and y coordinates are set to 0, otherwise returnsfalse
.
- PySide2.QtCore.QPoint.manhattanLength()¶
- Return type:
int
Returns the sum of the absolute values of
x()
andy()
, traditionally known as the “Manhattan length” of the vector from the origin to the point. For example:class MyWidget(QWidget): self.oldPosition = QPointer() # event : QMouseEvent def mouseMoveEvent(QMouseEvent event): point = event.pos() - self.oldPosition if (point.manhattanLength() > 3): # the mouse has moved more than 3 pixels since the oldPosition pass
This is a useful, and quick to calculate, approximation to the true length:
trueLength = sqrt(pow(x(), 2) + pow(y(), 2))
The tradition of “Manhattan length” arises because such distances apply to travelers who can only travel on a rectangular grid, like the streets of Manhattan.
- PySide2.QtCore.QPoint.__ne__(p2)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QPoint.__mul__(matrix)¶
- Parameters:
matrix –
PySide2.QtGui.QMatrix4x4
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor – int
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor – int
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor – float
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor – float
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor –
double
- Return type:
- PySide2.QtCore.QPoint.__mul__(factor)
- Parameters:
factor –
double
- Return type:
- PySide2.QtCore.QPoint.__mul__(m)
- Parameters:
- Return type:
- PySide2.QtCore.QPoint.__mul__(matrix)
- Parameters:
matrix –
PySide2.QtGui.QMatrix4x4
- Return type:
- PySide2.QtCore.QPoint.__mul__(m)
- Parameters:
- Return type:
- PySide2.QtCore.QPoint.__imul__(factor)¶
- Parameters:
factor –
double
- Return type:
Multiplies this point’s coordinates by the given
factor
, and returns a reference to this point. For example:p = QPoint(-1, 4) p *= 2.5 # p becomes (-3, 10)
Note that the result is rounded to the nearest integer as points are held as integers. Use
QPointF
for floating point accuracy.See also
operator/=()
- PySide2.QtCore.QPoint.__imul__(factor)
- Parameters:
factor – int
- Return type:
Multiplies this point’s coordinates by the given
factor
, and returns a reference to this point.See also
operator/=()
- PySide2.QtCore.QPoint.__imul__(factor)
- Parameters:
factor – float
- Return type:
Multiplies this point’s coordinates by the given
factor
, and returns a reference to this point.Note that the result is rounded to the nearest integer as points are held as integers. Use
QPointF
for floating point accuracy.See also
operator/=()
- PySide2.QtCore.QPoint.__add__()¶
- Return type:
- PySide2.QtCore.QPoint.__add__(p2)
- Parameters:
- Return type:
- PySide2.QtCore.QPoint.__iadd__(p)¶
- Parameters:
- Return type:
Adds the given
point
to this point and returns a reference to this point. For example:p = QPoint( 3, 7) q = QPoint(-1, 4) p += q # p becomes (2, 11)
See also
operator-=()
- PySide2.QtCore.QPoint.__sub__()¶
- Return type:
- PySide2.QtCore.QPoint.__sub__(p2)
- Parameters:
- Return type:
- PySide2.QtCore.QPoint.__isub__(p)¶
- Parameters:
- Return type:
Subtracts the given
point
from this point and returns a reference to this point. For example:p = QPoint( 3, 7) q = QPoint(-1, 4) p -= q # p becomes (4, 3)
See also
operator+=()
- PySide2.QtCore.QPoint.__div__(c)¶
- Parameters:
c – float
- Return type:
- PySide2.QtCore.QPoint.__idiv__(divisor)¶
- Parameters:
divisor – float
- Return type:
This is an overloaded function.
Divides both x and y by the given
divisor
, and returns a reference to this point. For example:p = QPoint(-3, 10) p /= 2.5 # p becomes (-1, 4)
Note that the result is rounded to the nearest integer as points are held as integers. Use
QPointF
for floating point accuracy.See also
operator*=()
- PySide2.QtCore.QPoint.__eq__(p2)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QPoint.setX(x)¶
- Parameters:
x – int
Sets the x coordinate of this point to the given
x
coordinate.
- PySide2.QtCore.QPoint.setY(y)¶
- Parameters:
y – int
Sets the y coordinate of this point to the given
y
coordinate.
- PySide2.QtCore.QPoint.toTuple()¶
- Return type:
object
- PySide2.QtCore.QPoint.transposed()¶
- Return type:
Returns a point with x and y coordinates exchanged:
QPoint{1, 2}.transposed() // {2, 1}
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.