QPolygonF¶
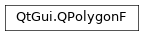
Synopsis¶
Functions¶
def
__add__
(l)def
__eq__
(v)def
__iadd__
(t)def
__iadd__
(t)def
__lshift__
(t)def
__mul__
(, m)def
__mul__
(, m)def
__ne__
(v)def
append
(l)def
append
(t)def
append
(t)def
at
(i)def
back
()def
boundingRect
()def
capacity
()def
clear
()def
constData
()def
constFirst
()def
constLast
()def
contains
(t)def
containsPoint
(pt, fillRule)def
count
()def
count
(t)def
data
()def
empty
()def
endsWith
(t)def
fill
(t[, size=-1])def
first
()def
front
()def
indexOf
(t[, from=0])def
insert
(i, n, t)def
insert
(i, t)def
insert
(i, t)def
intersected
(r)def
intersects
(r)def
isClosed
()def
isEmpty
()def
isSharedWith
(other)def
last
()def
lastIndexOf
(t[, from=-1])def
length
()def
mid
(pos[, len=-1])def
move
(from, to)def
operator[]
(i)def
pop_back
()def
pop_front
()def
prepend
(t)def
prepend
(t)def
push_back
(t)def
push_back
(t)def
push_front
(t)def
push_front
(t)def
remove
(i)def
remove
(i, n)def
removeAll
(t)def
removeAt
(i)def
removeFirst
()def
removeLast
()def
removeOne
(t)def
replace
(i, t)def
reserve
(size)def
resize
(size)def
setSharable
(sharable)def
shrink_to_fit
()def
size
()def
squeeze
()def
startsWith
(t)def
subtracted
(r)def
swap
(other)def
swapItemsAt
(i, j)def
takeAt
(i)def
takeFirst
()def
takeLast
()def
toList
()def
toPolygon
()def
translate
(dx, dy)def
translate
(offset)def
translated
(dx, dy)def
translated
(offset)def
united
(r)def
value
(i)def
value
(i, defaultValue)
Static functions¶
def
fromList
(list)
Detailed Description¶
A
QPolygonF
is aQVector
<QPointF
>. The easiest way to add points to aQPolygonF
is to use its streaming operator, as illustrated below:polygon = QPolygonF() polygon << QPointF(10.4, 20.5) << QPointF(20.2, 30.2)In addition to the functions provided by
QVector
,QPolygonF
provides theboundingRect()
andtranslate()
functions for geometry operations. Use themap()
function for more general transformations of QPolygonFs.
QPolygonF
also provides theisClosed()
function to determine whether a polygon’s start and end points are the same, and thetoPolygon()
function returning an integer precision copy of this polygon.The
QPolygonF
class is implicitly shared .See also
QVector
QPolygon
QLineF
- class PySide2.QtGui.QPolygonF¶
PySide2.QtGui.QPolygonF(v)
PySide2.QtGui.QPolygonF(a)
PySide2.QtGui.QPolygonF(a)
PySide2.QtGui.QPolygonF(r)
PySide2.QtGui.QPolygonF(v)
PySide2.QtGui.QPolygonF(size)
- param size:
int
- param a:
- param r:
- param v:
Constructs a polygon with no points.
See also
isEmpty()
Constructs a polygon of the given
size
. Creates an empty polygon ifsize
== 0.See also
isEmpty()
- PySide2.QtGui.QPolygonF.append(t)¶
- Parameters:
- PySide2.QtGui.QPolygonF.append(t)
- Parameters:
- PySide2.QtGui.QPolygonF.append(l)
- Parameters:
l –
- PySide2.QtGui.QPolygonF.at(i)¶
- Parameters:
i – int
- Return type:
- PySide2.QtGui.QPolygonF.back()¶
- Return type:
- PySide2.QtGui.QPolygonF.boundingRect()¶
- Return type:
Returns the bounding rectangle of the polygon, or
QRectF
(0,0,0,0) if the polygon is empty.See also
isEmpty()
- PySide2.QtGui.QPolygonF.capacity()¶
- Return type:
int
- PySide2.QtGui.QPolygonF.clear()¶
- PySide2.QtGui.QPolygonF.constData()¶
- Return type:
- PySide2.QtGui.QPolygonF.constFirst()¶
- Return type:
- PySide2.QtGui.QPolygonF.constLast()¶
- Return type:
- PySide2.QtGui.QPolygonF.contains(t)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPolygonF.containsPoint(pt, fillRule)¶
- Parameters:
fillRule –
FillRule
- Return type:
bool
Returns
true
if the givenpoint
is inside the polygon according to the specifiedfillRule
; otherwise returnsfalse
.
