Chapter 6 - Plot the data in the ChartView#
The last step of this tutorial is to plot the CSV data inside our QChart. For this, you need to go over our data and include the data on a QLineSeries.
After adding the data to the series, you can modify the axis to properly display the QDateTime on the X-axis, and the magnitude values on the Y-axis.
Here is the updated main_widget.py
that includes an additional
function to plot data using a QLineSeries:
1
2from PySide6.QtCore import QDateTime, Qt
3from PySide6.QtGui import QPainter
4from PySide6.QtWidgets import (QWidget, QHeaderView, QHBoxLayout, QTableView,
5 QSizePolicy)
6from PySide6.QtCharts import QChart, QChartView, QLineSeries, QDateTimeAxis, QValueAxis
7
8from table_model import CustomTableModel
9
10
11class Widget(QWidget):
12 def __init__(self, data):
13 QWidget.__init__(self)
14
15 # Getting the Model
16 self.model = CustomTableModel(data)
17
18 # Creating a QTableView
19 self.table_view = QTableView()
20 self.table_view.setModel(self.model)
21
22 # QTableView Headers
23 resize = QHeaderView.ResizeToContents
24 self.horizontal_header = self.table_view.horizontalHeader()
25 self.vertical_header = self.table_view.verticalHeader()
26 self.horizontal_header.setSectionResizeMode(resize)
27 self.vertical_header.setSectionResizeMode(resize)
28 self.horizontal_header.setStretchLastSection(True)
29
30 # Creating QChart
31 self.chart = QChart()
32 self.chart.setAnimationOptions(QChart.AllAnimations)
33 self.add_series("Magnitude (Column 1)", [0, 1])
34
35 # Creating QChartView
36 self.chart_view = QChartView(self.chart)
37 self.chart_view.setRenderHint(QPainter.Antialiasing)
38
39 # QWidget Layout
40 self.main_layout = QHBoxLayout()
41 size = QSizePolicy(QSizePolicy.Preferred, QSizePolicy.Preferred)
42
43 # Left layout
44 size.setHorizontalStretch(1)
45 self.table_view.setSizePolicy(size)
46 self.main_layout.addWidget(self.table_view)
47
48 # Right Layout
49 size.setHorizontalStretch(4)
50 self.chart_view.setSizePolicy(size)
51 self.main_layout.addWidget(self.chart_view)
52
53 # Set the layout to the QWidget
54 self.setLayout(self.main_layout)
55
56 def add_series(self, name, columns):
57 # Create QLineSeries
58 self.series = QLineSeries()
59 self.series.setName(name)
60
61 # Filling QLineSeries
62 for i in range(self.model.rowCount()):
63 # Getting the data
64 t = self.model.index(i, 0).data()
65 date_fmt = "yyyy-MM-dd HH:mm:ss.zzz"
66
67 x = QDateTime().fromString(t, date_fmt).toSecsSinceEpoch()
68 y = float(self.model.index(i, 1).data())
69
70 if x > 0 and y > 0:
71 self.series.append(x, y)
72
73 self.chart.addSeries(self.series)
74
75 # Setting X-axis
76 self.axis_x = QDateTimeAxis()
77 self.axis_x.setTickCount(10)
78 self.axis_x.setFormat("dd.MM (h:mm)")
79 self.axis_x.setTitleText("Date")
80 self.chart.addAxis(self.axis_x, Qt.AlignBottom)
81 self.series.attachAxis(self.axis_x)
82 # Setting Y-axis
83 self.axis_y = QValueAxis()
84 self.axis_y.setTickCount(10)
85 self.axis_y.setLabelFormat("%.2f")
86 self.axis_y.setTitleText("Magnitude")
87 self.chart.addAxis(self.axis_y, Qt.AlignLeft)
88 self.series.attachAxis(self.axis_y)
89
90 # Getting the color from the QChart to use it on the QTableView
91 color_name = self.series.pen().color().name()
92 self.model.color = f"{color_name}"
93
Now, run the application to visualize the earthquake magnitudes data at different times.
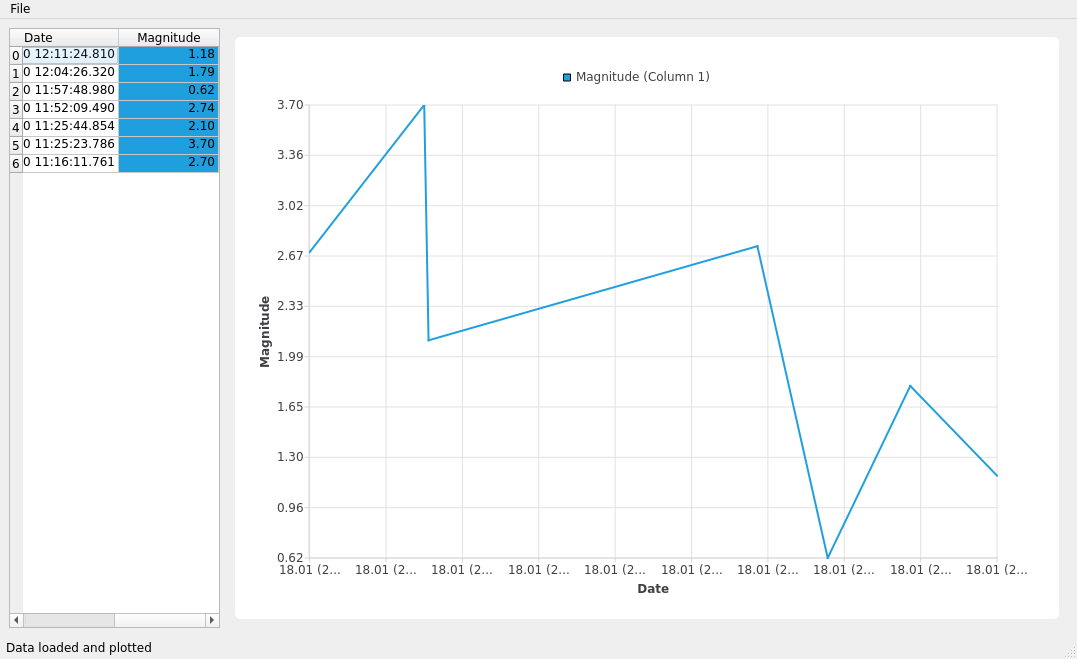
Try modifying the sources to get different output. For example, you could try to plot more data from the CSV.