QBrush¶
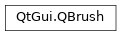
Synopsis¶
Functions¶
def
__eq__
(b)def
__ne__
(b)def
color
()def
gradient
()def
isOpaque
()def
matrix
()def
setColor
(color)def
setColor
(color)def
setMatrix
(mat)def
setStyle
(arg__1)def
setTexture
(pixmap)def
setTextureImage
(image)def
setTransform
(arg__1)def
style
()def
swap
(other)def
texture
()def
textureImage
()def
transform
()
Detailed Description¶
A brush has a style, a color, a gradient and a texture.
The brush
style()
defines the fill pattern using theBrushStyle
enum. The default brush style isNoBrush
(depending on how you construct a brush). This style tells the painter to not fill shapes. The standard style for filling isSolidPattern
. The style can be set when the brush is created using the appropriate constructor, and in addition thesetStyle()
function provides means for altering the style once the brush is constructed.![]()
The brush
color()
defines the color of the fill pattern. The color can either be one of Qt’s predefined colors,GlobalColor
, or any other customQColor
. The currently set color can be retrieved and altered using thecolor()
andsetColor()
functions, respectively.The
gradient()
defines the gradient fill used when the current style is eitherLinearGradientPattern
,RadialGradientPattern
orConicalGradientPattern
. Gradient brushes are created by giving aQGradient
as a constructor argument when creating theQBrush
. Qt provides three different gradients:QLinearGradient
,QConicalGradient
, andQRadialGradient
- all of which inheritQGradient
.QRadialGradient gradient(50, 50, 50, 50, 50); gradient.setColorAt(0, QColor::fromRgbF(0, 1, 0, 1)); gradient.setColorAt(1, QColor::fromRgbF(0, 0, 0, 0)); QBrush brush(gradient);The
texture()
defines the pixmap used when the current style isTexturePattern
. You can create a brush with a texture by providing the pixmap when the brush is created or by usingsetTexture()
.Note that applying
setTexture()
makesstyle()
==TexturePattern
, regardless of previous style settings. Also, callingsetColor()
will not make a difference if the style is a gradient. The same is the case if the style isTexturePattern
style unless the current texture is aQBitmap
.The
isOpaque()
function returnstrue
if the brush is fully opaque otherwise false. A brush is considered opaque if:
The alpha component of the
color()
is 255.Its
texture()
does not have an alpha channel and is not aQBitmap
.The colors in the
gradient()
all have an alpha component that is 255.
To specify the style and color of lines and outlines, use the
QPainter
‘spen
combined withPenStyle
andGlobalColor
:painter = QPainter(self) painter.setBrush(Qt.cyan) painter.setPen(Qt.darkCyan) painter.drawRect(0, 0, 100,100) painter.setBrush(Qt.NoBrush) painter.setPen(Qt.darkGreen) painter.drawRect(40, 40, 100, 100)Note that, by default,
QPainter
renders the outline (using the currently set pen) when drawing shapes. Usepainter.setPen(Qt::NoPen)
:attr:` <Qt.PenStyle>` to disable this behavior.For more information about painting in general, see the Paint System .
- class PySide2.QtGui.QBrush¶
PySide2.QtGui.QBrush(bs)
PySide2.QtGui.QBrush(color[, bs=Qt.SolidPattern])
PySide2.QtGui.QBrush(color, pixmap)
PySide2.QtGui.QBrush(brush)
PySide2.QtGui.QBrush(color[, bs=Qt.SolidPattern])
PySide2.QtGui.QBrush(color, pixmap)
PySide2.QtGui.QBrush(gradient)
PySide2.QtGui.QBrush(image)
PySide2.QtGui.QBrush(pixmap)
- param image:
- param bs:
- param brush:
- param color:
- param gradient:
- param pixmap:
Constructs a default black brush with the style
NoBrush
(i.e. this brush will not fill shapes).Constructs a black brush with the given
style
.See also
Constructs a brush with the given
color
andstyle
.See also
- PySide2.QtGui.QBrush.color()¶
- Return type:
Returns the brush color.
