QPalette¶
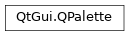
Synopsis¶
Functions¶
def
__eq__
(p)def
__ne__
(p)def
alternateBase
()def
background
()def
base
()def
brightText
()def
brush
(cg, cr)def
brush
(cr)def
button
()def
buttonText
()def
cacheKey
()def
color
(cg, cr)def
color
(cr)def
currentColorGroup
()def
dark
()def
foreground
()def
highlight
()def
highlightedText
()def
isBrushSet
(cg, cr)def
isCopyOf
(p)def
isEqual
(cr1, cr2)def
light
()def
link
()def
linkVisited
()def
mid
()def
midlight
()def
placeholderText
()def
resolve
()def
resolve
(arg__1)def
resolve
(mask)def
setBrush
(cg, cr, brush)def
setBrush
(cr, brush)def
setColor
(cg, cr, color)def
setColor
(cr, color)def
setColorGroup
(cr, windowText, button, light, dark, mid, text, bright_text, base, window)def
setCurrentColorGroup
(cg)def
shadow
()def
swap
(other)def
text
()def
toolTipBase
()def
toolTipText
()def
window
()def
windowText
()
Detailed Description¶
A palette consists of three color groups: Active , Disabled , and Inactive . All widgets in Qt contain a palette and use their palette to draw themselves. This makes the user interface easily configurable and easier to keep consistent.
If you create a new widget we strongly recommend that you use the colors in the palette rather than hard-coding specific colors.
The color groups:
The Active group is used for the window that has keyboard focus.
The Inactive group is used for other windows.
The Disabled group is used for widgets (not windows) that are disabled for some reason.
Both active and inactive windows can contain disabled widgets. (Disabled widgets are often called inaccessible or grayed out .)
In most styles, Active and Inactive look the same.
Colors and brushes can be set for particular roles in any of a palette’s color groups with
setColor()
andsetBrush()
. A color group contains a group of colors used by widgets for drawing themselves. We recommend that widgets use color group roles from the palette such as “foreground” and “base” rather than literal colors like “red” or “turquoise”. The color roles are enumerated and defined in theColorRole
documentation.We strongly recommend that you use the default palette of the current style (returned by
palette()
) and modify that as necessary. This is done by Qt’s widgets when they are drawn.To modify a color group you call the functions
setColor()
andsetBrush()
, depending on whether you want a pure color or a pixmap pattern.There are also corresponding
color()
andbrush()
getters, and a commonly used convenience function to get theColorRole
for the currentColorGroup
:window()
,windowText()
,base()
, etc.You can copy a palette using the copy constructor and test to see if two palettes are identical using
isCopyOf()
.
QPalette
is optimized by the use of implicit sharing , so it is very efficient to passQPalette
objects as arguments.Warning
Some styles do not use the palette for all drawing, for instance, if they make use of native theme engines. This is the case for both the Windows Vista and the macOS styles.
See also
setPalette()
setPalette()
QColor
- class PySide2.QtGui.QPalette¶
PySide2.QtGui.QPalette(button)
PySide2.QtGui.QPalette(windowText, button, light, dark, mid, text, bright_text, base, window)
PySide2.QtGui.QPalette(button)
PySide2.QtGui.QPalette(button, window)
PySide2.QtGui.QPalette(windowText, window, light, dark, mid, text, base)
PySide2.QtGui.QPalette(palette)
- param palette:
- param windowText:
- param mid:
- param base:
- param bright_text:
- param button:
- param dark:
- param light:
- param text:
- param window:
Constructs an empty palette object with no color roles set.
When used as the palette of a
QWidget
the colors are resolved as described bysetPalette()
.See also
setPalette()
palette()
Constructs a palette from the
button
color. The other colors are automatically calculated, based on this color.Window
will be the button color as well.Constructs a palette. You can pass either brushes, pixmaps or plain colors for
windowText
,button
,light
,dark
,mid
,text
,bright_text
,base
andwindow
.See also
Constructs a palette from a
button
color and awindow
. The other colors are automatically calculated, based on these colors.Constructs a palette with the specified
windowText
,window
,light
,dark
,mid
,text
, andbase
colors. The button color will be set to the window color.
