QTextCodec¶
The
QTextCodec
class provides conversions between text encodings. More…
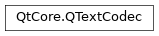
Synopsis¶
Functions¶
def
canEncode
(arg__1)def
canEncode
(arg__1)def
fromUnicode
(uc)def
makeDecoder
([flags=QTextCodec.DefaultConversion])def
makeEncoder
([flags=QTextCodec.DefaultConversion])def
toUnicode
(arg__1)def
toUnicode
(chars)def
toUnicode
(in, length[, state=None])
Virtual functions¶
def
aliases
()def
convertToUnicode
(in, length, state)def
mibEnum
()def
name
()
Static functions¶
def
availableCodecs
()def
availableMibs
()def
codecForHtml
(ba)def
codecForHtml
(ba, defaultCodec)def
codecForLocale
()def
codecForMib
(mib)def
codecForName
(name)def
codecForName
(name)def
codecForUtfText
(ba)def
codecForUtfText
(ba, defaultCodec)def
setCodecForLocale
(c)
Detailed Description¶
Qt uses Unicode to store, draw and manipulate strings. In many situations you may wish to deal with data that uses a different encoding. For example, most Japanese documents are still stored in Shift-JIS or ISO 2022-JP, while Russian users often have their documents in KOI8-R or Windows-1251.
Qt provides a set of
QTextCodec
classes to help with converting non-Unicode formats to and from Unicode. You can also create your own codec classes.The supported encodings are:
CP949
HP-ROMAN8
IBM 850
IBM 866
IBM 874
ISO 8859-1 to 10
ISO 8859-13 to 16
Iscii-Bng, Dev, Gjr, Knd, Mlm, Ori, Pnj, Tlg, and Tml
KOI8-R
KOI8-U
Macintosh
TIS-620
UTF-8
UTF-16
UTF-16BE
UTF-16LE
UTF-32
UTF-32BE
UTF-32LE
Windows-1250 to 1258
If Qt is compiled with ICU support enabled, most codecs supported by ICU will also be available to the application.
QTextCodec
s can be used as follows to convert some locally encoded string to Unicode. Suppose you have some string encoded in Russian KOI8-R encoding, and want to convert it to Unicode. The simple way to do it is like this:encodedString = QByteArray("...") codec = QTextCodec.codecForName("KOI8-R") string = codec.toUnicode(encodedString)After this,
string
holds the text converted to Unicode. Converting a string from Unicode to the local encoding is just as easy:string = u"..." codec = QTextCodec.codecForName("KOI8-R") encodedString = codec.fromUnicode(string)To read or write files in various encodings, use
QTextStream
and itssetCodec()
function. See the Codecs example for an application ofQTextCodec
to file I/O.Some care must be taken when trying to convert the data in chunks, for example, when receiving it over a network. In such cases it is possible that a multi-byte character will be split over two chunks. At best this might result in the loss of a character and at worst cause the entire conversion to fail.
The approach to use in these situations is to create a
QTextDecoder
object for the codec and use thisQTextDecoder
for the whole decoding process, as shown below:codec = QTextCodec.codecForName("Shift-JIS") decoder = codec.makeDecoder() string = u'' while new_data_available(): chunk = get_new_data() string += decoder.toUnicode(chunk)The
QTextDecoder
object maintains state between chunks and therefore works correctly even if a multi-byte character is split between chunks.
Creating Your Own Codec Class¶
Support for new text encodings can be added to Qt by creating
QTextCodec
subclasses.The pure virtual functions describe the encoder to the system and the coder is used as required in the different text file formats supported by
QTextStream
, and under X11, for the locale-specific character input and output.To add support for another encoding to Qt, make a subclass of
QTextCodec
and implement the functions listed in the table below.
Function
Description
Returns the official name for the encoding. If the encoding is listed in the IANA character-sets encoding file , the name should be the preferred MIME name for the encoding.
