QPaintEngine¶
The
QPaintEngine
class provides an abstract definition of howQPainter
draws to a given device on a given platform. More…
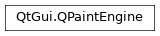
Synopsis¶
Functions¶
def
clearDirty
(df)def
hasFeature
(feature)def
isActive
()def
isExtended
()def
paintDevice
()def
painter
()def
setActive
(newState)def
setDirty
(df)def
setSystemClip
(baseClip)def
setSystemRect
(rect)def
syncState
()def
systemClip
()def
systemRect
()def
testDirty
(df)
Virtual functions¶
def
begin
(pdev)def
coordinateOffset
()def
drawEllipse
(r)def
drawEllipse
(r)def
drawImage
(r, pm, sr[, flags=Qt.AutoColor])def
drawLines
(lines, lineCount)def
drawLines
(lines, lineCount)def
drawPath
(path)def
drawPixmap
(r, pm, sr)def
drawPoints
(points, pointCount)def
drawPoints
(points, pointCount)def
drawPolygon
(points, pointCount, mode)def
drawPolygon
(points, pointCount, mode)def
drawRects
(rects, rectCount)def
drawRects
(rects, rectCount)def
drawTextItem
(p, textItem)def
drawTiledPixmap
(r, pixmap, s)def
end
()def
type
()def
updateState
(state)
Detailed Description¶
Qt provides several premade implementations of
QPaintEngine
for the different painter backends we support. The primary paint engine provided is the raster paint engine, which contains a software rasterizer which supports the full feature set on all supported platforms. This is the default for painting onQWidget
-based classes in e.g. on Windows, X11 and macOS, it is the backend for painting onQImage
and it is used as a fallback for paint engines that do not support a certain capability. In addition we provideQPaintEngine
implementations for OpenGL (accessible throughQOpenGLWidget
) and printing (which allows usingQPainter
to draw on aQPrinter
object).If one wants to use
QPainter
to draw to a different backend, one must subclassQPaintEngine
and reimplement all its virtual functions. TheQPaintEngine
implementation is then made available by subclassingQPaintDevice
and reimplementing the virtual functionpaintEngine()
.
QPaintEngine
is created and owned by theQPaintDevice
that created it.See also
- class PySide2.QtGui.QPaintEngine([features=QPaintEngine.PaintEngineFeatures()])¶
- param features:
PaintEngineFeatures
Creates a paint engine with the featureset specified by
caps
.
- PySide2.QtGui.QPaintEngine.PaintEngineFeature¶
This enum is used to describe the features or capabilities that the paint engine has. If a feature is not supported by the engine,
QPainter
will do a best effort to emulate that feature through other means and pass on an alpha blendedQImage
to the engine with the emulated results. Some features cannot be emulated: and .Constant
Description
QPaintEngine.AlphaBlend
The engine can alpha blend primitives.
QPaintEngine.Antialiasing
The engine can use antialising to improve the appearance of rendered primitives.
QPaintEngine.BlendModes
The engine supports blending modes.
QPaintEngine.BrushStroke
The engine supports drawing strokes that contain brushes as fills, not just solid colors (e.g. a dashed gradient line of width 2).
QPaintEngine.ConicalGradientFill
The engine supports conical gradient fills.
QPaintEngine.ConstantOpacity
The engine supports the feature provided by
setOpacity()
.QPaintEngine.LinearGradientFill
The engine supports linear gradient fills.
QPaintEngine.MaskedBrush
The engine is capable of rendering brushes that has a texture with an alpha channel or a mask.
QPaintEngine.ObjectBoundingModeGradients
The engine has native support for gradients with coordinate mode
ObjectBoundingMode
. Otherwise, if is supported, object bounding mode gradients are converted to gradients with coordinate modeLogicalMode
and a brush transform for the coordinate mapping.QPaintEngine.PainterPaths
The engine has path support.
QPaintEngine.PaintOutsidePaintEvent
The engine is capable of painting outside of paint events.
