Qt Quick Examples - Window and Screen¶
This example demonstrates the Window and Screen types in QML.
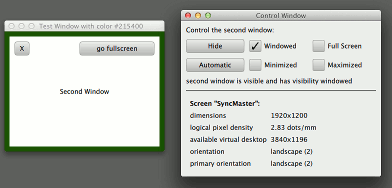
In addition, this example demonstrates the usage of the Qt Resource System in Qt for Python for more advanced scenarios. There are several QML files, one of which imports a module from this sibling directory. Both this “shared” module and the QML files of the example need to be compiled into Python modules with the resource compiler rcc.
For the “shared” module approach to work with QML and rcc, you need:
A module definition qmldir file
A Qt Resource Collection file (.qrc) specifying all the QML files and other resources, plus the qmldir file
The .qrc file is the input to rcc. This will generate a Python module (called shared_rc here) that can then be imported from the Python code. At runtime, only this Python module is needed, not the .qrc file or any of the .qml files or even the image resources, as they have all been compiled into the Python module.
For the example, rcc needs:
A Qt Resource Collection file (.qrc) specifying all the QML files and other resources. There is no qmldir file here because this is not a module.
This will generate a Python module (called window_rc here) that can then be imported from the Python code. Again, only the Python module is needed at runtime.
# Copyright (C) 2022 The Qt Company Ltd.
# SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
from __future__ import annotations
import os
from pathlib import Path
import sys
from PySide6.QtCore import QUrl, qWarning
from PySide6.QtGui import QGuiApplication
from PySide6.QtQml import QQmlComponent, QQmlEngine
from PySide6.QtQuick import QQuickWindow
from PySide6.QtQuickControls2 import QQuickStyle
import rc_window # noqa: F401
# Append the parent directory of this file so that Python can find and
# import from the "shared" sibling directory.
sys.path.append(os.fspath(Path(__file__).parent.parent))
from shared import shared_rc # noqa: F401, E402
if __name__ == "__main__":
app = QGuiApplication(sys.argv)
if sys.platform == "win32":
QQuickStyle.setStyle("Fusion")
engine = QQmlEngine()
# Add the qrc root as QML import path so that the "shared" module
# can be found.
engine.addImportPath(":/")
component = QQmlComponent(engine)
QQuickWindow.setDefaultAlphaBuffer(True)
component.loadUrl(QUrl("qrc:///window/window.qml"))
if component.isReady():
component.create()
else:
qWarning(component.errorString())
app.exit(1)
app.exec()
// Copyright (C) 2021 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import QtQuick.Controls
QtObject {
id: root
property real defaultSpacing: 10
property SystemPalette palette: SystemPalette { }
property var controlWindow: Window {
width: col.implicitWidth + root.defaultSpacing * 2
height: col.implicitHeight + root.defaultSpacing * 2
color: root.palette.window
title: "Control Window"
Column {
id: col
anchors.fill: parent
anchors.margins: root.defaultSpacing
spacing: root.defaultSpacing
property real cellWidth: col.width / 3 - spacing
Label { text: "Control the second window:" }
Grid {
id: grid
columns: 3
spacing: root.defaultSpacing
width: parent.width
Button {
id: showButton
width: col.cellWidth
text: root.testWindow.visible ? "Hide" : "Show"
onClicked: root.testWindow.visible = !root.testWindow.visible
}
//! [windowedCheckbox]
CheckBox {
text: "Windowed"
height: showButton.height
width: col.cellWidth
Binding on checked { value: root.testWindow.visibility === Window.Windowed }
onClicked: root.testWindow.visibility = Window.Windowed
}
//! [windowedCheckbox]
CheckBox {
height: showButton.height
width: col.cellWidth
text: "Full Screen"
Binding on checked { value: root.testWindow.visibility === Window.FullScreen }
onClicked: root.testWindow.visibility = Window.FullScreen
}
Button {
id: autoButton
width: col.cellWidth
text: "Automatic"
onClicked: root.testWindow.visibility = Window.AutomaticVisibility
}
CheckBox {
height: autoButton.height
text: "Minimized"
Binding on checked { value: root.testWindow.visibility === Window.Minimized }
onClicked: root.testWindow.visibility = Window.Minimized
}
CheckBox {
height: autoButton.height
text: "Maximized"
Binding on checked { value: root.testWindow.visibility === Window.Maximized }
onClicked: root.testWindow.visibility = Window.Maximized
}
}
function visibilityToString(v) {
switch (v) {
case Window.Windowed:
return "windowed";
case Window.Minimized:
return "minimized";
case Window.Maximized:
return "maximized";
case Window.FullScreen:
return "fullscreen";
case Window.AutomaticVisibility:
return "automatic";
case Window.Hidden:
return "hidden";
}
return "unknown";
}
Label {
id: visibilityLabel
text: "second window is " + (root.testWindow.visible ? "visible" : "invisible") +
" and has visibility " + parent.visibilityToString(root.testWindow.visibility)
}
Rectangle {
color: root.palette.text
width: parent.width
height: 1
}
CurrentScreen { }
Rectangle {
color: root.palette.text
width: parent.width
height: 1
}