Returns a list of alternative names for the encoding.
QTextCodec
provides a default implementation that returns an empty list. For example, “ISO-8859-1” has “latin1”, “CP819”, “IBM819”, and “iso-ir-100” as aliases.Return the MIB enum for the encoding if it is listed in the IANA character-sets encoding file .
Converts an 8-bit character string to Unicode.
convertFromUnicode()
Converts a Unicode string to an 8-bit character string.
- class PySide2.QtCore.QTextCodec¶
Constructs a
QTextCodec
, and gives it the highest precedence. TheQTextCodec
should always be constructed on the heap (i.e. withnew
). Qt takes ownership and will delete it when the application terminates.
- PySide2.QtCore.QTextCodec.ConversionFlag¶
Constant
Description
QTextCodec.DefaultConversion
No flag is set.
QTextCodec.ConvertInvalidToNull
If this flag is set, each invalid input character is output as a null character.
QTextCodec.IgnoreHeader
Ignore any Unicode byte-order mark and don’t generate any.
- PySide2.QtCore.QTextCodec.aliases()¶
- Return type:
Subclasses can return a number of aliases for the codec in question.
Standard aliases for codecs can be found in the IANA character-sets encoding file .
- static PySide2.QtCore.QTextCodec.availableCodecs()¶
- Return type:
Returns the list of all available codecs, by name. Call
codecForName()
to obtain theQTextCodec
for the name.The list may contain many mentions of the same codec if the codec has aliases.
See also
- static PySide2.QtCore.QTextCodec.availableMibs()¶
- Return type:
Returns the list of MIBs for all available codecs. Call
codecForMib()
to obtain theQTextCodec
for the MIB.See also
- PySide2.QtCore.QTextCodec.canEncode(arg__1)¶
- Parameters:
arg__1 –
QChar
- Return type:
bool
Returns
true
if the Unicode characterch
can be fully encoded with this codec; otherwise returnsfalse
.
- PySide2.QtCore.QTextCodec.canEncode(arg__1)
- Parameters:
arg__1 – str
- Return type:
bool
- static PySide2.QtCore.QTextCodec.codecForHtml(ba)¶
- Parameters:
- Return type:
This is an overloaded function.
Tries to detect the encoding of the provided snippet of HTML in the given byte array,
ba
, by checking the BOM (Byte Order Mark) and the content-type meta header and returns aQTextCodec
instance that is capable of decoding the html to unicode. If the codec cannot be detected, this overload returns a Latin-1QTextCodec
.
- static PySide2.QtCore.QTextCodec.codecForHtml(ba, defaultCodec)
- Parameters:
defaultCodec –
PySide2.QtCore.QTextCodec
- Return type:
Tries to detect the encoding of the provided snippet of HTML in the given byte array,
ba
, by checking the BOM (Byte Order Mark) and the content-type meta header and returns aQTextCodec
instance that is capable of decoding the html to unicode. If the codec cannot be detected from the content provided,defaultCodec
is returned.See also
- static PySide2.QtCore.QTextCodec.codecForLocale()¶
- Return type:
Returns a pointer to the codec most suitable for this locale.
The codec will be retrieved from ICU where that backend is in use, otherwise it may be obtained from an OS-specific API. In the latter case, the codec’s name may be “System”.
See also
- static PySide2.QtCore.QTextCodec.codecForMib(mib)¶
- Parameters:
mib – int
- Return type:
Returns the
QTextCodec
which matches theMIBenum
mib
.
- static PySide2.QtCore.QTextCodec.codecForName(name)¶
- Parameters:
name –
PySide2.QtCore.QByteArray
- Return type:
- static PySide2.QtCore.QTextCodec.codecForName(name)
- Parameters:
name – str
- Return type:
Searches all installed
QTextCodec
objects and returns the one which best matchesname
; the match is case-insensitive. Returns 0 if no codec matching the namename
could be found.
