QImageWriter¶
The
QImageWriter
class provides a format independent interface for writing images to files or other devices. More…
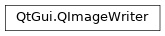
Synopsis¶
Functions¶
def
canWrite
()def
compression
()def
description
()def
device
()def
error
()def
errorString
()def
fileName
()def
format
()def
gamma
()def
optimizedWrite
()def
progressiveScanWrite
()def
quality
()def
setCompression
(compression)def
setDescription
(description)def
setDevice
(device)def
setFileName
(fileName)def
setFormat
(format)def
setGamma
(gamma)def
setOptimizedWrite
(optimize)def
setProgressiveScanWrite
(progressive)def
setQuality
(quality)def
setSubType
(type)def
setText
(key, text)def
setTransformation
(orientation)def
subType
()def
supportedSubTypes
()def
supportsOption
(option)def
transformation
()def
write
(image)
Static functions¶
def
imageFormatsForMimeType
(mimeType)def
supportedImageFormats
()def
supportedMimeTypes
()
Detailed Description¶
QImageWriter
supports setting format specific options, such as compression level and quality, prior to storing the image. If you do not need such options, you can usesave()
orsave()
instead.To store an image, you start by constructing a
QImageWriter
object. Pass either a file name or a device pointer, and the image format toQImageWriter
‘s constructor. You can then set several options, such as quality (by callingsetQuality()
).canWrite()
returnstrue
ifQImageWriter
can write the image (i.e., the image format is supported and the device is open for writing). Callwrite()
to write the image to the device.If any error occurs when writing the image,
write()
will return false. You can then callerror()
to find the type of error that occurred, orerrorString()
to get a human readable description of what went wrong.Call
supportedImageFormats()
for a list of formats thatQImageWriter
can write.QImageWriter
supports all built-in image formats, in addition to any image format plugins that support writing.Note
QImageWriter
assumes exclusive control over the file or device that is assigned. Any attempts to modify the assigned file or device during the lifetime of theQImageWriter
object will yield undefined results. If immediate access to a resource is desired, the use of a scope is the recommended method.For example:
QString imagePath(QStringLiteral("path/image.jpeg")); QImage image(64, 64, QImage::Format_RGB32); image.fill(Qt::red); { QImageWriter writer(imagePath); writer.write(image); } QFile::rename(imagePath, QStringLiteral("path/other_image.jpeg"));See also
QImageReader
QImageIOHandler
QImageIOPlugin
QColorSpace
- class PySide2.QtGui.QImageWriter¶
PySide2.QtGui.QImageWriter(device, format)
PySide2.QtGui.QImageWriter(fileName[, format=QByteArray()])
- param format:
- param device:
- param fileName:
str
Constructs an empty
QImageWriter
object. Before writing, you must callsetFormat()
to set an image format, thensetDevice()
orsetFileName()
.
- PySide2.QtGui.QImageWriter.ImageWriterError¶
This enum describes errors that can occur when writing images with
QImageWriter
.Constant
Description
QImageWriter.DeviceError
QImageWriter
encountered a device error when writing the image data. Consult your device for more details on what went wrong.QImageWriter.UnsupportedFormatError
Qt does not support the requested image format.
QImageWriter.InvalidImageError
An attempt was made to write an invalid
QImage
. An example of an invalid image would be a nullQImage
.QImageWriter.UnknownError
An unknown error occurred. If you get this value after calling
write()
, it is most likely caused by a bug inQImageWriter
.
- PySide2.QtGui.QImageWriter.canWrite()¶
- Return type:
bool
Returns
true
ifQImageWriter
can write the image; i.e., the image format is supported and the assigned device is open for reading.See also
- PySide2.QtGui.QImageWriter.compression()¶
- Return type:
int
Returns the compression of the image.
See also
- PySide2.QtGui.QImageWriter.description()¶
- Return type:
str
Note
This function is deprecated.
Use
text()
instead.Returns the description of the image.
See also
- PySide2.QtGui.QImageWriter.device()¶
- Return type:
Returns the device currently assigned to
QImageWriter
, orNone
if no device has been assigned.See also
- PySide2.QtGui.QImageWriter.error()¶
- Return type:
Returns the type of error that last occurred.
