QSqlField¶
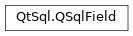
Synopsis¶
Functions¶
def
__eq__
(other)def
__ne__
(other)def
clear
()def
defaultValue
()def
isAutoValue
()def
isGenerated
()def
isNull
()def
isReadOnly
()def
isValid
()def
length
()def
name
()def
precision
()def
requiredStatus
()def
setAutoValue
(autoVal)def
setDefaultValue
(value)def
setGenerated
(gen)def
setLength
(fieldLength)def
setName
(name)def
setPrecision
(precision)def
setReadOnly
(readOnly)def
setRequired
(required)def
setRequiredStatus
(status)def
setSqlType
(type)def
setTableName
(tableName)def
setType
(type)def
setValue
(value)def
tableName
()def
type
()def
typeID
()def
value
()
Detailed Description¶
QSqlField
represents the characteristics of a single column in a database table or view, such as the data type and column name. A field also contains the value of the database column, which can be viewed or changed.Field data values are stored as QVariants. Using an incompatible type is not permitted. For example:
field = QSqlField("age", QVariant.Int) field.setValue(QPixmap()) # WRONGHowever, the field will attempt to cast certain data types to the field data type where possible:
field = QSqlField("age", QVariant.Int) field.setValue(str(123)) # casts str to int
QSqlField
objects are rarely created explicitly in application code. They are usually accessed indirectly throughQSqlRecord
s that already contain a list of fields. For example:query = QSqlQuery() ... record = query.record() field = record.field("country")A
QSqlField
object can provide some meta-data about the field, for example, itsname()
, varianttype()
,length()
,precision()
,defaultValue()
, , and itsrequiredStatus()
,isGenerated()
andisReadOnly()
. The field’s data can be checked to see if itisNull()
, and itsvalue()
retrieved. When editing the data can be set withsetValue()
or set to NULL withclear()
.See also
- class PySide2.QtSql.QSqlField(other)¶
PySide2.QtSql.QSqlField([fieldName=””[, type={}]])
PySide2.QtSql.QSqlField(fieldName, type, tableName)
- param type:
QVariant::Type
- param other:
- param fieldName:
str
- param tableName:
str
Constructs a copy of
other
.Constructs an empty field called
fieldName
of variant typetype
.See also
setRequiredStatus()
setLength()
setPrecision()
setDefaultValue()
setGenerated()
setReadOnly()
This is an overloaded function.
Constructs an empty field called
fieldName
of variant typetype
intable
.
- PySide2.QtSql.QSqlField.RequiredStatus¶
Specifies whether the field is required or optional.
Constant
Description
QSqlField.Required
The field must be specified when inserting records.
QSqlField.Optional
The fields doesn’t have to be specified when inserting records.
QSqlField.Unknown
The database driver couldn’t determine whether the field is required or optional.
See also
- PySide2.QtSql.QSqlField.clear()¶
Clears the value of the field and sets it to NULL. If the field is read-only, nothing happens.
See also
- PySide2.QtSql.QSqlField.defaultValue()¶
- Return type:
object
Returns the field’s default value (which may be NULL).
- PySide2.QtSql.QSqlField.isAutoValue()¶
- Return type:
bool
Returns
true
if the value is auto-generated by the database, for example auto-increment primary key values.Note
When using the ODBC driver, due to limitations in the ODBC API, the
isAutoValue()
field is only populated in aQSqlField
resulting from aQSqlRecord
obtained by executing aSELECT
query. It isfalse
in aQSqlField
resulting from aQSqlRecord
returned fromrecord()
orprimaryIndex()
.See also
- PySide2.QtSql.QSqlField.isGenerated()¶
- Return type:
bool
Returns
true
if the field is generated; otherwise returns false.
- PySide2.QtSql.QSqlField.isNull()¶
- Return type:
bool
Returns
true
if the field’s value is NULL; otherwise returns false.See also
- PySide2.QtSql.QSqlField.isReadOnly()¶
- Return type:
bool
Returns
true
if the field’s value is read-only; otherwise returns false.
- PySide2.QtSql.QSqlField.isValid()¶
- Return type:
bool
Returns
true
if the field’s variant type is valid; otherwise returnsfalse
.