- PySide2.QtGui.QPalette.ColorGroup¶
Constant
Description
QPalette.Disabled
QPalette.Active
QPalette.Inactive
QPalette.Normal
synonym for Active
- PySide2.QtGui.QPalette.ColorRole¶
The enum defines the different symbolic color roles used in current GUIs.
The central roles are:
Constant
Description
QPalette.Window
A general background color.
QPalette.Background
This value is obsolete. Use Window instead.
QPalette.WindowText
A general foreground color.
QPalette.Foreground
This value is obsolete. Use instead.
QPalette.Base
Used mostly as the background color for text entry widgets, but can also be used for other painting - such as the background of combobox drop down lists and toolbar handles. It is usually white or another light color.
QPalette.AlternateBase
Used as the alternate background color in views with alternating row colors (see
setAlternatingRowColors()
).QPalette.ToolTipBase
Used as the background color for
QToolTip
andQWhatsThis
. Tool tips use the Inactive color group ofQPalette
, because tool tips are not active windows.QPalette.ToolTipText
Used as the foreground color for
QToolTip
andQWhatsThis
. Tool tips use the Inactive color group ofQPalette
, because tool tips are not active windows.QPalette.PlaceholderText
Used as the placeholder color for various text input widgets. This enum value has been introduced in Qt 5.12
QPalette.Text
The foreground color used with
Base
. This is usually the same as theWindowText
, in which case it must provide good contrast withWindow
andBase
.QPalette.Button
The general button background color. This background can be different from
Window
as some styles require a different background color for buttons.QPalette.ButtonText
A foreground color used with the
Button
color.QPalette.BrightText
A text color that is very different from
WindowText
, and contrasts well with e.g.Dark
. Typically used for text that needs to be drawn whereText
orWindowText
would give poor contrast, such as on pressed push buttons. Note that text colors can be used for things other than just words; text colors are usually used for text, but it’s quite common to use the text color roles for lines, icons, etc.There are some color roles used mostly for 3D bevel and shadow effects. All of these are normally derived from
Window
, and used in ways that depend on that relationship. For example, buttons depend on it to make the bevels look attractive, and Motif scroll bars depend onMid
to be slightly different fromWindow
.Constant
Description
QPalette.Light
Lighter than
Button
color.QPalette.Midlight
Between
Button
andLight
.QPalette.Dark
Darker than
Button
.QPalette.Mid
Between
Button
andDark
.QPalette.Shadow
A very dark color. By default, the shadow color is
black
.Selected (marked) items have two roles:
Constant
Description
QPalette.Highlight
A color to indicate a selected item or the current item. By default, the highlight color is
darkBlue
.QPalette.HighlightedText
A text color that contrasts with
Highlight
. By default, the highlighted text color iswhite
.There are two color roles related to hyperlinks:
Constant
Description
QPalette.Link
A text color used for unvisited hyperlinks. By default, the link color is
blue
.QPalette.LinkVisited
A text color used for already visited hyperlinks. By default, the linkvisited color is
magenta
.Note that we do not use the
Link
andLinkVisited
roles when rendering rich text in Qt, and that we recommend that you use CSS and thesetDefaultStyleSheet()
function to alter the appearance of links. For example:browser = QTextBrowser() linkColor = QColor(Qt.red) sheet = QString.fromLatin1("a { text-decoration: underline color: %1 }").arg(linkColor.name()) browser.document().setDefaultStyleSheet(sheet)
Constant
Description
QPalette.NoRole
No role; this special role is often used to indicate that a role has not been assigned.
- PySide2.QtGui.QPalette.alternateBase()¶
- Return type:
Returns the alternate base brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.background()¶
- Return type:
Note
This function is deprecated.
Use
window()
instead.