QPaintEngine.PatternBrush
The engine is capable of rendering brushes with the brush patterns specified in
BrushStyle
.QPaintEngine.PatternTransform
The engine has support for transforming brush patterns.
QPaintEngine.PerspectiveTransform
The engine has support for performing perspective transformations on primitives.
QPaintEngine.PixmapTransform
The engine can transform pixmaps, including rotation and shearing.
QPaintEngine.PorterDuff
The engine supports Porter-Duff operations
QPaintEngine.PrimitiveTransform
The engine has support for transforming drawing primitives.
QPaintEngine.RadialGradientFill
The engine supports radial gradient fills.
QPaintEngine.RasterOpModes
The engine supports bitwise raster operations.
QPaintEngine.AllFeatures
All of the above features. This enum value is usually used as a bit mask.
- PySide2.QtGui.QPaintEngine.DirtyFlag¶
Constant
Description
QPaintEngine.DirtyPen
The pen is dirty and needs to be updated.
QPaintEngine.DirtyBrush
The brush is dirty and needs to be updated.
QPaintEngine.DirtyBrushOrigin
The brush origin is dirty and needs to updated.
QPaintEngine.DirtyFont
The font is dirty and needs to be updated.
QPaintEngine.DirtyBackground
The background is dirty and needs to be updated.
QPaintEngine.DirtyBackgroundMode
The background mode is dirty and needs to be updated.
QPaintEngine.DirtyTransform
The transform is dirty and needs to be updated.
QPaintEngine.DirtyClipRegion
The clip region is dirty and needs to be updated.
QPaintEngine.DirtyClipPath
The clip path is dirty and needs to be updated.
QPaintEngine.DirtyHints
The render hints is dirty and needs to be updated.
QPaintEngine.DirtyCompositionMode
The composition mode is dirty and needs to be updated.
QPaintEngine.DirtyClipEnabled
Whether clipping is enabled or not is dirty and needs to be updated.
QPaintEngine.DirtyOpacity
The constant opacity has changed and needs to be updated as part of the state change in
updateState()
.QPaintEngine.AllDirty
Convenience enum used internally.
These types are used by
QPainter
to trigger lazy updates of the various states in theQPaintEngine
usingupdateState()
.A paint engine must update every dirty state.
- PySide2.QtGui.QPaintEngine.PolygonDrawMode¶
Constant
Description
QPaintEngine.OddEvenMode
The polygon should be drawn using OddEven fill rule.
QPaintEngine.WindingMode
The polygon should be drawn using Winding fill rule.
QPaintEngine.ConvexMode
The polygon is a convex polygon and can be drawn using specialized algorithms where available.
QPaintEngine.PolylineMode
Only the outline of the polygon should be drawn.
- PySide2.QtGui.QPaintEngine.Type¶
Constant
Description
QPaintEngine.X11
QPaintEngine.Windows
QPaintEngine.MacPrinter
QPaintEngine.CoreGraphics
macOS’s Quartz2D ()
QPaintEngine.QuickDraw
macOS’s
QPaintEngine.QWindowSystem
Qt for Embedded Linux
QPaintEngine.PostScript
(No longer supported)
QPaintEngine.OpenGL
QPaintEngine.Picture
QPicture
formatQPaintEngine.SVG
Scalable Vector Graphics XML format
QPaintEngine.Raster
QPaintEngine.Direct3D
Windows only, based engine
QPaintEngine.Pdf
Portable Document Format
QPaintEngine.OpenVG
QPaintEngine.User
First user type ID
QPaintEngine.MaxUser
Last user type ID
QPaintEngine.OpenGL2
QPaintEngine.PaintBuffer
QPaintEngine.Blitter
QPaintEngine.Direct2D
Windows only, based engine
- PySide2.QtGui.QPaintEngine.state¶
- PySide2.QtGui.QPaintEngine.gccaps¶
- PySide2.QtGui.QPaintEngine.active¶
- PySide2.QtGui.QPaintEngine.selfDestruct¶
- PySide2.QtGui.QPaintEngine.extended¶
- PySide2.QtGui.QPaintEngine.begin(pdev)¶
- Parameters:
pdev –
PySide2.QtGui.QPaintDevice
- Return type:
bool
Reimplement this function to initialise your paint engine when painting is to start on the paint device
pdev
. Return true if the initialization was successful; otherwise return false.See also
- PySide2.QtGui.QPaintEngine.clearDirty(df)¶
- Parameters:
df –
DirtyFlags
- PySide2.QtGui.QPaintEngine.coordinateOffset()¶
- Return type:
Returns the offset from the painters origo to the engines origo. This value is used by
QPainter
for engines who have internal double buffering.This function only makes sense when the engine is active.