- PySide2.QtSql.QSqlField.length()¶
- Return type:
int
Returns the field’s length.
If the returned value is negative, it means that the information is not available from the database.
- PySide2.QtSql.QSqlField.__ne__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlField
- Return type:
bool
Returns
true
if the field is unequal toother
; otherwise returns false.
- PySide2.QtSql.QSqlField.__eq__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlField
- Return type:
bool
Returns
true
if the field is equal toother
; otherwise returns false.
- PySide2.QtSql.QSqlField.precision()¶
- Return type:
int
Returns the field’s precision; this is only meaningful for numeric types.
If the returned value is negative, it means that the information is not available from the database.
- PySide2.QtSql.QSqlField.requiredStatus()¶
- Return type:
Returns
true
if this is a required field; otherwise returnsfalse
. AnINSERT
will fail if a required field does not have a value.
- PySide2.QtSql.QSqlField.setAutoValue(autoVal)¶
- Parameters:
autoVal – bool
Marks the field as an auto-generated value if
autoVal
is true.See also
- PySide2.QtSql.QSqlField.setDefaultValue(value)¶
- Parameters:
value – object
Sets the default value used for this field to
value
.
- PySide2.QtSql.QSqlField.setGenerated(gen)¶
- Parameters:
gen – bool
Sets the generated state. If
gen
is false, no SQL will be generated for this field; otherwise, Qt classes such asQSqlQueryModel
andQSqlTableModel
will generate SQL for this field.
- PySide2.QtSql.QSqlField.setLength(fieldLength)¶
- Parameters:
fieldLength – int
Sets the field’s length to
fieldLength
. For strings this is the maximum number of characters the string can hold; the meaning varies for other types.
- PySide2.QtSql.QSqlField.setName(name)¶
- Parameters:
name – str
Sets the name of the field to
name
.See also
- PySide2.QtSql.QSqlField.setPrecision(precision)¶
- Parameters:
precision – int
Sets the field’s
precision
. This only affects numeric fields.
- PySide2.QtSql.QSqlField.setReadOnly(readOnly)¶
- Parameters:
readOnly – bool
Sets the read only flag of the field’s value to
readOnly
. A read-only field cannot have its value set withsetValue()
and cannot be cleared to NULL withclear()
.See also
- PySide2.QtSql.QSqlField.setRequired(required)¶
- Parameters:
required – bool
Sets the required status of this field to
Required
ifrequired
is true; otherwise sets it toOptional
.See also
- PySide2.QtSql.QSqlField.setRequiredStatus(status)¶
- Parameters:
status –
RequiredStatus
Sets the required status of this field to
required
.
- PySide2.QtSql.QSqlField.setSqlType(type)¶
- Parameters:
type – int
- PySide2.QtSql.QSqlField.setTableName(tableName)¶
- Parameters:
tableName – str
Sets the
tableName
of the field totable
.See also
- PySide2.QtSql.QSqlField.setType(type)¶
- Parameters:
type –
QVariant::Type
Set’s the field’s variant type to
type
.
- PySide2.QtSql.QSqlField.setValue(value)¶
- Parameters:
value – object
Sets the value of the field to
value
. If the field is read-only (isReadOnly()
returnstrue
), nothing happens.If the data type of
value
differs from the field’s current data type, an attempt is made to cast it to the proper type. This preserves the data type of the field in the case of assignment, e.g. aQString
to an integer data type.To set the value to NULL, use
clear()
.See also
- PySide2.QtSql.QSqlField.tableName()¶
- Return type:
str
Returns the of the field.
Note
When using the QPSQL driver, due to limitations in the libpq library, the
tableName()
field is not populated in aQSqlField
resulting from aQSqlRecord
obtained byrecord()
of a forward-only query.See also
- PySide2.QtSql.QSqlField.type()¶
- Return type:
QVariant::Type
Returns the field’s type as stored in the database. Note that the actual value might have a different type, Numerical values that are too large to store in a long int or double are usually stored as strings to prevent precision loss.
See also
- PySide2.QtSql.QSqlField.typeID()¶
- Return type:
int
Returns the type ID for the field.
If the returned value is negative, it means that the information is not available from the database.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.