- PySide2.QtGui.QPalette.base()¶
- Return type:
Returns the base brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.brightText()¶
- Return type:
Returns the bright text foreground brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.brush(cg, cr)¶
- Parameters:
cg –
ColorGroup
cr –
ColorRole
- Return type:
Returns the brush in the specified color
group
, used for the given colorrole
.See also
color()
setBrush()
ColorRole
- PySide2.QtGui.QPalette.brush(cr)
- Parameters:
cr –
ColorRole
- Return type:
This is an overloaded function.
Returns the brush that has been set for the given color
role
in the currentColorGroup
.See also
color()
setBrush()
ColorRole
- PySide2.QtGui.QPalette.button()¶
- Return type:
Returns the button brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.buttonText()¶
- Return type:
Returns the button text foreground brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.cacheKey()¶
- Return type:
int
Returns a number that identifies the contents of this
QPalette
object. DistinctQPalette
objects can have the same key if they refer to the same contents.The will change when the palette is altered.
- PySide2.QtGui.QPalette.color(cg, cr)¶
- Parameters:
cg –
ColorGroup
cr –
ColorRole
- Return type:
Returns the color in the specified color
group
, used for the given colorrole
.See also
brush()
setColor()
ColorRole
- PySide2.QtGui.QPalette.color(cr)
- Parameters:
cr –
ColorRole
- Return type:
This is an overloaded function.
Returns the color that has been set for the given color
role
in the currentColorGroup
.See also
brush()
ColorRole
- PySide2.QtGui.QPalette.currentColorGroup()¶
- Return type:
Returns the palette’s current color group.
See also
- PySide2.QtGui.QPalette.dark()¶
- Return type:
Returns the dark brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.foreground()¶
- Return type:
Note
This function is deprecated.
Use
windowText()
instead.
- PySide2.QtGui.QPalette.highlight()¶
- Return type:
Returns the highlight brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.highlightedText()¶
- Return type:
Returns the highlighted text brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.isBrushSet(cg, cr)¶
- Parameters:
cg –
ColorGroup
cr –
ColorRole
- Return type:
bool
Returns
true
if theColorGroup
cg
andColorRole
cr
has been set previously on this palette; otherwise returnsfalse
.See also
- PySide2.QtGui.QPalette.isCopyOf(p)¶
- Parameters:
- Return type:
bool
Returns
true
if this palette andp
are copies of each other, i.e. one of them was created as a copy of the other and neither was subsequently modified; otherwise returnsfalse
. This is much stricter than equality.See also
operator=()
operator==()
- PySide2.QtGui.QPalette.isEqual(cr1, cr2)¶
- Parameters:
cr1 –
ColorGroup
cr2 –
ColorGroup
- Return type:
bool
Returns
true
(usually quickly) if color groupcg1
is equal tocg2
; otherwise returnsfalse
.
- PySide2.QtGui.QPalette.light()¶
- Return type:
Returns the light brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.link()¶
- Return type:
Returns the unvisited link text brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.linkVisited()¶
- Return type:
Returns the visited link text brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.mid()¶
- Return type:
Returns the mid brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.midlight()¶
- Return type:
Returns the midlight brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.__ne__(p)¶
- Parameters:
- Return type:
bool
Returns
true
(slowly) if this palette is different fromp
; otherwise returnsfalse
(usually quickly).Note
The current
ColorGroup
is not taken into account when comparing palettesSee also
operator==()
- PySide2.QtGui.QPalette.__eq__(p)¶
- Parameters:
- Return type:
bool
Returns
true
(usually quickly) if this palette is equal top
; otherwise returnsfalse
(slowly).Note
The current
ColorGroup
is not taken into account when comparing palettesSee also
operator!=()
- PySide2.QtGui.QPalette.placeholderText()¶
- Return type:
Returns the placeholder text brush of the current color group.