See also
ImageWriterError
errorString()
- PySide2.QtGui.QImageWriter.errorString()¶
- Return type:
str
Returns a human readable description of the last error that occurred.
See also
- PySide2.QtGui.QImageWriter.fileName()¶
- Return type:
str
If the currently assigned device is a
QFile
, or ifsetFileName()
has been called, this function returns the name of the fileQImageWriter
writes to. Otherwise (i.e., if no device has been assigned or the device is not aQFile
), an emptyQString
is returned.See also
- PySide2.QtGui.QImageWriter.format()¶
- Return type:
Returns the format
QImageWriter
uses for writing images.See also
- PySide2.QtGui.QImageWriter.gamma()¶
- Return type:
float
Note
This function is deprecated.
Use
colorSpace()
andgamma()
instead.Returns the gamma level of the image.
See also
- static PySide2.QtGui.QImageWriter.imageFormatsForMimeType(mimeType)¶
- Parameters:
mimeType –
PySide2.QtCore.QByteArray
- Return type:
Returns the list of image formats corresponding to
mimeType
.Note that the
QGuiApplication
instance must be created before this function is called.
- PySide2.QtGui.QImageWriter.optimizedWrite()¶
- Return type:
bool
Returns whether optimization has been turned on for writing the image.
See also
- PySide2.QtGui.QImageWriter.progressiveScanWrite()¶
- Return type:
bool
Returns whether the image should be written as a progressive image.
See also
- PySide2.QtGui.QImageWriter.quality()¶
- Return type:
int
Returns the quality setting of the image format.
See also
- PySide2.QtGui.QImageWriter.setCompression(compression)¶
- Parameters:
compression – int
This is an image format specific function that set the compression of an image. For image formats that do not support setting the compression, this value is ignored.
The value range of
compression
depends on the image format. For example, the “tiff” format supports two values, 0(no compression) and 1(LZW-compression).See also
- PySide2.QtGui.QImageWriter.setDescription(description)¶
- Parameters:
description – str
Note
This function is deprecated.
Use
setText()
instead.This is an image format specific function that sets the description of the image to
description
. For image formats that do not support setting the description, this value is ignored.The contents of
description
depends on the image format.See also
- PySide2.QtGui.QImageWriter.setDevice(device)¶
- Parameters:
device –
PySide2.QtCore.QIODevice
Sets
QImageWriter
‘s device todevice
. If a device has already been set, the old device is removed fromQImageWriter
and is otherwise left unchanged.If the device is not already open,
QImageWriter
will attempt to open the device inWriteOnly
mode by calling open(). Note that this does not work for certain devices, such asQProcess
,QTcpSocket
andQUdpSocket
, where more logic is required to open the device.See also
- PySide2.QtGui.QImageWriter.setFileName(fileName)¶
- Parameters:
fileName – str
Sets the file name of
QImageWriter
tofileName
. Internally,QImageWriter
will create aQFile
and open it inWriteOnly
mode, and use this file when writing images.See also
- PySide2.QtGui.QImageWriter.setFormat(format)¶
- Parameters:
format –
PySide2.QtCore.QByteArray
Sets the format
QImageWriter
will use when writing images, toformat
.format
is a case insensitive text string. Example:writer = QImageWriter() writer.setFormat("png") # same as writer.setFormat("PNG")
You can call
supportedImageFormats()
for the full list of formatsQImageWriter
supports.See also
- PySide2.QtGui.QImageWriter.setGamma(gamma)¶
- Parameters:
gamma – float
Note
This function is deprecated.
Use
QColorSpace
conversion on theQImage
instead.This is an image format specific function that sets the gamma level of the image to
gamma
. For image formats that do not support setting the gamma level, this value is ignored.The value range of
gamma
depends on the image format. For example, the “png” format supports a gamma range from 0.0 to 1.0.
- PySide2.QtGui.QImageWriter.setOptimizedWrite(optimize)¶
- Parameters:
optimize – bool
This is an image format-specific function which sets the
optimize
flags when writing images. For image formats that do not support setting anoptimize
flag, this value is ignored.The default is false.
See also
- PySide2.QtGui.QImageWriter.setProgressiveScanWrite(progressive)¶
- Parameters:
progressive – bool
This is an image format-specific function which turns on
progressive
scanning when writing images. For image formats that do not support setting aprogressive
scan flag, this value is ignored.The default is false.