- PySide2.QtGui.QPaintEngine.drawEllipse(r)¶
- Parameters:
- PySide2.QtGui.QPaintEngine.drawEllipse(r)
- Parameters:
- PySide2.QtGui.QPaintEngine.drawImage(r, pm, sr[, flags=Qt.AutoColor])¶
- Parameters:
pm –
PySide2.QtGui.QImage
flags –
ImageConversionFlags
Reimplement this function to draw the part of the
image
specified by thesr
rectangle in the givenrectangle
using the given conversion flagsflags
, to convert it to a pixmap.
- PySide2.QtGui.QPaintEngine.drawLines(lines, lineCount)¶
- Parameters:
lines –
PySide2.QtCore.QLine
lineCount – int
This is an overloaded function.
The default implementation converts the first
lineCount
lines inlines
to aQLineF
and calls the floating point version of this function.
- PySide2.QtGui.QPaintEngine.drawLines(lines, lineCount)
- Parameters:
lines –
PySide2.QtCore.QLineF
lineCount – int
The default implementation splits the list of lines in
lines
intolineCount
separate calls todrawPath()
ordrawPolygon()
depending on the feature set of the paint engine.
- PySide2.QtGui.QPaintEngine.drawPath(path)¶
- Parameters:
path –
PySide2.QtGui.QPainterPath
The default implementation ignores the
path
and does nothing.
- PySide2.QtGui.QPaintEngine.drawPixmap(r, pm, sr)¶
- Parameters:
Reimplement this function to draw the part of the
pm
specified by thesr
rectangle in the givenr
.
- PySide2.QtGui.QPaintEngine.drawPoints(points, pointCount)¶
- Parameters:
points –
PySide2.QtCore.QPoint
pointCount – int
Draws the first
pointCount
points in the bufferpoints
The default implementation converts the first
pointCount
QPoints inpoints
to QPointFs and calls the floating point version ofdrawPoints
.
- PySide2.QtGui.QPaintEngine.drawPoints(points, pointCount)
- Parameters:
points –
PySide2.QtCore.QPointF
pointCount – int
Draws the first
pointCount
points in the bufferpoints
- PySide2.QtGui.QPaintEngine.drawPolygon(points, pointCount, mode)¶
- Parameters:
points –
PySide2.QtCore.QPoint
pointCount – int
mode –
PolygonDrawMode
This is an overloaded function.
Reimplement this virtual function to draw the polygon defined by the
pointCount
first points inpoints
, using modemode
.Note
At least one of the
drawPolygon()
functions must be reimplemented.
- PySide2.QtGui.QPaintEngine.drawPolygon(points, pointCount, mode)
- Parameters:
points –
PySide2.QtCore.QPointF
pointCount – int
mode –
PolygonDrawMode
Reimplement this virtual function to draw the polygon defined by the
pointCount
first points inpoints
, using modemode
.Note
At least one of the functions must be reimplemented.
- PySide2.QtGui.QPaintEngine.drawRects(rects, rectCount)¶
- Parameters:
rects –
PySide2.QtCore.QRect
rectCount – int
This is an overloaded function.