- static PySide2.QtCore.QTextCodec.codecForUtfText(ba, defaultCodec)¶
- Parameters:
defaultCodec –
PySide2.QtCore.QTextCodec
- Return type:
Tries to detect the encoding of the provided snippet
ba
by using the BOM (Byte Order Mark) and returns aQTextCodec
instance that is capable of decoding the text to unicode. This function can detect one of the following codecs:UTF-32 Little Endian
UTF-32 Big Endian
UTF-16 Little Endian
UTF-16 Big Endian
UTF-8
If the codec cannot be detected from the content provided,
defaultCodec
is returned.See also
- static PySide2.QtCore.QTextCodec.codecForUtfText(ba)
- Parameters:
- Return type:
This is an overloaded function.
Tries to detect the encoding of the provided snippet
ba
by using the BOM (Byte Order Mark) and returns aQTextCodec
instance that is capable of decoding the text to unicode. This function can detect one of the following codecs:UTF-32 Little Endian
UTF-32 Big Endian
UTF-16 Little Endian
UTF-16 Big Endian
UTF-8
If the codec cannot be detected from the content provided, this overload returns a Latin-1
QTextCodec
.See also
- PySide2.QtCore.QTextCodec.convertToUnicode(in, length, state)¶
- Parameters:
in – str
length – int
- Return type:
str
QTextCodec
subclasses must reimplement this function.Converts the first
len
characters ofchars
from the encoding of the subclass to Unicode, and returns the result in aQString
.state
can beNone
, in which case the conversion is stateless and default conversion rules should be used. If state is not 0, the codec should save the state after the conversion instate
, and adjust theremainingChars
andinvalidChars
members of the struct.
- PySide2.QtCore.QTextCodec.fromUnicode(uc)¶
- Parameters:
uc – str
- Return type:
- PySide2.QtCore.QTextCodec.makeDecoder([flags=QTextCodec.DefaultConversion])¶
- Parameters:
flags –
ConversionFlags
- Return type:
Creates a
QTextDecoder
with a specifiedflags
to decode chunks ofchar *
data to create chunks of Unicode data.The caller is responsible for deleting the returned object.
- PySide2.QtCore.QTextCodec.makeEncoder([flags=QTextCodec.DefaultConversion])¶
- Parameters:
flags –
ConversionFlags
- Return type:
Creates a
QTextEncoder
with a specifiedflags
to encode chunks of Unicode data aschar *
data.The caller is responsible for deleting the returned object.
- PySide2.QtCore.QTextCodec.mibEnum()¶
- Return type:
int
Subclasses of
QTextCodec
must reimplement this function. It returns the MIBenum (see IANA character-sets encoding file for more information). It is important that eachQTextCodec
subclass returns the correct unique value for this function.
- PySide2.QtCore.QTextCodec.name()¶
- Return type:
QTextCodec
subclasses must reimplement this function. It returns the name of the encoding supported by the subclass.If the codec is registered as a character set in the IANA character-sets encoding file this method should return the preferred mime name for the codec if defined, otherwise its name.
- static PySide2.QtCore.QTextCodec.setCodecForLocale(c)¶
- Parameters:
Set the codec to
c
; this will be returned bycodecForLocale()
. Ifc
isNone
, the codec is reset to the default.This might be needed for some applications that want to use their own mechanism for setting the locale.
See also
- PySide2.QtCore.QTextCodec.toUnicode(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QByteArray
- Return type:
str
- PySide2.QtCore.QTextCodec.toUnicode(chars)
- Parameters:
chars – str
- Return type:
str
This is an overloaded function.
chars
contains the source characters.
- PySide2.QtCore.QTextCodec.toUnicode(in, length[, state=None])
- Parameters:
in – str
length – int
- Return type:
str
Converts the first
size
characters from theinput
from the encoding of this codec to Unicode, and returns the result in aQString
.The
state
of the convertor used is updated.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.