See also
- PySide2.QtGui.QBrush.gradient()¶
- Return type:
Returns the gradient describing this brush.
- PySide2.QtGui.QBrush.isOpaque()¶
- Return type:
bool
Returns
true
if the brush is fully opaque otherwise false. A brush is considered opaque if:The alpha component of the
color()
is 255.Its
texture()
does not have an alpha channel and is not aQBitmap
.The colors in the
gradient()
all have an alpha component that is 255.It is an extended radial gradient.
- PySide2.QtGui.QBrush.matrix()¶
- Return type:
Note
This function is deprecated.
Use
transform()
instead.Returns the current transformation matrix for the brush.
See also
- PySide2.QtGui.QBrush.__ne__(b)¶
- Parameters:
- Return type:
bool
Returns
true
if the brush is different from the givenbrush
; otherwise returnsfalse
.Two brushes are different if they have different styles, colors or transforms or different pixmaps or gradients depending on the style.
See also
operator==()
- PySide2.QtGui.QBrush.__eq__(b)¶
- Parameters:
- Return type:
bool
Returns
true
if the brush is equal to the givenbrush
; otherwise returnsfalse
.Two brushes are equal if they have equal styles, colors and transforms and equal pixmaps or gradients depending on the style.
See also
operator!=()
- PySide2.QtGui.QBrush.setColor(color)¶
- Parameters:
color –
GlobalColor
This is an overloaded function.
Sets the brush color to the given
color
.
- PySide2.QtGui.QBrush.setColor(color)
- Parameters:
color –
PySide2.QtGui.QColor
- PySide2.QtGui.QBrush.setMatrix(mat)¶
- Parameters:
mat –
PySide2.QtGui.QMatrix
Note
This function is deprecated.
Use
setTransform()
instead.Sets
matrix
as an explicit transformation matrix on the current brush. The brush transformation matrix is merged withQPainter
transformation matrix to produce the final result.See also
- PySide2.QtGui.QBrush.setStyle(arg__1)¶
- Parameters:
arg__1 –
BrushStyle
Sets the brush style to
style
.See also
- PySide2.QtGui.QBrush.setTexture(pixmap)¶
- Parameters:
pixmap –
PySide2.QtGui.QPixmap
Sets the brush pixmap to
pixmap
. The style is set toTexturePattern
.The current brush color will only have an effect for monochrome pixmaps, i.e. for
depth()
== 1 (QBitmaps
).See also
- PySide2.QtGui.QBrush.setTextureImage(image)¶
- Parameters:
image –
PySide2.QtGui.QImage
Sets the brush image to
image
. The style is set toTexturePattern
.Note the current brush color will not have any affect on monochrome images, as opposed to calling
setTexture()
with aQBitmap
. If you want to change the color of monochrome image brushes, either convert the image toQBitmap
withQBitmap::fromImage()
and set the resultingQBitmap
as a texture, or change the entries in the color table for the image.See also
- PySide2.QtGui.QBrush.setTransform(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtGui.QTransform
Sets
matrix
as an explicit transformation matrix on the current brush. The brush transformation matrix is merged withQPainter
transformation matrix to produce the final result.See also
- PySide2.QtGui.QBrush.style()¶
- Return type:
Returns the brush style.
See also
- PySide2.QtGui.QBrush.swap(other)¶
- Parameters:
other –
PySide2.QtGui.QBrush
Swaps brush
other
with this brush. This operation is very fast and never fails.
- PySide2.QtGui.QBrush.texture()¶
- Return type:
Returns the custom brush pattern, or a null pixmap if no custom brush pattern has been set.
See also
- PySide2.QtGui.QBrush.textureImage()¶
- Return type:
Returns the custom brush pattern, or a null image if no custom brush pattern has been set.
If the texture was set as a
QPixmap
it will be converted to aQImage
.See also
- PySide2.QtGui.QBrush.transform()¶
- Return type:
Returns the current transformation matrix for the brush.
See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.