QQmlProperty¶
The
QQmlProperty
class abstracts accessing properties on objects created from QML. More…
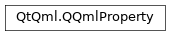
Synopsis¶
Functions¶
def
__eq__
(arg__1)def
connectNotifySignal
(dest, method)def
connectNotifySignal
(dest, slot)def
hasNotifySignal
()def
index
()def
isDesignable
()def
isProperty
()def
isResettable
()def
isSignalProperty
()def
isValid
()def
isWritable
()def
method
()def
name
()def
needsNotifySignal
()def
object
()def
property
()def
propertyType
()def
propertyTypeCategory
()def
propertyTypeName
()def
read
()def
reset
()def
type
()def
write
(arg__1)
Static functions¶
Detailed Description¶
As QML uses Qt’s meta-type system all of the existing
QMetaObject
classes can be used to introspect and interact with objects created by QML. However, some of the new features provided by QML - such as type safety and attached properties - are most easily used through theQQmlProperty
class that simplifies some of their natural complexity.Unlike
QMetaProperty
which represents a property on a class type,QQmlProperty
encapsulates a property on a specific object instance. To read a property’s value, programmers create aQQmlProperty
instance and call theread()
method. Likewise to write a property value thewrite()
method is used.For example, for the following QML code:
The Text object’s properties could be accessed using
QQmlProperty
, like this:#include <QQmlProperty> #include <QGraphicsObject> ... QQuickView view(QUrl::fromLocalFile("MyItem.qml")); QQmlProperty property(view.rootObject(), "font.pixelSize"); qWarning() << "Current pixel size:" << property.read().toInt(); property.write(24); qWarning() << "Pixel size should now be 24:" << property.read().toInt();
- class PySide2.QtQml.QQmlProperty¶
PySide2.QtQml.QQmlProperty(arg__1)
PySide2.QtQml.QQmlProperty(arg__1, arg__2)
PySide2.QtQml.QQmlProperty(arg__1, arg__2)
PySide2.QtQml.QQmlProperty(arg__1, arg__2)
PySide2.QtQml.QQmlProperty(arg__1, arg__2, arg__3)
PySide2.QtQml.QQmlProperty(arg__1, arg__2, arg__3)
PySide2.QtQml.QQmlProperty(arg__1)
- param arg__1:
- param arg__2:
- param arg__3:
Create an invalid
QQmlProperty
.Creates a
QQmlProperty
for the default property ofobj
. If there is no default property, an invalidQQmlProperty
will be created.Creates a
QQmlProperty
for the default property ofobj
using thecontext
ctxt
. If there is no default property, an invalidQQmlProperty
will be created.Creates a
QQmlProperty
for the default property ofobj
using the environment for instantiating QML components that is provided byengine
. If there is no default property, an invalidQQmlProperty
will be created.
- PySide2.QtQml.QQmlProperty.PropertyTypeCategory¶
This enum specifies a category of QML property.
Constant
Description
QQmlProperty.InvalidCategory
The property is invalid, or is a signal property.
QQmlProperty.List
The property is a
QQmlListProperty
list propertyQQmlProperty.Object
The property is a
QObject
derived type pointerQQmlProperty.Normal
The property is a normal value property.
- PySide2.QtQml.QQmlProperty.Type¶
This enum specifies a type of QML property.
Constant
Description
QQmlProperty.Invalid
The property is invalid.
QQmlProperty.Property
The property is a regular Qt property.
QQmlProperty.SignalProperty
The property is a signal property.
- PySide2.QtQml.QQmlProperty.connectNotifySignal(dest, slot)¶
- Parameters:
dest –
PySide2.QtCore.QObject
slot – str
- Return type:
bool
Connects the property’s change notifier signal to the specified
slot
of thedest
object and returns true. Returns false if this metaproperty does not represent a regular Qt property or if it has no change notifier signal, or if thedest
object does not have the specifiedslot
.Note
slot
should be passed using the SLOT() macro so it is correctly identified.
- PySide2.QtQml.QQmlProperty.connectNotifySignal(dest, method)
- Parameters:
dest –
PySide2.QtCore.QObject
method – int
- Return type:
bool
Connects the property’s change notifier signal to the specified
method
of thedest
object and returns true. Returns false if this metaproperty does not represent a regular Qt property or if it has no change notifier signal, or if thedest
object does not have the specifiedmethod
.
- PySide2.QtQml.QQmlProperty.hasNotifySignal()¶
- Return type:
bool
Returns true if the property has a change notifier signal, otherwise false.
