HelloGraphs Example¶
The example shows how to make a simple 2D bar graph and line graph.
BarGraph¶
The first graph in the example is a bar graph. Creating it starts with a GraphsView component and setting the theme to one which is suitable on dark backgrounds. This theme adjusts the graph background grid and axis lines and labels.
To make this a bar graph, add a BarSeries.
The X axis of the series is a
BarCategoryAxis
with 3 categories. We hide both the vertical grid and the
axis lines. The Y axis of the series is ValueAxis
with visible range
between 20 and 100. Major ticks with labels will be shown on every 10 values
using the tickInterval
property. Minor ticks will be shown on every 1
values setting the minorTickCount
propertyt to 9, which means that between
every major ticks there will be 9 minor ones.
Then data is added into BarSeries
using BarSet.
There are 3 bars, and we define
custom bars color and border properties. These properties will override the possible
theme set for the AbstractSeries.
LineGraph¶
The second graph of the example is a line graph. It also starts by defining a
GraphsView
element. A custom GraphTheme
is created to get a custom appearance.
GraphTheme
offers quite a wide range of customization possibilities for the background
grid and axis, which get applied after the colorTheme
.
A custom Marker
component is used to visualize the data points.
The previous bar graph didn’t define a separate SeriesTheme
, so it uses the
default theme. This line graph uses a custom theme with the desired line colors.
To make this a line graph, add a LineSeries.
The first series defines
axisX
and axisY
for this graph. It also sets the pointMarker
to use
the custom Marker
component that was created earlier. Data points are added
using XYPoint
elements.
The second line series is similar to the first. The axisX
and axisY
don’t need to be defined as the graph already contains them. As this is the
second LineSeries
inside the GraphsView
, second color from the
seriesTheme
gets automatically picked.
