QFontDatabase¶
The
QFontDatabase
class provides information about the fonts available in the underlying window system. More…
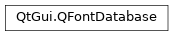
Synopsis¶
Functions¶
def
bold
(family, style)def
families
([writingSystem=Any])def
font
(family, style, pointSize)def
hasFamily
(family)def
isBitmapScalable
(family[, style=””])def
isFixedPitch
(family[, style=””])def
isPrivateFamily
(family)def
isScalable
(family[, style=””])def
isSmoothlyScalable
(family[, style=””])def
italic
(family, style)def
pointSizes
(family[, style=””])def
smoothSizes
(family, style)def
styleString
(font)def
styleString
(fontInfo)def
styles
(family)def
weight
(family, style)def
writingSystems
()def
writingSystems
(family)
Static functions¶
def
addApplicationFont
(fileName)def
addApplicationFontFromData
(fontData)def
applicationFontFamilies
(id)def
removeAllApplicationFonts
()def
removeApplicationFont
(id)def
standardSizes
()def
systemFont
(type)def
writingSystemName
(writingSystem)def
writingSystemSample
(writingSystem)
Detailed Description¶
The most common uses of this class are to query the database for the list of font
families()
and for thepointSizes()
andstyles()
that are available for each family. An alternative topointSizes()
issmoothSizes()
which returns the sizes at which a given family and style will look attractive.If the font family is available from two or more foundries the foundry name is included in the family name; for example: “Helvetica [Adobe]” and “Helvetica [Cronyx]”. When you specify a family, you can either use the old hyphenated “foundry-family” format or the bracketed “family [foundry]” format; for example: “Cronyx-Helvetica” or “Helvetica [Cronyx]”. If the family has a foundry it is always returned using the bracketed format, as is the case with the value returned by
families()
.The
font()
function returns aQFont
given a family, style and point size.A family and style combination can be checked to see if it is
italic()
orbold()
, and to retrieve itsweight()
. Similarly we can callisBitmapScalable()
,isSmoothlyScalable()
,isScalable()
andisFixedPitch()
.Use the
styleString()
to obtain a text version of a style.The
QFontDatabase
class also supports some static functions, for example,standardSizes()
. You can retrieve the description of a writing system usingwritingSystemName()
, and a sample of characters in a writing system withwritingSystemSample()
.Example:
database = QFontDatabase() fontTree = QTreeWidget() fontTree.setColumnCount(2) fontTree.setHeaderLabels(QStringList() << "Font" << "Smooth Sizes") for family in database.families(): familyItem = QTreeWidgetItem(fontTree) familyItem.setText(0, family) for style in database.styles(family): styleItem = QTreeWidgetItem(familyItem) styleItem.setText(0, style) sizes = 0 for points in database.smoothSizes(family, style): sizes += QString.number(points) + " " styleItem.setText(1, sizes.trimmed())This example gets the list of font families, the list of styles for each family, and the point sizes that are available for each combination of family and style, displaying this information in a tree view.
See also
- class PySide2.QtGui.QFontDatabase¶
PySide2.QtGui.QFontDatabase(QFontDatabase)
- param QFontDatabase:
Creates a font database object.
- PySide2.QtGui.QFontDatabase.WritingSystem¶
Constant
Description
QFontDatabase.Any
QFontDatabase.Latin
QFontDatabase.Greek
QFontDatabase.Cyrillic
QFontDatabase.Armenian
QFontDatabase.Hebrew
QFontDatabase.Arabic
QFontDatabase.Syriac
QFontDatabase.Thaana
QFontDatabase.Devanagari
QFontDatabase.Bengali
QFontDatabase.Gurmukhi
QFontDatabase.Gujarati
QFontDatabase.Oriya
QFontDatabase.Tamil
QFontDatabase.Telugu
QFontDatabase.Kannada
QFontDatabase.Malayalam
QFontDatabase.Sinhala
QFontDatabase.Thai
QFontDatabase.Lao
QFontDatabase.Tibetan
QFontDatabase.Myanmar
QFontDatabase.Georgian
QFontDatabase.Khmer
QFontDatabase.SimplifiedChinese
QFontDatabase.TraditionalChinese
QFontDatabase.Japanese
QFontDatabase.Korean
QFontDatabase.Vietnamese
QFontDatabase.Symbol
QFontDatabase.Other
(the same as Symbol)
QFontDatabase.Ogham
QFontDatabase.Runic
QFontDatabase.Nko
- PySide2.QtGui.QFontDatabase.SystemFont¶
Constant
Description
QFontDatabase.GeneralFont
The default system font.
