QPaintDevice¶
The
QPaintDevice
class is the base class of objects that can be painted on withQPainter
. More…
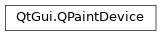
Inherited by: QBitmap, QImage, QOpenGLPaintDevice, QPagedPaintDevice, QPicture, QPixmap, QGLFramebufferObject, QGLPixelBuffer, QPrinter, QSvgGenerator
Synopsis¶
Functions¶
def
colorCount
()def
depth
()def
devicePixelRatio
()def
devicePixelRatioF
()def
height
()def
heightMM
()def
logicalDpiX
()def
logicalDpiY
()def
paintingActive
()def
physicalDpiX
()def
physicalDpiY
()def
width
()def
widthMM
()
Virtual functions¶
def
devType
()def
initPainter
(painter)def
metric
(metric)def
paintEngine
()def
redirected
(offset)def
sharedPainter
()
Static functions¶
def
devicePixelRatioFScale
()
Detailed Description¶
A paint device is an abstraction of a two-dimensional space that can be drawn on using a
QPainter
. Its default coordinate system has its origin located at the top-left position. X increases to the right and Y increases downwards. The unit is one pixel.The drawing capabilities of
QPaintDevice
are currently implemented by theQWidget
,QImage
,QPixmap
,QGLPixelBuffer
,QPicture
, andQPrinter
subclasses.To implement support for a new backend, you must derive from
QPaintDevice
and reimplement the virtualpaintEngine()
function to tellQPainter
which paint engine should be used to draw on this particular device. Note that you also must create a corresponding paint engine to be able to draw on the device, i.e derive fromQPaintEngine
and reimplement its virtual functions.Warning
Qt requires that a
QGuiApplication
object exists before any paint devices can be created. Paint devices access window system resources, and these resources are not initialized before an application object is created.The
QPaintDevice
class provides several functions returning the various device metrics: Thedepth()
function returns its bit depth (number of bit planes). Theheight()
function returns its height in default coordinate system units (e.g. pixels forQPixmap
andQWidget
) whileheightMM()
returns the height of the device in millimeters. Similiarily, thewidth()
andwidthMM()
functions return the width of the device in default coordinate system units and in millimeters, respectively. Alternatively, the protectedmetric()
function can be used to retrieve the metric information by specifying the desiredPaintDeviceMetric
as argument.The
logicalDpiX()
andlogicalDpiY()
functions return the horizontal and vertical resolution of the device in dots per inch. ThephysicalDpiX()
andphysicalDpiY()
functions also return the resolution of the device in dots per inch, but note that if the logical and physical resolution differ, the correspondingQPaintEngine
must handle the mapping. Finally, thecolorCount()
function returns the number of different colors available for the paint device.
- class PySide2.QtGui.QPaintDevice¶
Constructs a paint device. This constructor can be invoked only from subclasses of
QPaintDevice
.
- PySide2.QtGui.QPaintDevice.PaintDeviceMetric¶
Describes the various metrics of a paint device.
Constant
Description
QPaintDevice.PdmWidth
The width of the paint device in default coordinate system units (e.g. pixels for
QPixmap
andQWidget
). See alsowidth()
.QPaintDevice.PdmHeight
The height of the paint device in default coordinate system units (e.g. pixels for
QPixmap
andQWidget
). See alsoheight()
.QPaintDevice.PdmWidthMM
The width of the paint device in millimeters. See also
widthMM()
.QPaintDevice.PdmHeightMM
The height of the paint device in millimeters. See also
heightMM()
.QPaintDevice.PdmNumColors
The number of different colors available for the paint device. See also
colorCount()
.QPaintDevice.PdmDepth
The bit depth (number of bit planes) of the paint device. See also
depth()
.QPaintDevice.PdmDpiX
The horizontal resolution of the device in dots per inch. See also
logicalDpiX()
.QPaintDevice.PdmDpiY
The vertical resolution of the device in dots per inch. See also
logicalDpiY()
.QPaintDevice.PdmPhysicalDpiX
The horizontal resolution of the device in dots per inch. See also
physicalDpiX()
.QPaintDevice.PdmPhysicalDpiY
The vertical resolution of the device in dots per inch. See also
physicalDpiY()
.QPaintDevice.PdmDevicePixelRatio
The device pixel ratio for device. Common values are 1 for normal-dpi displays and 2 for high-dpi “retina” displays.