- PySide2.QtQml.QQmlProperty.index()¶
- Return type:
int
Return the Qt metaobject index of the property.
- PySide2.QtQml.QQmlProperty.isDesignable()¶
- Return type:
bool
Returns true if the property is designable, otherwise false.
- PySide2.QtQml.QQmlProperty.isProperty()¶
- Return type:
bool
Returns true if this
QQmlProperty
represents a regular Qt property.
- PySide2.QtQml.QQmlProperty.isResettable()¶
- Return type:
bool
Returns true if the property is resettable, otherwise false.
- PySide2.QtQml.QQmlProperty.isSignalProperty()¶
- Return type:
bool
Returns true if this
QQmlProperty
represents a QML signal property.
- PySide2.QtQml.QQmlProperty.isValid()¶
- Return type:
bool
Returns true if the
QQmlProperty
refers to a valid property, otherwise false.
- PySide2.QtQml.QQmlProperty.isWritable()¶
- Return type:
bool
Returns true if the property is writable, otherwise false.
- PySide2.QtQml.QQmlProperty.method()¶
- Return type:
Return the
QMetaMethod
for this property if it is aSignalProperty
, otherwise returns an invalidQMetaMethod
.
- PySide2.QtQml.QQmlProperty.name()¶
- Return type:
str
Return the name of this QML property.
- PySide2.QtQml.QQmlProperty.needsNotifySignal()¶
- Return type:
bool
Returns true if the property needs a change notifier signal for bindings to remain upto date, false otherwise.
Some properties, such as attached properties or those whose value never changes, do not require a change notifier.
- PySide2.QtQml.QQmlProperty.object()¶
- Return type:
Returns the
QQmlProperty
‘sQObject
.
- PySide2.QtQml.QQmlProperty.__eq__(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtQml.QQmlProperty
- Return type:
bool
Returns true if
other
and thisQQmlProperty
represent the same property.
- PySide2.QtQml.QQmlProperty.property()¶
- Return type:
Returns the
Qt property
associated with this QML property.
- PySide2.QtQml.QQmlProperty.propertyType()¶
- Return type:
int
Returns the
QVariant
type of the property, orInvalid
if the property has noQVariant
type.
- PySide2.QtQml.QQmlProperty.propertyTypeCategory()¶
- Return type:
Returns the property category.
- PySide2.QtQml.QQmlProperty.propertyTypeName()¶
- Return type:
str
Returns the type name of the property, or 0 if the property has no type name.
- static PySide2.QtQml.QQmlProperty.read(arg__1, arg__2)¶
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
- Return type:
object
Return the
name
property value ofobject
. This method is equivalent to:QQmlProperty p(object, name); p.read();
- static PySide2.QtQml.QQmlProperty.read(arg__1, arg__2, arg__3)
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
arg__3 –
PySide2.QtQml.QQmlEngine
- Return type:
object
- static PySide2.QtQml.QQmlProperty.read(arg__1, arg__2, arg__3)
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
arg__3 –
PySide2.QtQml.QQmlContext
- Return type:
object
- PySide2.QtQml.QQmlProperty.read()
- Return type:
object
Returns the property value.
- PySide2.QtQml.QQmlProperty.reset()¶
- Return type:
bool
Resets the property and returns true if the property is resettable. If the property is not resettable, nothing happens and false is returned.
- static PySide2.QtQml.QQmlProperty.write(arg__1, arg__2, arg__3)¶
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
arg__3 – object
- Return type:
bool
Writes
value
to thename
property ofobject
. This method is equivalent to:QQmlProperty p(object, name); p.write(value);
Returns
true
on success,false
otherwise.
- static PySide2.QtQml.QQmlProperty.write(arg__1, arg__2, arg__3, arg__4)
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
arg__3 – object
arg__4 –
PySide2.QtQml.QQmlContext
- Return type:
bool
- static PySide2.QtQml.QQmlProperty.write(arg__1, arg__2, arg__3, arg__4)
- Parameters:
arg__1 –
PySide2.QtCore.QObject
arg__2 – str
arg__3 – object
arg__4 –
PySide2.QtQml.QQmlEngine
- Return type:
bool
- PySide2.QtQml.QQmlProperty.write(arg__1)
- Parameters:
arg__1 – object
- Return type:
bool
Sets the property value to
value
. Returnstrue
on success, orfalse
if the property can’t be set because thevalue
is the wrong type, for example.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.