QFontDatabase.FixedFont
The fixed font that the system recommends.
QFontDatabase.TitleFont
The system standard font for titles.
QFontDatabase.SmallestReadableFont
The smallest readable system font.
- static PySide2.QtGui.QFontDatabase.addApplicationFont(fileName)¶
- Parameters:
fileName – str
- Return type:
int
Loads the font from the file specified by
fileName
and makes it available to the application. An ID is returned that can be used to remove the font again withremoveApplicationFont()
or to retrieve the list of family names contained in the font.The function returns -1 if the font could not be loaded.
Currently only TrueType fonts, TrueType font collections, and OpenType fonts are supported.
- static PySide2.QtGui.QFontDatabase.addApplicationFontFromData(fontData)¶
- Parameters:
fontData –
PySide2.QtCore.QByteArray
- Return type:
int
Loads the font from binary data specified by
fontData
and makes it available to the application. An ID is returned that can be used to remove the font again withremoveApplicationFont()
or to retrieve the list of family names contained in the font.The function returns -1 if the font could not be loaded.
Currently only TrueType fonts, TrueType font collections, and OpenType fonts are supported.
- static PySide2.QtGui.QFontDatabase.applicationFontFamilies(id)¶
- Parameters:
id – int
- Return type:
list of strings
Returns a list of font families for the given application font identified by
id
.
- PySide2.QtGui.QFontDatabase.bold(family, style)¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is bold; otherwise returnsfalse
.
- PySide2.QtGui.QFontDatabase.families([writingSystem=Any])¶
- Parameters:
writingSystem –
WritingSystem
- Return type:
list of strings
Returns a sorted list of the available font families which support the
writingSystem
.If a family exists in several foundries, the returned name for that font is in the form “family [foundry]”. Examples: “Times [Adobe]”, “Times [Cronyx]”, “Palatino”.
See also
- PySide2.QtGui.QFontDatabase.font(family, style, pointSize)¶
- Parameters:
family – str
style – str
pointSize – int
- Return type:
Returns a
QFont
object that has familyfamily
, stylestyle
and point sizepointSize
. If no matching font could be created, aQFont
object that uses the application’s default font is returned.
- PySide2.QtGui.QFontDatabase.hasFamily(family)¶
- Parameters:
family – str
- Return type:
bool
- PySide2.QtGui.QFontDatabase.isBitmapScalable(family[, style=""])¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is a scalable bitmap font; otherwise returnsfalse
. Scaling a bitmap font usually produces an unattractive hardly readable result, because the pixels of the font are scaled. If you need to scale a bitmap font it is better to scale it to one of the fixed sizes returned bysmoothSizes()
.See also
- PySide2.QtGui.QFontDatabase.isFixedPitch(family[, style=""])¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is fixed pitch; otherwise returnsfalse
.
- PySide2.QtGui.QFontDatabase.isPrivateFamily(family)¶
- Parameters:
family – str
- Return type:
bool
Returns
true
if and only if thefamily
font family is private.This happens, for instance, on macOS and iOS, where the system UI fonts are not accessible to the user. For completeness,
families()
returns all font families, including the private ones. You should use this function if you are developing a font selection control in order to keep private fonts hidden.See also
- PySide2.QtGui.QFontDatabase.isScalable(family[, style=""])¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is scalable; otherwise returnsfalse
.See also
- PySide2.QtGui.QFontDatabase.isSmoothlyScalable(family[, style=""])¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is smoothly scalable; otherwise returnsfalse
. If this function returnstrue
, it’s safe to scale this font to any size, and the result will always look attractive.See also
- PySide2.QtGui.QFontDatabase.italic(family, style)¶
- Parameters:
family – str
style – str
- Return type:
bool
Returns
true
if the font that has familyfamily
and stylestyle
is italic; otherwise returnsfalse
.