Note
Before Qt 5.12, the placeholder text color was hard-coded in the code as
text()
.color()
where an alpha of 128 was applied. We continue to support this behavior by default, unless you set your own brush. One can get back the original placeholder color setting the specialQBrush
default constructor as placeholder brush.See also
ColorRole
brush()
- PySide2.QtGui.QPalette.resolve()¶
- Return type:
uint
- PySide2.QtGui.QPalette.resolve(arg__1)
- Parameters:
arg__1 –
PySide2.QtGui.QPalette
- Return type:
Returns a new
QPalette
that is a union of this instance andother
. Color roles set in this instance take precedence.
- PySide2.QtGui.QPalette.resolve(mask)
- Parameters:
mask –
uint
- PySide2.QtGui.QPalette.setBrush(cr, brush)¶
- Parameters:
cr –
ColorRole
brush –
PySide2.QtGui.QBrush
Sets the brush for the given color
role
to the specifiedbrush
for all groups in the palette.See also
brush()
setColor()
ColorRole
- PySide2.QtGui.QPalette.setBrush(cg, cr, brush)
- Parameters:
cg –
ColorGroup
cr –
ColorRole
brush –
PySide2.QtGui.QBrush
This is an overloaded function.
Sets the brush in the specified color
group
, used for the given colorrole
, tobrush
.See also
brush()
setColor()
ColorRole
- PySide2.QtGui.QPalette.setColor(cg, cr, color)¶
- Parameters:
cg –
ColorGroup
cr –
ColorRole
color –
PySide2.QtGui.QColor
Sets the color in the specified color
group
, used for the given colorrole
, to the specified solidcolor
.See also
setBrush()
color()
ColorRole
- PySide2.QtGui.QPalette.setColor(cr, color)
- Parameters:
cr –
ColorRole
color –
PySide2.QtGui.QColor
This is an overloaded function.
Sets the color used for the given color
role
, in all color groups, to the specified solidcolor
.See also
brush()
setColor()
ColorRole
- PySide2.QtGui.QPalette.setColorGroup(cr, windowText, button, light, dark, mid, text, bright_text, base, window)¶
- Parameters:
cr –
ColorGroup
windowText –
PySide2.QtGui.QBrush
button –
PySide2.QtGui.QBrush
light –
PySide2.QtGui.QBrush
dark –
PySide2.QtGui.QBrush
mid –
PySide2.QtGui.QBrush
text –
PySide2.QtGui.QBrush
bright_text –
PySide2.QtGui.QBrush
base –
PySide2.QtGui.QBrush
window –
PySide2.QtGui.QBrush
Sets a the group at
cg
. You can pass either brushes, pixmaps or plain colors forwindowText
,button
,light
,dark
,mid
,text
,bright_text
,base
andwindow
.See also
- PySide2.QtGui.QPalette.setCurrentColorGroup(cg)¶
- Parameters:
cg –
ColorGroup
Set the palette’s current color group to
cg
.See also
- PySide2.QtGui.QPalette.shadow()¶
- Return type:
Returns the shadow brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.swap(other)¶
- Parameters:
other –
PySide2.QtGui.QPalette
Swaps this palette instance with
other
. This function is very fast and never fails.
- PySide2.QtGui.QPalette.text()¶
- Return type:
Returns the text foreground brush of the current color group.
See also
ColorRole
brush()
- PySide2.QtGui.QPalette.toolTipBase()¶
- Return type:
Returns the tool tip base brush of the current color group. This brush is used by
QToolTip
andQWhatsThis
.Note
Tool tips use the Inactive color group of
QPalette
, because tool tips are not active windows.See also
ColorRole
brush()
- PySide2.QtGui.QPalette.toolTipText()¶
- Return type:
Returns the tool tip text brush of the current color group. This brush is used by
QToolTip
andQWhatsThis
.Note
Tool tips use the Inactive color group of
QPalette
, because tool tips are not active windows.See also
ColorRole
brush()
- PySide2.QtGui.QPalette.window()¶
- Return type:
Returns the window (general background) brush of the current color group.
See also
ColorRole
brush()
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.