QPaintDevice.PdmDevicePixelRatioScaled
The scaled device pixel ratio for the device. This is identical to , except that the value is scaled by a constant factor in order to support paint devices with fractional scale factors. The constant scaling factor used is . This enum value has been introduced in Qt 5.6.
See also
- PySide2.QtGui.QPaintDevice.painters¶
- PySide2.QtGui.QPaintDevice.colorCount()¶
- Return type:
int
Returns the number of different colors available for the paint device. If the number of colors available is too great to be represented by the int data type, then INT_MAX will be returned instead.
- PySide2.QtGui.QPaintDevice.depth()¶
- Return type:
int
Returns the bit depth (number of bit planes) of the paint device.
- PySide2.QtGui.QPaintDevice.devType()¶
- Return type:
int
- PySide2.QtGui.QPaintDevice.devicePixelRatio()¶
- Return type:
int
Returns the device pixel ratio for device.
Common values are 1 for normal-dpi displays and 2 for high-dpi “retina” displays.
- PySide2.QtGui.QPaintDevice.devicePixelRatioF()¶
- Return type:
float
Returns the device pixel ratio for the device as a floating point number.
- static PySide2.QtGui.QPaintDevice.devicePixelRatioFScale()¶
- Return type:
float
- PySide2.QtGui.QPaintDevice.height()¶
- Return type:
int
Returns the height of the paint device in default coordinate system units (e.g. pixels for
QPixmap
andQWidget
).See also
- PySide2.QtGui.QPaintDevice.heightMM()¶
- Return type:
int
Returns the height of the paint device in millimeters. Due to platform limitations it may not be possible to use this function to determine the actual physical size of a widget on the screen.
See also
- PySide2.QtGui.QPaintDevice.initPainter(painter)¶
- Parameters:
painter –
PySide2.QtGui.QPainter
- PySide2.QtGui.QPaintDevice.logicalDpiX()¶
- Return type:
int
Returns the horizontal resolution of the device in dots per inch, which is used when computing font sizes. For X11, this is usually the same as could be computed from
widthMM()
.Note that if the doesn’t equal the
physicalDpiX()
, the correspondingQPaintEngine
must handle the resolution mapping.See also
- PySide2.QtGui.QPaintDevice.logicalDpiY()¶
- Return type:
int
Returns the vertical resolution of the device in dots per inch, which is used when computing font sizes. For X11, this is usually the same as could be computed from
heightMM()
.Note that if the doesn’t equal the
physicalDpiY()
, the correspondingQPaintEngine
must handle the resolution mapping.See also
- PySide2.QtGui.QPaintDevice.metric(metric)¶
- Parameters:
metric –
PaintDeviceMetric
- Return type:
int
Returns the metric information for the given paint device
metric
.See also
PaintDeviceMetric
- PySide2.QtGui.QPaintDevice.paintEngine()¶
- Return type:
Returns a pointer to the paint engine used for drawing on the device.
- PySide2.QtGui.QPaintDevice.paintingActive()¶
- Return type:
bool
Returns
true
if the device is currently being painted on, i.e. someone has calledbegin()
but not yet calledend()
for this device; otherwise returnsfalse
.See also
- PySide2.QtGui.QPaintDevice.physicalDpiX()¶
- Return type:
int
Returns the horizontal resolution of the device in dots per inch. For example, when printing, this resolution refers to the physical printer’s resolution. The logical DPI on the other hand, refers to the resolution used by the actual paint engine.
Note that if the doesn’t equal the
logicalDpiX()
, the correspondingQPaintEngine
must handle the resolution mapping.See also
- PySide2.QtGui.QPaintDevice.physicalDpiY()¶
- Return type:
int
Returns the horizontal resolution of the device in dots per inch. For example, when printing, this resolution refers to the physical printer’s resolution. The logical DPI on the other hand, refers to the resolution used by the actual paint engine.
Note that if the doesn’t equal the
logicalDpiY()
, the correspondingQPaintEngine
must handle the resolution mapping.See also
- PySide2.QtGui.QPaintDevice.redirected(offset)¶
- Parameters:
offset –
PySide2.QtCore.QPoint
- Return type:
- Return type:
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.