The default implementation converts the first
rectCount
rectangles in the bufferrects
to aQRectF
and calls the floating point version of this function.
- PySide2.QtGui.QPaintEngine.drawRects(rects, rectCount)
- Parameters:
rects –
PySide2.QtCore.QRectF
rectCount – int
Draws the first
rectCount
rectangles in the bufferrects
. The default implementation of this function callsdrawPath()
ordrawPolygon()
depending on the feature set of the paint engine.
- PySide2.QtGui.QPaintEngine.drawTextItem(p, textItem)¶
- Parameters:
textItem –
PySide2.QtGui.QTextItem
This function draws the text item
textItem
at positionp
. The default implementation of this function converts the text to aQPainterPath
and paints the resulting path.
- PySide2.QtGui.QPaintEngine.drawTiledPixmap(r, pixmap, s)¶
- Parameters:
pixmap –
PySide2.QtGui.QPixmap
Reimplement this function to draw the
pixmap
in the givenrect
, starting at the givenp
. The pixmap will be drawn repeatedly until therect
is filled.
- PySide2.QtGui.QPaintEngine.end()¶
- Return type:
bool
Reimplement this function to finish painting on the current paint device. Return true if painting was finished successfully; otherwise return false.
See also
- PySide2.QtGui.QPaintEngine.hasFeature(feature)¶
- Parameters:
feature –
PaintEngineFeatures
- Return type:
bool
Returns
true
if the paint engine supports the specifiedfeature
; otherwise returnsfalse
.
- PySide2.QtGui.QPaintEngine.isActive()¶
- Return type:
bool
Returns
true
if the paint engine is actively drawing; otherwise returnsfalse
.See also
- PySide2.QtGui.QPaintEngine.isExtended()¶
- Return type:
bool
- PySide2.QtGui.QPaintEngine.paintDevice()¶
- Return type:
Returns the device that this engine is painting on, if painting is active; otherwise returns
None
.
- PySide2.QtGui.QPaintEngine.painter()¶
- Return type:
Returns the paint engine’s painter.
- PySide2.QtGui.QPaintEngine.setActive(newState)¶
- Parameters:
newState – bool
Sets the active state of the paint engine to
state
.See also
- PySide2.QtGui.QPaintEngine.setDirty(df)¶
- Parameters:
df –
DirtyFlags
- PySide2.QtGui.QPaintEngine.setSystemClip(baseClip)¶
- Parameters:
baseClip –
PySide2.QtGui.QRegion
Sets the system clip for this engine. The system clip defines the basis area that the engine has to draw in. All clips that are set will be an intersection with the system clip.
Reset the systemclip to no clip by setting an empty region.
- PySide2.QtGui.QPaintEngine.setSystemRect(rect)¶
- Parameters:
rect –
PySide2.QtCore.QRect
Sets the target rect for drawing within the backing store. This function should ONLY be used by the backing store.
- PySide2.QtGui.QPaintEngine.syncState()¶
- PySide2.QtGui.QPaintEngine.systemClip()¶
- Return type:
Returns the system clip. The system clip is read only while the painter is active. An empty region indicates that system clip is not in use.
- PySide2.QtGui.QPaintEngine.systemRect()¶
- Return type:
Retrieves the rect for drawing within the backing store. This function should ONLY be used by the backing store.
- PySide2.QtGui.QPaintEngine.testDirty(df)¶
- Parameters:
df –
DirtyFlags
- Return type:
bool
- PySide2.QtGui.QPaintEngine.type()¶
- Return type:
Reimplement this function to return the paint engine
Type
.
- PySide2.QtGui.QPaintEngine.updateState(state)¶
- Parameters:
state –
PySide2.QtGui.QPaintEngineState
Reimplement this function to update the state of a paint engine.
When implemented, this function is responsible for checking the paint engine’s current
state
and update the properties that are changed. Use thestate()
function to find out which properties that must be updated, then use the correspondingget function
to retrieve the current values for the given properties.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.