- PySide2.QtGui.QPolygonF.count()¶
- Return type:
int
- PySide2.QtGui.QPolygonF.count(t)
- Parameters:
- Return type:
int
- PySide2.QtGui.QPolygonF.data()¶
- Return type:
- PySide2.QtGui.QPolygonF.empty()¶
- Return type:
bool
- PySide2.QtGui.QPolygonF.endsWith(t)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPolygonF.fill(t[, size=-1])¶
- Parameters:
size – int
- Return type:
- PySide2.QtGui.QPolygonF.first()¶
- Return type:
- static PySide2.QtGui.QPolygonF.fromList(list)¶
- Parameters:
list –
- Return type:
- PySide2.QtGui.QPolygonF.front()¶
- Return type:
- PySide2.QtGui.QPolygonF.indexOf(t[, from=0])¶
- Parameters:
from – int
- Return type:
int
- PySide2.QtGui.QPolygonF.insert(i, t)¶
- Parameters:
i – int
- PySide2.QtGui.QPolygonF.insert(i, t)
- Parameters:
i – int
- PySide2.QtGui.QPolygonF.insert(i, n, t)
- Parameters:
i – int
n – int
- PySide2.QtGui.QPolygonF.intersected(r)¶
- Parameters:
- Return type:
Returns a polygon which is the intersection of this polygon and
r
.Set operations on polygons will treat the polygons as areas. Non-closed polygons will be treated as implicitly closed.
See also
- PySide2.QtGui.QPolygonF.intersects(r)¶
- Parameters:
- Return type:
bool
Returns
true
if the current polygon intersects at any point the given polygonp
. Also returnstrue
if the current polygon contains or is contained by any part ofp
.Set operations on polygons will treat the polygons as areas. Non-closed polygons will be treated as implicitly closed.
See also
- PySide2.QtGui.QPolygonF.isClosed()¶
- Return type:
bool
Returns
true
if the polygon is closed; otherwise returnsfalse
.A polygon is said to be closed if its start point and end point are equal.
See also
first()
last()
- PySide2.QtGui.QPolygonF.isEmpty()¶
- Return type:
bool
- Parameters:
other –
- Return type:
bool
- PySide2.QtGui.QPolygonF.last()¶
- Return type:
- PySide2.QtGui.QPolygonF.lastIndexOf(t[, from=-1])¶
- Parameters:
from – int
- Return type:
int
- PySide2.QtGui.QPolygonF.length()¶
- Return type:
int
- PySide2.QtGui.QPolygonF.mid(pos[, len=-1])¶
- Parameters:
pos – int
len – int
- Return type:
- PySide2.QtGui.QPolygonF.move(from, to)¶
- Parameters:
from – int
to – int
- PySide2.QtGui.QPolygonF.__ne__(v)¶
- Parameters:
v –
- Return type:
bool
- PySide2.QtGui.QPolygonF.__mul__(m)¶
- Parameters:
- Return type:
- PySide2.QtGui.QPolygonF.__mul__(m)
- Parameters:
- Return type:
- PySide2.QtGui.QPolygonF.__add__(l)¶
- Parameters:
l –
- Return type:
- PySide2.QtGui.QPolygonF.__iadd__(t)¶
- Parameters:
- Return type:
- PySide2.QtGui.QPolygonF.__iadd__(t)
- Parameters:
- Return type:
- PySide2.QtGui.QPolygonF.__lshift__(t)¶
- Parameters:
- Return type:
- PySide2.QtGui.QPolygonF.__eq__(v)¶
- Parameters:
v –
- Return type:
bool
- PySide2.QtGui.QPolygonF.operator[](i)
- Parameters:
i – int
- Return type:
- PySide2.QtGui.QPolygonF.pop_back()¶
- PySide2.QtGui.QPolygonF.pop_front()¶
- PySide2.QtGui.QPolygonF.prepend(t)¶
- Parameters:
- PySide2.QtGui.QPolygonF.prepend(t)
- Parameters:
- PySide2.QtGui.QPolygonF.push_back(t)¶
- Parameters:
- PySide2.QtGui.QPolygonF.push_back(t)
- Parameters:
- PySide2.QtGui.QPolygonF.push_front(t)¶
- Parameters:
- PySide2.QtGui.QPolygonF.push_front(t)
- Parameters:
- PySide2.QtGui.QPolygonF.remove(i)¶
- Parameters:
i – int
- PySide2.QtGui.QPolygonF.remove(i, n)
- Parameters:
i – int
n – int
- PySide2.QtGui.QPolygonF.removeAll(t)¶
- Parameters:
- Return type:
int
- PySide2.QtGui.QPolygonF.removeAt(i)¶
- Parameters:
i – int
- PySide2.QtGui.QPolygonF.removeFirst()¶
- PySide2.QtGui.QPolygonF.removeLast()¶
- PySide2.QtGui.QPolygonF.removeOne(t)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPolygonF.replace(i, t)¶
- Parameters:
i – int
- PySide2.QtGui.QPolygonF.reserve(size)¶
- Parameters:
size – int
- PySide2.QtGui.QPolygonF.resize(size)¶
- Parameters:
size – int
- PySide2.QtGui.QPolygonF.setSharable(sharable)¶
- Parameters:
sharable – bool
- PySide2.QtGui.QPolygonF.shrink_to_fit()¶
- PySide2.QtGui.QPolygonF.size()¶
- Return type:
int
- PySide2.QtGui.QPolygonF.squeeze()¶
- PySide2.QtGui.QPolygonF.startsWith(t)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QPolygonF.subtracted(r)¶
- Parameters:
- Return type:
Returns a polygon which is
r
subtracted from this polygon.Set operations on polygons will treat the polygons as areas. Non-closed polygons will be treated as implicitly closed.
- PySide2.QtGui.QPolygonF.swap(other)¶
- Parameters:
other –
PySide2.QtGui.QPolygonF
Swaps polygon
other
with this polygon. This operation is very fast and never fails.
- PySide2.QtGui.QPolygonF.swapItemsAt(i, j)¶
- Parameters:
i – int
j – int
- PySide2.QtGui.QPolygonF.takeAt(i)¶
- Parameters:
i – int
- Return type:
- PySide2.QtGui.QPolygonF.takeFirst()¶
- Return type:
- PySide2.QtGui.QPolygonF.takeLast()¶
- Return type:
- PySide2.QtGui.QPolygonF.toList()¶
- Return type:
- PySide2.QtGui.QPolygonF.toPolygon()¶
- Return type:
Creates and returns a
QPolygon
by converting eachQPointF
to aQPoint
.See also
toPoint()
- PySide2.QtGui.QPolygonF.translate(offset)¶
- Parameters:
offset –
PySide2.QtCore.QPointF
Translate all points in the polygon by the given
offset
.See also
- PySide2.QtGui.QPolygonF.translate(dx, dy)
- Parameters:
dx – float
dy – float
This is an overloaded function.
Translates all points in the polygon by (
dx
,dy
).See also
- PySide2.QtGui.QPolygonF.translated(offset)¶
- Parameters:
offset –
PySide2.QtCore.QPointF
- Return type:
Returns a copy of the polygon that is translated by the given
offset
.See also
- PySide2.QtGui.QPolygonF.translated(dx, dy)
- Parameters:
dx – float
dy – float
- Return type:
This is an overloaded function.
Returns a copy of the polygon that is translated by (
dx
,dy
).See also
- PySide2.QtGui.QPolygonF.united(r)¶
- Parameters:
- Return type:
Returns a polygon which is the union of this polygon and
r
.Set operations on polygons will treat the polygons as areas. Non-closed polygons will be treated as implicitly closed.
See also
- PySide2.QtGui.QPolygonF.value(i)¶
- Parameters:
i – int
- Return type:
- PySide2.QtGui.QPolygonF.value(i, defaultValue)
- Parameters:
i – int
defaultValue –
PySide2.QtCore.QPointF
- Return type:
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.