See also
- PySide2.QtGui.QImageWriter.setQuality(quality)¶
- Parameters:
quality – int
Sets the quality setting of the image format to
quality
.Some image formats, in particular lossy ones, entail a tradeoff between a) visual quality of the resulting image, and b) encoding execution time and compression level. This function sets the level of that tradeoff for image formats that support it. For other formats, this value is ignored.
The value range of
quality
depends on the image format. For example, the “jpeg” format supports a quality range from 0 (low visual quality, high compression) to 100 (high visual quality, low compression).See also
- PySide2.QtGui.QImageWriter.setSubType(type)¶
- Parameters:
type –
PySide2.QtCore.QByteArray
This is an image format specific function that sets the subtype of the image to
type
. Subtype can be used by a handler to determine which format it should use while saving the image.For example, saving an image in DDS format with A8R8G8R8 subtype:
QImageWriter writer("some/image.dds"); if (writer.supportsOption(QImageIOHandler::SubType)) writer.setSubType("A8R8G8B8"); writer.write(image);
See also
- PySide2.QtGui.QImageWriter.setText(key, text)¶
- Parameters:
key – str
text – str
Sets the image text associated with the key
key
totext
. This is useful for storing copyright information or other information about the image. Example:image = QImage("some/image.jpeg") writer = QImageWriter("images/outimage.png", "png") writer.setText("Author", "John Smith") writer.write(image)
If you want to store a single block of data (e.g., a comment), you can pass an empty key, or use a generic key like “Description”.
The key and text will be embedded into the image data after calling
write()
.Support for this option is implemented through
Description
.
- PySide2.QtGui.QImageWriter.setTransformation(orientation)¶
- Parameters:
orientation – Transformations
Sets the image transformations metadata including orientation to
transform
.If transformation metadata is not supported by the image format, the transform is applied before writing.
See also
- PySide2.QtGui.QImageWriter.subType()¶
- Return type:
Returns the subtype of the image.
See also
- static PySide2.QtGui.QImageWriter.supportedImageFormats()¶
- Return type:
Returns the list of image formats supported by
QImageWriter
.By default, Qt can write the following formats:
Format
MIME type
Description
BMP
image/bmp
Windows Bitmap
JPG
image/jpeg
Joint Photographic Experts Group
PNG
image/png
Portable Network Graphics
PBM
image/x-portable-bitmap
Portable Bitmap
PGM
image/x-portable-graymap
Portable Graymap
PPM
image/x-portable-pixmap
Portable Pixmap
XBM
image/x-xbitmap
X11 Bitmap
XPM
image/x-xpixmap
X11 Pixmap
Reading and writing SVG files is supported through the Qt SVG module. The Qt Image Formats module provides support for additional image formats.
Note that the
QApplication
instance must be created before this function is called.See also
setFormat()
supportedImageFormats()
QImageIOPlugin
- static PySide2.QtGui.QImageWriter.supportedMimeTypes()¶
- Return type:
Returns the list of MIME types supported by
QImageWriter
.Note that the
QApplication
instance must be created before this function is called.
- PySide2.QtGui.QImageWriter.supportedSubTypes()¶
- Return type:
Returns the list of subtypes supported by an image.
- PySide2.QtGui.QImageWriter.supportsOption(option)¶
- Parameters:
option –
ImageOption
- Return type:
bool
Returns
true
if the writer supportsoption
; otherwise returns false.Different image formats support different options. Call this function to determine whether a certain option is supported by the current format. For example, the PNG format allows you to embed text into the image’s metadata (see text()).
writer = QImageWriter(fileName) if writer.supportsOption(QImageIOHandler.Description): writer.setText("Author", "John Smith")
Options can be tested after the writer has been associated with a format.
See also
- PySide2.QtGui.QImageWriter.transformation()¶
- Return type:
Returns the transformation and orientation the image has been set to written with.
See also
- PySide2.QtGui.QImageWriter.write(image)¶
- Parameters:
image –
PySide2.QtGui.QImage
- Return type:
bool
Writes the image
image
to the assigned device or file name. Returnstrue
on success; otherwise returnsfalse
. If the operation fails, you can callerror()
to find the type of error that occurred, orerrorString()
to get a human readable description of the error.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.