- PySide2.QtGui.QFontDatabase.pointSizes(family[, style=""])¶
- Parameters:
family – str
style – str
- Return type:
Returns a list of the point sizes available for the font that has family
family
and stylestyleName
. The list may be empty.See also
- static PySide2.QtGui.QFontDatabase.removeAllApplicationFonts()¶
- Return type:
bool
Removes all application-local fonts previously added using
addApplicationFont()
andaddApplicationFontFromData()
.Returns
true
if unloading of the fonts succeeded; otherwise returnsfalse
.
- static PySide2.QtGui.QFontDatabase.removeApplicationFont(id)¶
- Parameters:
id – int
- Return type:
bool
Removes the previously loaded application font identified by
id
. Returnstrue
if unloading of the font succeeded; otherwise returnsfalse
.
- PySide2.QtGui.QFontDatabase.smoothSizes(family, style)¶
- Parameters:
family – str
style – str
- Return type:
Returns the point sizes of a font that has family
family
and stylestyleName
that will look attractive. The list may be empty. For non-scalable fonts and bitmap scalable fonts, this function is equivalent topointSizes()
.See also
- static PySide2.QtGui.QFontDatabase.standardSizes()¶
- Return type:
Returns a list of standard font sizes.
See also
- PySide2.QtGui.QFontDatabase.styleString(font)¶
- Parameters:
font –
PySide2.QtGui.QFont
- Return type:
str
- PySide2.QtGui.QFontDatabase.styleString(fontInfo)
- Parameters:
fontInfo –
PySide2.QtGui.QFontInfo
- Return type:
str
- PySide2.QtGui.QFontDatabase.styles(family)¶
- Parameters:
family – str
- Return type:
list of strings
Returns a list of the styles available for the font family
family
. Some example styles: “Light”, “Light Italic”, “Bold”, “Oblique”, “Demi”. The list may be empty.See also
- static PySide2.QtGui.QFontDatabase.supportsThreadedFontRendering()¶
- Return type:
bool
Note
This function is deprecated.
Returns
true
if font rendering is supported outside the GUI thread, false otherwise. In other words, a return value of false means that alldrawText()
calls outside the GUI thread will not produce readable output.As of 5.0, always returns
true
.See also
Painting In Threads
- static PySide2.QtGui.QFontDatabase.systemFont(type)¶
- Parameters:
type –
SystemFont
- Return type:
Returns the most adequate font for a given
type
case for proper integration with the system’s look and feel.See also
- PySide2.QtGui.QFontDatabase.weight(family, style)¶
- Parameters:
family – str
style – str
- Return type:
int
Returns the weight of the font that has family
family
and stylestyle
. If there is no such family and style combination, returns -1.
- static PySide2.QtGui.QFontDatabase.writingSystemName(writingSystem)¶
- Parameters:
writingSystem –
WritingSystem
- Return type:
str
Returns the names the
writingSystem
(e.g. for displaying to the user in a dialog).
- static PySide2.QtGui.QFontDatabase.writingSystemSample(writingSystem)¶
- Parameters:
writingSystem –
WritingSystem
- Return type:
str
Returns a string with sample characters from
writingSystem
.
- PySide2.QtGui.QFontDatabase.writingSystems()¶
- Return type:
Returns a sorted list of the available writing systems. This is list generated from information about all installed fonts on the system.
See also
- PySide2.QtGui.QFontDatabase.writingSystems(family)
- Parameters:
family – str
- Return type:
Returns a sorted list of the writing systems supported by a given font
family
.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.