Extending QML - Plugins Example#
This is the last of a series of 6 examples forming a tutorial about extending QML with Python.
This example refers to the Python version of using a QML plugin in Python. The
idea of plugins in Python is non-existent because Python modules are
dynamically loaded anyway. We use this idea and our QML type registration
decorators - QmlELement
/QmlNamedElement
- to register the QML modules as they
are imported. The pyside6-qml
tool does this for you by simply pointing to the
.qml
file.
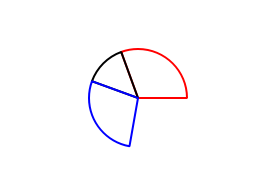
Running the Example#
pyside6-qml examples/qml/tutorials/extending-qml/chapter6-plugins/app.qml -I examples/qml/tutorials/extending-qml/chapter6-plugins/Charts
// Copyright (C) 2022 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import Charts 1.0
Item {
width: 300; height: 200
PieChart {
anchors.centerIn: parent
width: 100; height: 100
slices: [
PieSlice {
anchors.fill: parent
color: "red"
fromAngle: 0; angleSpan: 110
},
PieSlice {
anchors.fill: parent
color: "black"
fromAngle: 110; angleSpan: 50
},
PieSlice {
anchors.fill: parent
color: "blue"
fromAngle: 160; angleSpan: 100
}
]
}
}
# Copyright (C) 2022 The Qt Company Ltd.
# SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
from PySide6.QtCore import Property
from PySide6.QtQml import QmlElement, ListProperty
from PySide6.QtQuick import QQuickItem
from pieslice import PieSlice
# To be used on the @QmlElement decorator
# (QML_IMPORT_MINOR_VERSION is optional)
QML_IMPORT_NAME = "Charts"
QML_IMPORT_MAJOR_VERSION = 1
@QmlElement
class PieChart(QQuickItem):
def __init__(self, parent=None):
super().__init__(parent)
self._slices = []
self._name = ''
@Property(str, final=True)
def name(self):
return self._name
@name.setter
def name(self, name):
self._name = name
def slice(self, n):
return self._slices[n]
def sliceCount(self):
return len(self._slices)
def append_and_setparent(self, slice):
self._slices.append(slice)
slice.setParentItem(self)
slices = ListProperty(PieSlice, append_and_setparent)
# Copyright (C) 2022 The Qt Company Ltd.
# SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
from PySide6.QtCore import Property, QRectF
from PySide6.QtGui import QColor, QPainter, QPen
from PySide6.QtQuick import QQuickPaintedItem
from PySide6.QtQml import QmlElement
# To be used on the @QmlElement decorator
# (QML_IMPORT_MINOR_VERSION is optional)
QML_IMPORT_NAME = "Charts"
QML_IMPORT_MAJOR_VERSION = 1
@QmlElement
class PieSlice(QQuickPaintedItem):
def __init__(self, parent=None):
super().__init__(parent)
self._color = QColor()
self._from_angle = 0
self._angle_span = 0
@Property(QColor, final=True)
def color(self):
return self._color
@color.setter
def color(self, color):
self._color = QColor(color)
@Property(int, final=True)
def fromAngle(self):
return self._from_angle
@fromAngle.setter
def fromAngle(self, fromAngle):
self._from_angle = fromAngle
@Property(int, final=True)
def angleSpan(self):
return self._angle_span
@angleSpan.setter
def angleSpan(self, angleSpan):
self._angle_span = angleSpan
def paint(self, painter):
painter.setPen(QPen(self._color, 2))
painter.setRenderHint(QPainter.RenderHint.Antialiasing, True)
rect = QRectF(0, 0, self.width(), self.height()).adjusted(1, 1, -1, -1)
painter.drawPie(rect, self._from_angle * 16, self._angle_span * 16)