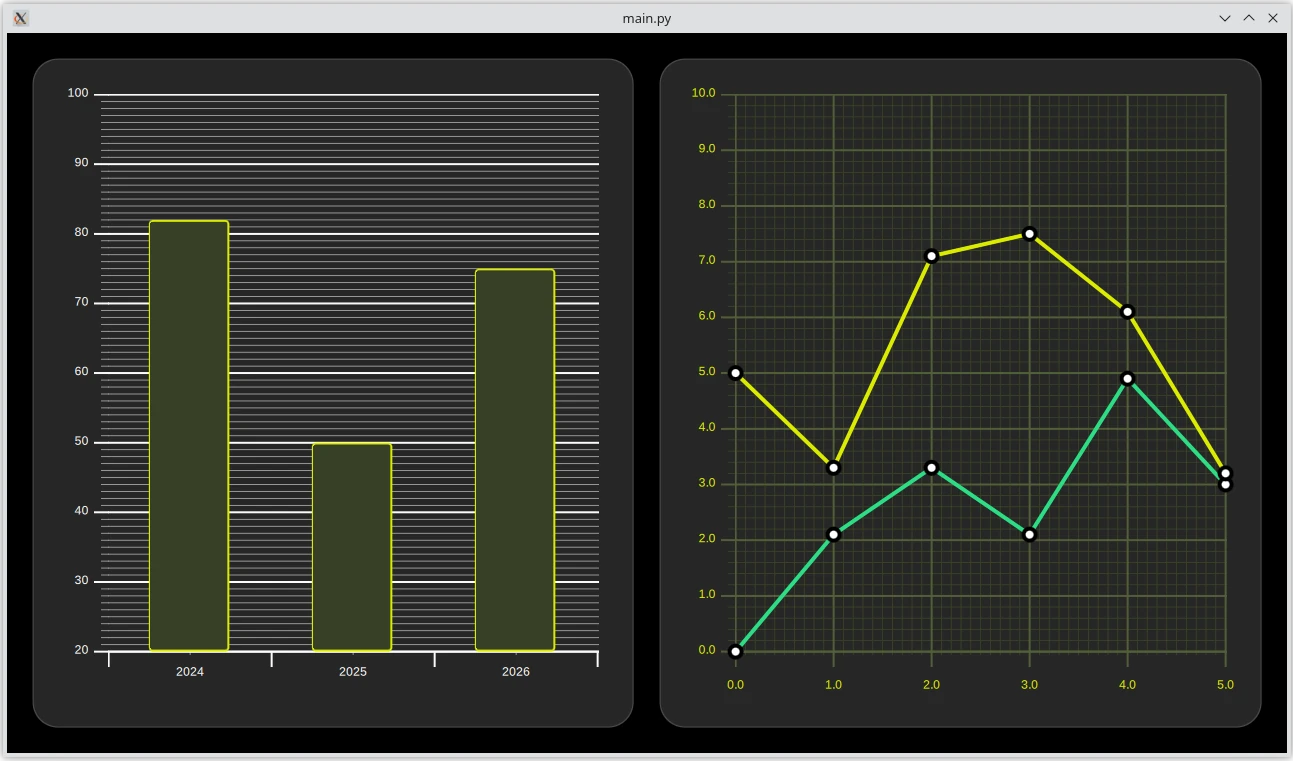
# Copyright (C) 2024 The Qt Company Ltd.
# SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
from __future__ import annotations
"""PySide6 port of the Qt Hello Graphs example from Qt v6.x"""
from pathlib import Path
import sys
from PySide6.QtGui import QGuiApplication
from PySide6.QtQuick import QQuickView
if __name__ == '__main__':
app = QGuiApplication(sys.argv)
viewer = QQuickView()
viewer.engine().addImportPath(Path(__file__).parent)
viewer.setColor("black")
viewer.loadFromModule("HelloGraphs", "Main")
viewer.show()
r = app.exec()
del viewer
sys.exit(r)
// Copyright (C) 2024 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import QtQuick.Layouts
import QtGraphs
Item {
id: mainView
width: 1280
height: 720
RowLayout {
id: graphsRow
readonly property real margin: mainView.width * 0.02
anchors.fill: parent
anchors.margins: margin
spacing: margin
Rectangle {
Layout.fillHeight: true
Layout.fillWidth: true
color: "#262626"
border.color: "#4d4d4d"
border.width: 1
radius: graphsRow.margin
//! [bargraph]
GraphsView {
anchors.fill: parent
anchors.margins: 16
axisX: BarCategoryAxis {
categories: [2024, 2025, 2026]
gridVisible: false
subGridVisible: false
}
axisY: ValueAxis {
min: 20
max: 100
tickInterval: 10
subTickCount: 9
}
theme: GraphsTheme {
colorScheme: Qt.Dark
theme: GraphsTheme.Theme.QtGreen
}
//! [bargraph]
//! [barseries]
BarSeries {
//! [barseries]
//! [barset]
BarSet {
values: [82, 50, 75]
borderWidth: 2
color: "#373F26"
borderColor: "#DBEB00"
}
//! [barset]
}
}
}
Rectangle {
Layout.fillHeight: true
Layout.fillWidth: true
color: "#262626"
border.color: "#4d4d4d"
border.width: 1
radius: graphsRow.margin
//! [linegraph]
GraphsView {
anchors.fill: parent
anchors.margins: 16
theme: GraphsTheme {
readonly property color c1: "#DBEB00"
readonly property color c2: "#373F26"
readonly property color c3: Qt.lighter(c2, 1.5)
colorScheme: Qt.Dark
seriesColors: ["#2CDE85", "#DBEB00"]
grid.mainColor: c3
grid.subColor: c2
axisX.mainColor: c3
axisY.mainColor: c3
axisX.subColor: c2
axisY.subColor: c2
axisX.labelTextColor: c1
axisY.labelTextColor: c1
}
axisX: ValueAxis {
max: 5
tickInterval: 1
subTickCount: 9
labelDecimals: 1
}
axisY: ValueAxis {
max: 10
tickInterval: 1
subTickCount: 4
labelDecimals: 1
}
//! [linegraph]
//! [linemarker]
component Marker : Rectangle {
width: 16
height: 16
color: "#ffffff"
radius: width * 0.5
border.width: 4
border.color: "#000000"
}
//! [linemarker]
//! [lineseries1]
LineSeries {
id: lineSeries1
width: 4
pointDelegate: Marker { }
XYPoint { x: 0; y: 0 }
XYPoint { x: 1; y: 2.1 }
XYPoint { x: 2; y: 3.3 }
XYPoint { x: 3; y: 2.1 }
XYPoint { x: 4; y: 4.9 }
XYPoint { x: 5; y: 3.0 }
}
//! [lineseries1]
//! [lineseries2]
LineSeries {
id: lineSeries2
width: 4
pointDelegate: Marker { }
XYPoint { x: 0; y: 5.0 }
XYPoint { x: 1; y: 3.3 }
XYPoint { x: 2; y: 7.1 }
XYPoint { x: 3; y: 7.5 }
XYPoint { x: 4; y: 6.1 }
XYPoint { x: 5; y: 3.2 }
}
//! [lineseries2]
}
}
}
}
module HelloGraphs
Main 1.0 Main.qml