AllScreens { width: parent.width }
}
}
property var testWindow: Window {
width: 320
height: 240
color: "#215400"
title: "Test Window with color " + color
flags: Qt.Window | Qt.WindowFullscreenButtonHint
Rectangle {
anchors.fill: parent
anchors.margins: root.defaultSpacing
Label {
anchors.centerIn: parent
text: "Second Window"
}
MouseArea {
anchors.fill: parent
onClicked: root.testWindow.color = "#e0c31e"
}
Button {
anchors.right: parent.right
anchors.top: parent.top
anchors.margins: root.defaultSpacing
text: root.testWindow.visibility === Window.FullScreen ? "exit fullscreen" : "go fullscreen"
width: 150
onClicked: {
if (root.testWindow.visibility === Window.FullScreen)
root.testWindow.visibility = Window.AutomaticVisibility
else
root.testWindow.visibility = Window.FullScreen
}
}
Button {
anchors.left: parent.left
anchors.top: parent.top
anchors.margins: root.defaultSpacing
text: "X"
width: 30
onClicked: root.testWindow.close()
}
}
}
property var splashWindow: Splash {
onTimeout: root.controlWindow.visible = true
}
}
<RCC>
<qresource prefix="/window">
<file>window.qml</file>
<file>Splash.qml</file>
<file>CurrentScreen.qml</file>
<file>AllScreens.qml</file>
</qresource>
</RCC>
// Copyright (C) 2021 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import shared
//! [splash-properties]
Window {
id: splash
color: "transparent"
title: "Splash Window"
modality: Qt.ApplicationModal
flags: Qt.SplashScreen
property int timeoutInterval: 2000
signal timeout
//! [splash-properties]
//! [screen-properties]
x: (Screen.width - splashImage.width) / 2
y: (Screen.height - splashImage.height) / 2
//! [screen-properties]
width: splashImage.width
height: splashImage.height
Image {
id: splashImage
source: Images.qtLogo
MouseArea {
anchors.fill: parent
onClicked: Qt.quit()
}
}
//! [timer]
Timer {
interval: splash.timeoutInterval; running: true; repeat: false
onTriggered: {
splash.visible = false
splash.timeout()
}
}
//! [timer]
Component.onCompleted: visible = true
}
// Copyright (C) 2021 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import QtQuick.Controls
Column {
id: root
spacing: 8
Label {
text: "Total number of screens: " + screenInfo.count
font.bold: true
}
Flow {
spacing: 12
width: parent.width
Repeater {
id: screenInfo
model: (Qt.application as Application).screens
Label {
required property string name
required property int virtualX
required property int virtualY
required property var modelData // avoid shadowing Label.width and height
lineHeight: 1.5
text: name + "\n" + virtualX + ", " + virtualY + " " + modelData.width + "x" + modelData.height
}
}
}
Component.onCompleted: {
var screens = (Qt.application as Application).screens;
for (var i = 0; i < screens.length; ++i)
console.log("screen " + screens[i].name + " has geometry " +
screens[i].virtualX + ", " + screens[i].virtualY + " " +
screens[i].width + "x" + screens[i].height)
}
}
// Copyright (C) 2021 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import QtQuick.Controls
Item {
id: root
width: 400
height: propertyGrid.implicitHeight + 16
function orientationToString(o) {
switch (o) {
case Qt.PrimaryOrientation:
return "primary";
case Qt.PortraitOrientation:
return "portrait";
case Qt.LandscapeOrientation:
return "landscape";
case Qt.InvertedPortraitOrientation:
return "inverted portrait";
case Qt.InvertedLandscapeOrientation:
return "inverted landscape";
}
return "unknown";
}
Grid {
id: propertyGrid
columns: 2
spacing: 8
x: spacing
y: spacing
//! [screen]
Label {
text: "Screen \"" + Screen.name + "\":"
font.bold: true
}
Item { width: 1; height: 1 } // spacer
Label { text: "manufacturer" }
Label { text: Screen.manufacturer ? Screen.manufacturer : "unknown" }
Label { text: "model" }
Label { text: Screen.model ? Screen.model : "unknown" }
Label { text: "serial number" }
Label { text: Screen.serialNumber ? Screen.serialNumber : "unknown" }
Label { text: "dimensions" }
Label { text: Screen.width + "x" + Screen.height }
Label { text: "pixel density" }
Label { text: Screen.pixelDensity.toFixed(2) + " dots/mm (" + (Screen.pixelDensity * 25.4).toFixed(2) + " dots/inch)" }
Label { text: "logical pixel density" }
Label { text: Screen.logicalPixelDensity.toFixed(2) + " dots/mm (" + (Screen.logicalPixelDensity * 25.4).toFixed(2) + " dots/inch)" }
Label { text: "device pixel ratio" }
Label { text: Screen.devicePixelRatio.toFixed(2) }
Label { text: "available virtual desktop" }
Label { text: Screen.desktopAvailableWidth + "x" + Screen.desktopAvailableHeight }
Label { text: "position in virtual desktop" }
Label { text: Screen.virtualX + ", " + Screen.virtualY }
Label { text: "orientation" }
Label { text: root.orientationToString(Screen.orientation) + " (" + Screen.orientation + ")" }
Label { text: "primary orientation" }
Label { text: root.orientationToString(Screen.primaryOrientation) + " (" + Screen.primaryOrientation + ")" }
//! [screen]
Label { text: "10mm rectangle" }
Rectangle {
color: "red"
width: Screen.pixelDensity * 10
height: width
}
}
}