QSqlError¶
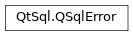
Synopsis¶
Functions¶
def
__eq__
(other)def
__ne__
(other)def
databaseText
()def
driverText
()def
isValid
()def
nativeErrorCode
()def
number
()def
setDatabaseText
(databaseText)def
setDriverText
(driverText)def
setNumber
(number)def
setType
(type)def
swap
(other)def
text
()def
type
()
Detailed Description¶
A
QSqlError
object can provide database-specific error data, including thedriverText()
anddatabaseText()
messages (or both concatenated together astext()
), and thenativeErrorCode()
andtype()
.See also
- class PySide2.QtSql.QSqlError(other)¶
PySide2.QtSql.QSqlError([driverText=””[, databaseText=””[, type=NoError[, errorCode=””]]]])
PySide2.QtSql.QSqlError(driverText, databaseText, type, number)
Note
This constructor is deprecated.
- Parameters:
type –
ErrorType
driverText – str
databaseText – str
other –
PySide2.QtSql.QSqlError
number – int
errorCode – str
- PySide2.QtSql.QSqlError.ErrorType¶
This enum type describes the context in which the error occurred, e.g., a connection error, a statement error, etc.
Constant
Description
QSqlError.NoError
No error occurred.
QSqlError.ConnectionError
Connection error.
QSqlError.StatementError
SQL statement syntax error.
QSqlError.TransactionError
Transaction failed error.
QSqlError.UnknownError
Unknown error.
- PySide2.QtSql.QSqlError.databaseText()¶
- Return type:
str
Returns the text of the error as reported by the database. This may contain database-specific descriptions; it may be empty.
See also
- PySide2.QtSql.QSqlError.driverText()¶
- Return type:
str
Returns the text of the error as reported by the driver. This may contain database-specific descriptions. It may also be empty.
See also
- PySide2.QtSql.QSqlError.isValid()¶
- Return type:
bool
Returns
true
if an error is set, otherwise false.Example:
model = QSqlQueryModel() model.setQuery("select * from myTable") if model.lastError().isValid(): print model.lastError()
See also
- PySide2.QtSql.QSqlError.nativeErrorCode()¶
- Return type:
str
Returns the database-specific error code, or an empty string if it cannot be determined.
- PySide2.QtSql.QSqlError.number()¶
- Return type:
int
Note
This function is deprecated.
Returns the database-specific error number, or -1 if it cannot be determined.
Returns 0 if the error code is not an integer.
Warning
Some databases use alphanumeric error codes, which makes unreliable if such a database is used.
Use
nativeErrorCode()
insteadSee also
- PySide2.QtSql.QSqlError.__ne__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlError
- Return type:
bool
Compare the
other
error’s values to this error and returnstrue
if it is not equal.
- PySide2.QtSql.QSqlError.__eq__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlError
- Return type:
bool
Compare the
other
error’s values to this error and returnstrue
, if it equal.
- PySide2.QtSql.QSqlError.setDatabaseText(databaseText)¶
- Parameters:
databaseText – str
Note
This function is deprecated.
Sets the database error text to the value of
databaseText
.Use
QSqlError
(constQString
&driverText
, constQString
&databaseText
,ErrorType
type, int number) insteadSee also
- PySide2.QtSql.QSqlError.setDriverText(driverText)¶
- Parameters:
driverText – str
Note
This function is deprecated.
Sets the driver error text to the value of
driverText
.Use
QSqlError
(constQString
&driverText
, constQString
&databaseText
,ErrorType
type, int number) insteadSee also
- PySide2.QtSql.QSqlError.setNumber(number)¶
- Parameters:
number – int
Note
This function is deprecated.
Sets the database-specific error number to
number
.Use
QSqlError
(constQString
&driverText
, constQString
&databaseText
,ErrorType
type, int number) insteadSee also
- PySide2.QtSql.QSqlError.setType(type)¶
- Parameters:
type –
ErrorType
Note
This function is deprecated.
Sets the error type to the value of
type
.Use
QSqlError
(constQString
&driverText
, constQString
&databaseText
,ErrorType
type, int number) insteadSee also
- PySide2.QtSql.QSqlError.swap(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlError
Swaps error
other
with this error. This operation is very fast and never fails.
- PySide2.QtSql.QSqlError.text()¶
- Return type:
str
This is a convenience function that returns
databaseText()
anddriverText()
concatenated into a single string.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.