QSqlRecord¶
The
QSqlRecord
class encapsulates a database record. More…
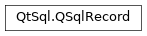
Inherited by: QSqlIndex
Synopsis¶
Functions¶
def
__eq__
(other)def
__ne__
(other)def
append
(field)def
clear
()def
clearValues
()def
contains
(name)def
count
()def
field
(i)def
field
(name)def
fieldName
(i)def
indexOf
(name)def
insert
(pos, field)def
isEmpty
()def
isGenerated
(i)def
isGenerated
(name)def
isNull
(i)def
isNull
(name)def
keyValues
(keyFields)def
remove
(pos)def
replace
(pos, field)def
setGenerated
(i, generated)def
setGenerated
(name, generated)def
setNull
(i)def
setNull
(name)def
setValue
(i, val)def
setValue
(name, val)def
value
(i)def
value
(name)
Detailed Description¶
The
QSqlRecord
class encapsulates the functionality and characteristics of a database record (usually a row in a table or view within the database).QSqlRecord
supports adding and removing fields as well as setting and retrieving field values.The values of a record’s fields can be set by name or position with
setValue()
; if you want to set a field to null usesetNull()
. To find the position of a field by name useindexOf()
, and to find the name of a field at a particular position usefieldName()
. Usefield()
to retrieve aQSqlField
object for a given field. Usecontains()
to see if the record contains a particular field name.When queries are generated to be executed on the database only those fields for which
isGenerated()
is true are included in the generated SQL.A record can have fields added with
append()
orinsert()
, replaced withreplace()
, and removed withremove()
. All the fields can be removed withclear()
. The number of fields is given bycount()
; all their values can be cleared (to null) usingclearValues()
.
- class PySide2.QtSql.QSqlRecord¶
PySide2.QtSql.QSqlRecord(other)
- param other:
Constructs an empty record.
Constructs a copy of
other
.QSqlRecord
is implicitly shared . This means you can make copies of a record in constant time .
- PySide2.QtSql.QSqlRecord.append(field)¶
- Parameters:
field –
PySide2.QtSql.QSqlField
Append a copy of field
field
to the end of the record.
- PySide2.QtSql.QSqlRecord.clear()¶
Removes all the record’s fields.
See also
- PySide2.QtSql.QSqlRecord.clearValues()¶
Clears the value of all fields in the record and sets each field to null.
See also
- PySide2.QtSql.QSqlRecord.contains(name)¶
- Parameters:
name – str
- Return type:
bool
Returns
true
if there is a field in the record calledname
; otherwise returnsfalse
.
- PySide2.QtSql.QSqlRecord.count()¶
- Return type:
int
Returns the number of fields in the record.
See also
- PySide2.QtSql.QSqlRecord.field(name)¶
- Parameters:
name – str
- Return type:
- PySide2.QtSql.QSqlRecord.field(i)
- Parameters:
i – int
- Return type:
Returns the field at position
index
. If theindex
is out of range, function returns a default-constructed value .
- PySide2.QtSql.QSqlRecord.fieldName(i)¶
- Parameters:
i – int
- Return type:
str
Returns the name of the field at position
index
. If the field does not exist, an empty string is returned.See also
- PySide2.QtSql.QSqlRecord.indexOf(name)¶
- Parameters:
name – str
- Return type:
int
Returns the position of the field called
name
within the record, or -1 if it cannot be found. Field names are not case-sensitive. If more than one field matches, the first one is returned.See also
- PySide2.QtSql.QSqlRecord.insert(pos, field)¶
- Parameters:
pos – int
field –
PySide2.QtSql.QSqlField
Inserts the field
field
at positionpos
in the record.
- PySide2.QtSql.QSqlRecord.isEmpty()¶
- Return type:
bool
Returns
true
if there are no fields in the record; otherwise returnsfalse
.
- PySide2.QtSql.QSqlRecord.isGenerated(name)¶
- Parameters:
name – str
- Return type:
bool
- PySide2.QtSql.QSqlRecord.isGenerated(i)
- Parameters:
i – int
- Return type:
bool
This is an overloaded function.
Returns
true
if the record has a field at positionindex
and this field is to be generated (the default); otherwise returnsfalse
.See also
- PySide2.QtSql.QSqlRecord.isNull(name)¶
- Parameters:
name – str
- Return type:
bool
- PySide2.QtSql.QSqlRecord.isNull(i)
- Parameters:
i – int
- Return type:
bool
This is an overloaded function.
Returns
true
if the fieldindex
is null or if there is no field at positionindex
; otherwise returnsfalse
.
- PySide2.QtSql.QSqlRecord.keyValues(keyFields)¶
- Parameters:
keyFields –
PySide2.QtSql.QSqlRecord
- Return type:
Returns a record containing the fields represented in
keyFields
set to values that match by field name.
- PySide2.QtSql.QSqlRecord.__ne__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlRecord
- Return type:
bool
Returns
true
if this object is not identical toother
; otherwise returnsfalse
.See also
operator==()
- PySide2.QtSql.QSqlRecord.__eq__(other)¶
- Parameters:
other –
PySide2.QtSql.QSqlRecord
- Return type:
bool
Returns
true
if this object is identical toother
(i.e., has the same fields in the same order); otherwise returnsfalse
.See also
operator!=()
- PySide2.QtSql.QSqlRecord.remove(pos)¶
- Parameters:
pos – int
Removes the field at position
pos
. Ifpos
is out of range, nothing happens.
- PySide2.QtSql.QSqlRecord.replace(pos, field)¶
- Parameters:
pos – int
field –
PySide2.QtSql.QSqlField
Replaces the field at position
pos
with the givenfield
. Ifpos
is out of range, nothing happens.
- PySide2.QtSql.QSqlRecord.setGenerated(name, generated)¶
- Parameters:
name – str
generated – bool
- PySide2.QtSql.QSqlRecord.setGenerated(i, generated)
- Parameters:
i – int
generated – bool
This is an overloaded function.
Sets the generated flag for the field
index
togenerated
.See also
- PySide2.QtSql.QSqlRecord.setNull(name)¶
- Parameters:
name – str
- PySide2.QtSql.QSqlRecord.setNull(i)
- Parameters:
i – int
Sets the value of field
index
to null. If the field does not exist, nothing happens.See also
- PySide2.QtSql.QSqlRecord.setValue(name, val)¶
- Parameters:
name – str
val – object
- PySide2.QtSql.QSqlRecord.setValue(i, val)
- Parameters:
i – int
val – object
- PySide2.QtSql.QSqlRecord.value(name)¶
- Parameters:
name – str
- Return type:
object
- PySide2.QtSql.QSqlRecord.value(i)
- Parameters:
i – int
- Return type:
object
Returns the value of the field located at position
index
in the record. Ifindex
is out of bounds, an invalidQVariant
is returned.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.